Vector and ArrayList in Java
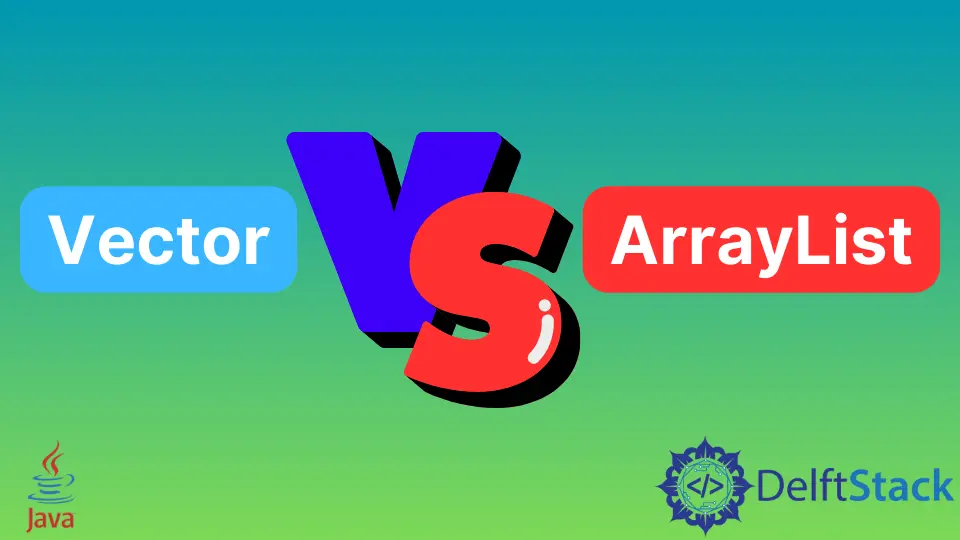
Both ArrayList
and Vector
implement the List Interface, and both can be used as arrays for the internal data structure. But though they are mostly the same, they have their differences.
The synchronization is the most significant difference between the ArrayList
and Vectors. Among them, the Vector
is synchronized, which means only one thread can access it at a time.
On the other hand, the ArrayList
is not synchronized; multiple threads can access it simultaneously.
If we compare the ArrayList
and the Vector
in terms of performance, then the ArrayList
is much faster than the Vector
because only one thread can work on a vector at a time.
Most professional programmers support using the ArrayList
instead of the Vector
because the ArrayList
can be synchronized.
This article will discuss using the ArrayList
and the Vector
. Also, we will see necessary examples and explanations to make the topic easier.
Vector and ArrayList
in Java
In our example below, we illustrated both the ArrayList
and the Vector
. We will declare both the ArrayList
and the Vector
, add some elements, and print the element we added to them.
Have a look at our below example code.
// Importing necessary packages.
import java.io.*;
import java.util.*;
public class JavaArraylist {
public static void main(String[] args) {
// Creating an ArrayList with the type "String"
ArrayList<String> MyArrayList = new ArrayList<String>();
// Adding elements to arraylist
MyArrayList.add("This is the first string element.");
MyArrayList.add("This is the second string element.");
MyArrayList.add("This is the third string element.");
MyArrayList.add("This is the fourth string element.");
// Traversing the elements using Iterator from the MyArrayList
System.out.println("The arrayList elements are:");
Iterator Itr = MyArrayList.iterator();
while (Itr.hasNext()) System.out.println(Itr.next());
// Creating a Vector with the type "String"
Vector<String> MyVectorList = new Vector<String>();
MyVectorList.addElement("Designing");
MyVectorList.addElement("Planning");
MyVectorList.addElement("Coding");
// Traversing the elements using Enumeration from the MyVectorList
System.out.println("\nThe Vector elements are:");
Enumeration enm = MyVectorList.elements();
while (enm.hasMoreElements()) System.out.println(enm.nextElement());
}
}
Output:
The arrayList elements are:
This is the first string element.
This is the second string element.
This is the third string element.
This is the fourth string element.
The Vector elements are:
Designing
Planning
coding
Let’s explain the code. We already commented on the purpose of each line.
In our example above, we first created an ArrayList
named MyArrayList
and then added some string elements. After that, we printed all the elements of the MyArrayList
using the Iterator
.
Now we created a VectorList
named MyVectorList
. Then we added some string elements to it and printed the elements of the MyVectorList
using the Enumeration
.
After running the above example, you will get an output like the one below.
The arrayList elements are:
This is the first string element.
This is the second string element.
This is the third string element.
This is the fourth string element.
The Vector elements are:
Designing
Planning
coding
If you are working on a multi-threaded project, then the ArrayList
will be your best option.
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn