How to Find Unique Values in Java ArrayList
-
Use the
distinct()
Method in theArrayList
to Find Unique Values in Java -
Use the
HashSet
to Find Unique Values in Java
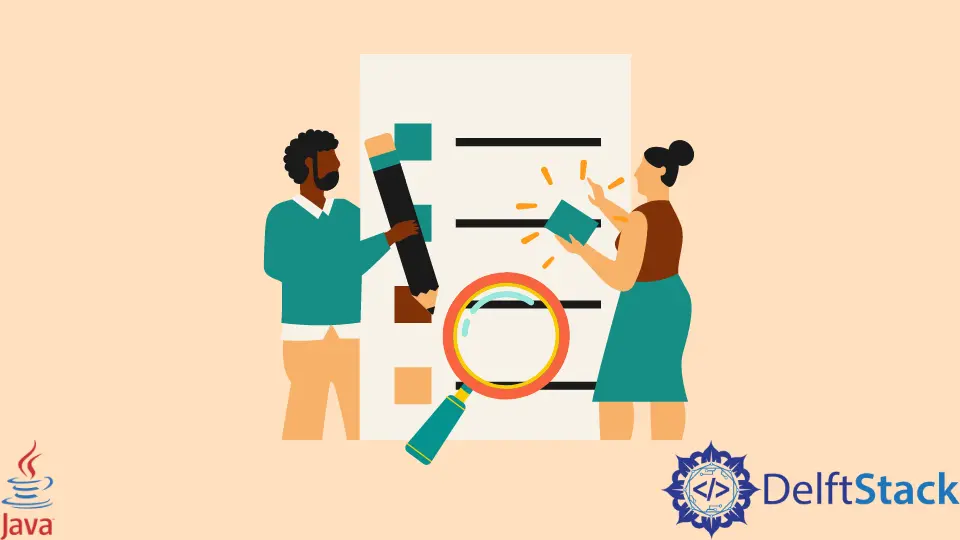
In Java, the ArrayList
can’t prevent the list that contains any duplicate values. But sometimes, we need to extract only the unique values for numerous purposes.
This article will demonstrate how we can only extract the unique values from the Java list. We will discuss the topic by following examples and explanations to make the topic easier.
In our discussion, we will introduce two methods to extract unique values from the list. First, we will see the ArrayList
, and secondly, we will see the HashMap
for extracting the unique values from the list.
Use the distinct()
Method in the ArrayList
to Find Unique Values in Java
In the below example, we will illustrate how we can find the unique values from the list using the method distinct()
. The example code is shown below:
// importing necessary packages
import java.util.*;
import java.util.stream.Collectors;
public class UniqueList {
public static void main(String[] args) {
// Creating an integer ArrayList
ArrayList<Integer> NumList = new ArrayList<Integer>();
// Adding elements to the ArrayList
NumList.add(10);
NumList.add(20);
NumList.add(10);
NumList.add(40);
NumList.add(20);
// Collecting the unique values
List<Integer> UniqueList = NumList.stream().distinct().collect(Collectors.toList());
System.out.println("The unique values are:");
// Printing the unique values
for (int i = 0; i < UniqueList.size(); ++i) {
System.out.println(UniqueList.get(i));
}
}
}
We have commanded the purpose of each line. When you execute the above example code, you will get an output like the one below on your console.
The unique values are:
20
40
10
Use the HashSet
to Find Unique Values in Java
Our example below will demonstrate how we can find the unique values from the list using the HashSet
. Have a look at the following example code.
// importing necessary packages
import java.util.ArrayList;
import java.util.HashSet;
public class UniqueList {
public static void main(String[] args) {
// Creating an integer ArrayList
ArrayList<Integer> NumList = new ArrayList<Integer>();
// Adding elements to the ArrayList
NumList.add(10);
NumList.add(20);
NumList.add(10);
NumList.add(40);
NumList.add(20);
// Creating a HashSet using the ArrayList
HashSet<Integer> UniqueList = new HashSet<Integer>(NumList);
System.out.println("The unique values are:");
// Printing the unique values
for (Integer StrNum : UniqueList) System.out.println(StrNum);
}
}
We have commanded the purpose of each line. When you execute the above example code, you will get an output like the one below on your console.
The unique values are:
20
40
10
Note that the example codes shared here are in Java. You must install Java on your environment if your system doesn’t have it.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn