Java의 벡터 및 ArrayList
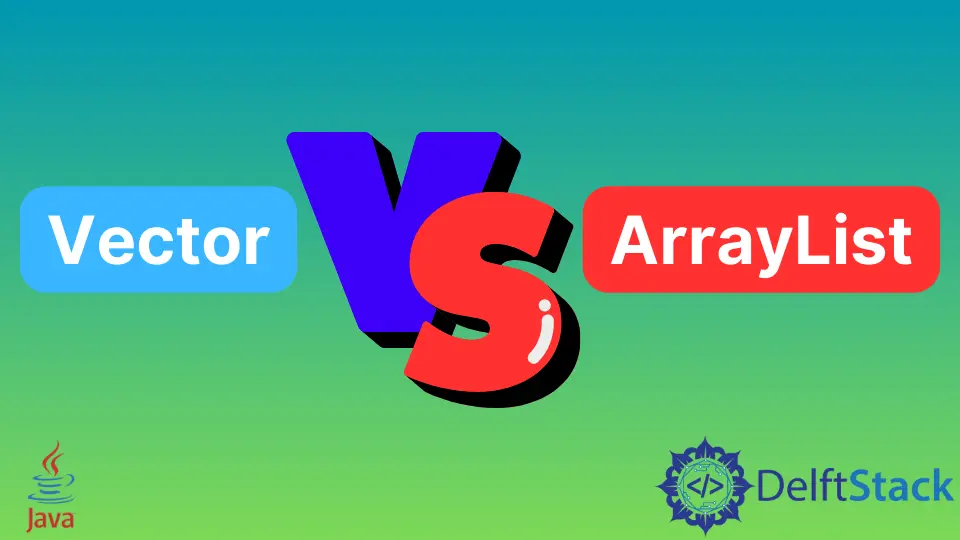
ArrayList
와 Vector
모두 목록 인터페이스를 구현하며 둘 다 내부 데이터 구조의 배열로 사용할 수 있습니다. 그러나 그들은 대부분 동일하지만 차이점이 있습니다.
동기화는 ArrayList
와 벡터 간의 가장 중요한 차이점입니다. 그중 Vector
는 동기화되어 있어 한 번에 하나의 스레드만 액세스할 수 있습니다.
반면 ArrayList
는 동기화되지 않습니다. 여러 스레드가 동시에 액세스할 수 있습니다.
성능 측면에서 ArrayList
와 Vector
를 비교하면 ArrayList
가 Vector
보다 훨씬 빠릅니다. 왜냐하면 한 번에 하나의 벡터에서만 스레드가 작업할 수 있기 때문입니다.
대부분의 전문 프로그래머는 ArrayList
를 동기화할 수 있기 때문에 Vector
대신 ArrayList
사용을 지원합니다.
이 기사에서는 ArrayList
및 Vector
사용에 대해 설명합니다. 또한 주제를 더 쉽게 만들기 위해 필요한 예와 설명을 볼 것입니다.
Java의 벡터 및 ArrayList
아래 예에서는 ArrayList
와 Vector
를 모두 설명했습니다. ArrayList
와 Vector
를 모두 선언하고 일부 요소를 추가한 다음 여기에 추가한 요소를 인쇄합니다.
아래 예제 코드를 살펴보십시오.
// Importing necessary packages.
import java.io.*;
import java.util.*;
public class JavaArraylist {
public static void main(String[] args) {
// Creating an ArrayList with the type "String"
ArrayList<String> MyArrayList = new ArrayList<String>();
// Adding elements to arraylist
MyArrayList.add("This is the first string element.");
MyArrayList.add("This is the second string element.");
MyArrayList.add("This is the third string element.");
MyArrayList.add("This is the fourth string element.");
// Traversing the elements using Iterator from the MyArrayList
System.out.println("The arrayList elements are:");
Iterator Itr = MyArrayList.iterator();
while (Itr.hasNext()) System.out.println(Itr.next());
// Creating a Vector with the type "String"
Vector<String> MyVectorList = new Vector<String>();
MyVectorList.addElement("Designing");
MyVectorList.addElement("Planning");
MyVectorList.addElement("Coding");
// Traversing the elements using Enumeration from the MyVectorList
System.out.println("\nThe Vector elements are:");
Enumeration enm = MyVectorList.elements();
while (enm.hasMoreElements()) System.out.println(enm.nextElement());
}
}
코드를 설명하겠습니다. 우리는 이미 각 행의 목적에 대해 설명했습니다.
위의 예에서는 먼저 MyArrayList
라는 ArrayList
를 만든 다음 일부 문자열 요소를 추가했습니다. 그런 다음 Iterator
를 사용하여 MyArrayList
의 모든 요소를 인쇄했습니다.
이제 MyVectorList
라는 VectorList
를 만들었습니다. 그런 다음 문자열 요소를 추가하고 Enumeration
을 사용하여 MyVectorList
의 요소를 인쇄했습니다.
위의 예제를 실행하면 아래와 같은 결과를 얻을 수 있습니다.
The arrayList elements are:
This is the first string element.
This is the second string element.
This is the third string element.
This is the fourth string element.
The Vector elements are:
Designing
Planning
coding
다중 스레드 프로젝트에서 작업하는 경우 ArrayList
가 최선의 선택이 될 것입니다.
여기에서 공유되는 코드 예제는 Java로 되어 있으며 시스템에 Java가 포함되어 있지 않은 경우 환경에 Java를 설치해야 합니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn