Java에서 날짜별로 ArrayList의 객체를 정렬하는 방법
Asad Riaz
2023년10월12일
Java
Java ArrayList
Java Sorting
-
Java의 날짜별로
ArrayList
의 객체를 정렬하는comparable<>
메소드 -
Java의 날짜순으로
ArrayList
의 객체를 정렬하는collections.sort()
메소드 -
Java에서 날짜별로
ArrayList
에서 객체를 정렬하는list.sort()
메소드
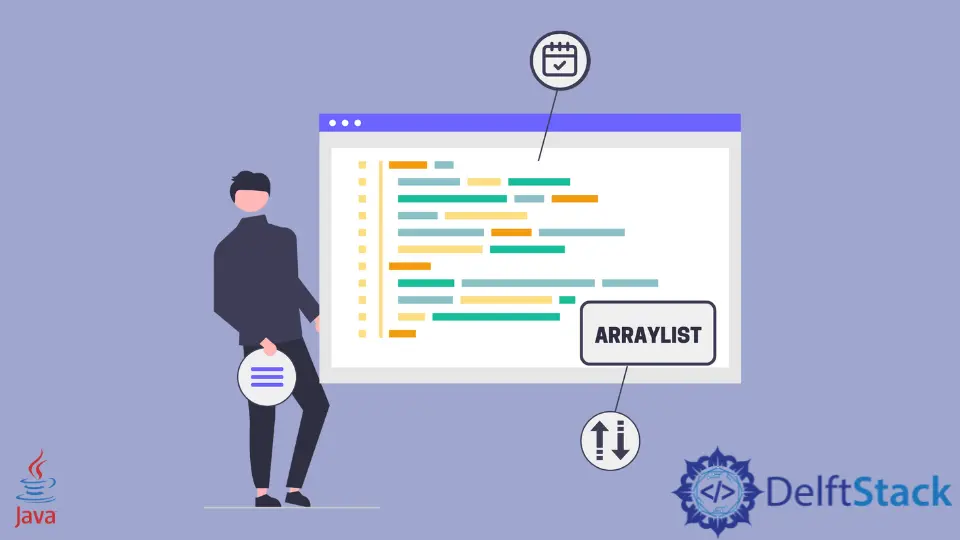
ArrayList
의 객체를 날짜별로 Java로 정렬하는 여러 가지 방법이 있습니다. 이러한 정렬은 날짜 또는 기타 조건에 따라 수행 될 수 있습니다. 예를 들어,comparable<>
,Collections.sort()
및list.sort()
메소드를 작성하십시오.
Java의 날짜별로ArrayList
의 객체를 정렬하는comparable<>
메소드
첫 번째 방법은compareTo()
,compare()
및collections.sort()
클래스를 사용하여comparable<>
객체를 만들어 작동합니다. DateItem
객체에 대한 새 클래스를 만들고Comparator<DateItem>
인터페이스를 구현하여 배열을 정렬합니다.
예제 코드:
import java.util.*;
public class SimpleTesing {
static class DateItem {
String datetime;
DateItem(String date) {
this.datetime = date;
}
}
static class SortByDate implements Comparator<DateItem> {
@Override
public int compare(DateItem a, DateItem b) {
return a.datetime.compareTo(b.datetime);
}
}
public static void main(String args[]) {
List<DateItem> dateList = new ArrayList<>();
dateList.add(new DateItem("2020-03-25"));
dateList.add(new DateItem("2019-01-27"));
dateList.add(new DateItem("2020-03-26"));
dateList.add(new DateItem("2020-02-26"));
Collections.sort(dateList, new SortByDate());
dateList.forEach(date -> { System.out.println(date.datetime); });
}
}
출력:
2019-01-27
2020-02-26
2020-03-25
2020-03-26
Java의 날짜순으로 ArrayList
의 객체를 정렬하는collections.sort()
메소드
collections.sort()
메소드는ArrayList
에서 날짜별로 정렬을 수행 할 수 있습니다.
예제 코드:
import java.util.*;
public class SimpleTesting {
public static void main(String[] args) {
List<String> dateArray = new ArrayList<String>();
dateArray.add("2020-03-25");
dateArray.add("2019-01-27");
dateArray.add("2020-03-26");
dateArray.add("2020-02-26");
System.out.println("The Object before sorting is : " + dateArray);
Collections.sort(dateArray);
System.out.println("The Object after sorting is : " + dateArray);
}
}
출력:
The Object before sorting is : [2020-03-25, 2019-01-27, 2020-03-26, 2020-02-26]
The Object after sorting is : [2019-01-27, 2020-02-26, 2020-03-25, 2020-03-26]
Java에서 날짜별로ArrayList
에서 객체를 정렬하는list.sort()
메소드
Java의list.sort()
메소드는 람다 식과 결합하여ArrayList
에서date
를 기준으로 정렬을 수행합니다.
예제 코드:
import java.util.*;
public class SimpleTesting {
public static void main(String[] args) {
List<String> dateArray = new ArrayList<String>();
dateArray.add("2020-03-25");
dateArray.add("2019-01-27");
dateArray.add("2020-03-26");
dateArray.add("2020-02-26");
System.out.println("The Object before sorting is : " + dateArray);
dateArray.sort((d1, d2) -> d1.compareTo(d2));
System.out.println("The Object after sorting is : " + dateArray);
}
}
출력:
The Object before sorting is : [2020-03-25, 2019-01-27, 2020-03-26, 2020-02-26]
The Object after sorting is : [2019-01-27, 2020-02-26, 2020-03-25, 2020-03-26]
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다