How to Get First Element From List in Java
- Understanding Java Lists
- Using ArrayList to Get the First Element
- Using LinkedList to Get the First Element
- Conclusion
- FAQ
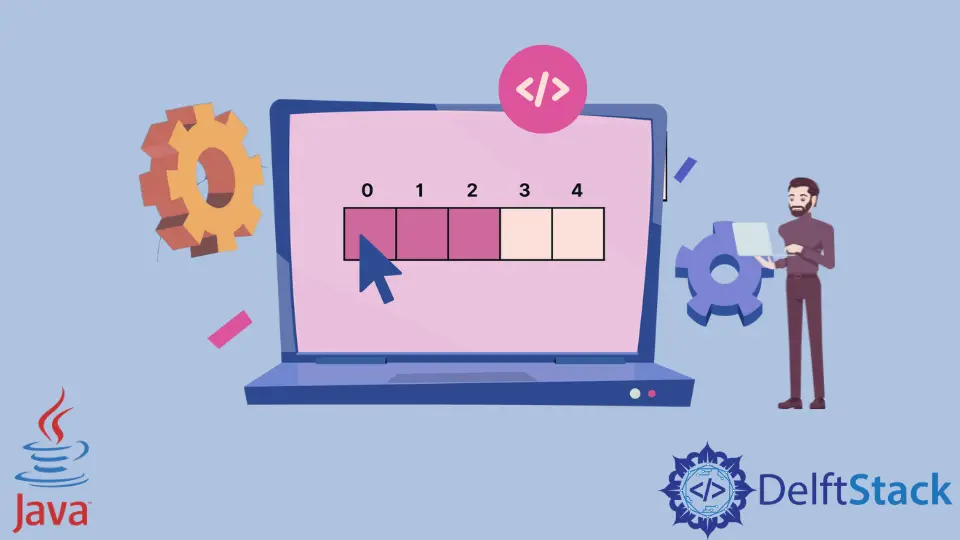
In Java, lists are widely used to store collections of data. Whether you’re working with an ArrayList, LinkedList, or any other type of list, knowing how to retrieve the first element is essential for effective data manipulation.
This tutorial will discuss how to get the first element from a list in Java, providing clear explanations and examples to help you master this fundamental skill. By the end of this article, you’ll be equipped with the knowledge to confidently extract the first item from any list, enhancing your programming capabilities and efficiency.
Understanding Java Lists
Java provides several built-in classes for managing lists, with the most common being ArrayList
and LinkedList
. Both of these classes implement the List
interface, allowing you to store elements in a dynamic array or a linked structure, respectively. Lists in Java are zero-indexed, meaning the first element is always at index 0. This feature makes it straightforward to access the first element, but the method of doing so can vary slightly depending on the type of list you are using.
Using ArrayList to Get the First Element
The ArrayList
class is one of the most commonly used list implementations in Java. It allows you to store elements dynamically and provides various methods to manipulate the list. To retrieve the first element from an ArrayList
, you can use the get()
method, passing in the index of the desired element. Here’s how you can do it:
import java.util.ArrayList;
public class FirstElementExample {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.add("C++");
String firstElement = list.get(0);
System.out.println("The first element is: " + firstElement);
}
}
Output:
The first element is: Java
In this example, we first create an ArrayList
of strings and add three programming languages to it. By calling list.get(0)
, we retrieve the first element, which is “Java”. The get()
method is efficient and provides direct access to the element at the specified index. This method is particularly useful when you need to access elements frequently or perform operations based on the first item in the list.
Using LinkedList to Get the First Element
The LinkedList
class is another popular list implementation in Java, especially when you need to perform frequent insertions and deletions. Like ArrayList
, you can also retrieve the first element using the get()
method. However, LinkedList
also provides a more efficient method called getFirst()
, which is specifically designed for this purpose. Here’s an example:
import java.util.LinkedList;
public class FirstElementLinkedListExample {
public static void main(String[] args) {
LinkedList<String> linkedList = new LinkedList<>();
linkedList.add("JavaScript");
linkedList.add("Ruby");
linkedList.add("Go");
String firstElement = linkedList.getFirst();
System.out.println("The first element is: " + firstElement);
}
}
Output:
The first element is: JavaScript
In this example, we create a LinkedList
and add three programming languages. Using linkedList.getFirst()
, we retrieve the first element, which is “JavaScript”. This method is particularly advantageous because it avoids the overhead of indexing, making it slightly faster than using the get()
method for the first element. If you are working with linked lists and need to frequently access the first element, using getFirst()
is a best practice.
Conclusion
Retrieving the first element from a list in Java is a straightforward process, whether you are using an ArrayList
or a LinkedList
. By understanding the differences between these two implementations and the methods available to access the first element, you can choose the right approach for your specific needs. With this knowledge, you can enhance your Java programming skills and effectively manipulate lists in your applications.
FAQ
-
How do I check if a list is empty before getting the first element?
You can use theisEmpty()
method to check if a list is empty. If it returnstrue
, you should avoid callingget(0)
orgetFirst()
to prevent anIndexOutOfBoundsException
. -
Can I get the first element from a list of different data types?
Yes, in Java, you can create a list that holds different object types using generics with theObject
class. However, this is not a common practice as it can lead to type safety issues. -
What happens if I try to get the first element from an empty list?
If you attempt to retrieve the first element from an empty list, it will throw anIndexOutOfBoundsException
forArrayList
and aNoSuchElementException
forLinkedList
.
-
Is there a method to remove the first element from a list?
Yes, bothArrayList
andLinkedList
provide methods to remove the first element. You can useremove(0)
forArrayList
andremoveFirst()
forLinkedList
. -
Are there performance differences between using ArrayList and LinkedList?
Yes,ArrayList
provides faster access times for indexed elements due to its underlying array structure. In contrast,LinkedList
is more efficient for insertions and deletions, especially at the beginning or end of the list.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn