How to Find the Index of an Element in a List Using Java
-
Method 1: Use the
indexOf()
Method to Find Index -
Method 2: Use the
Stream
API to Find Index - Method 3: Use Loop to Find Index
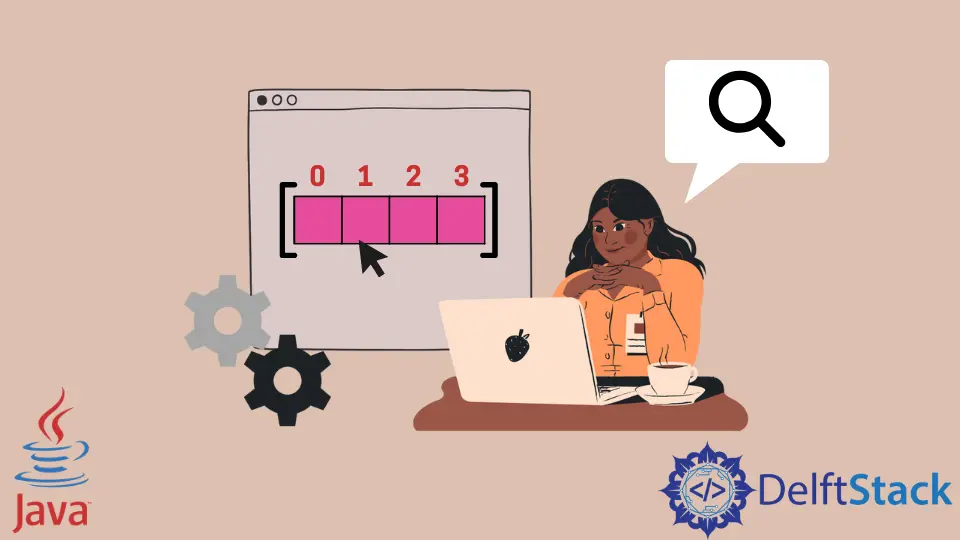
When working with lists, you may need to find the index of a specific element because, in some cases, finding the index becomes significant.
Suppose you are working with an employee
list and need to find a specific employee
serial number. In that case, you need to find that particular employee
index on the list.
In this article, we’ll learn how we can use the indexOf()
method, Stream
API and loop to find the index of a specific element of a list.
Method 1: Use the indexOf()
Method to Find Index
We can easily find the index of a specific list element using the indexOf()
method. You can follow the below example code with an explanation for this purpose.
Example Code:
// importing necessary packages
import java.util.List;
public class FindIndex {
public static void main(String[] args) {
// Creating a list
List<Integer> MyVal = List.of(5, 10, 4, 3, 6, 2, 80, 6);
// Specifying the item
int FindItem = 80;
// Finding the index
int Index = MyVal.indexOf(FindItem);
// printing the index for the specific item
System.out.println("The index is: " + Index);
}
}
Output:
The index is: 6
In the example, we first take a list named MyVal
and also take an item for which we are going to find an index. Then we passed it to the indexOf()
method with the item we are looking for.
It prints the index if the item is found on the list; otherwise, it returns -1
, which means the item we are looking for is not found.
Method 2: Use the Stream
API to Find Index
In this method, we will use the Stream
API to find the index of the specific element in a list. To use this approach, you can follow below example code and its explanation.
Example Code:
// importing necessary packages
import java.util.List;
import java.util.Objects;
import java.util.stream.IntStream;
public class FindIndex {
public static void main(String[] args) {
// Creating a list
List<Integer> MyVal = List.of(5, 10, 4, 3, 6, 2, 80, 6);
// Specifying the item
int FindItem = 80;
// Finding the index
int Index = IntStream.range(0, MyVal.size())
.filter(i -> Objects.equals(MyVal.get(i), FindItem))
.findFirst()
.orElse(-1);
// printing the index for the specific item
System.out.println("The index is: " + Index);
}
}
Output:
The index is: 6
In the above code fence, we first take a list named MyVal
and also take an item which index we are going to find.
Then we passed it to IntStream
to filter the elements and find the index of the element that we provided. We get the index if the item is in the MyVal
list; otherwise, we will get -1
as output.
Method 3: Use Loop to Find Index
We also can use loops (for
, while
, or do...while
based on project requirements) to find an index of a specific element in a list. To understand it, look at the example code below and its explanation.
Example Code:
// importing necessary packages
import java.util.List;
import java.util.Objects;
public class FindIndex {
public static void main(String[] args) {
// Creating a list
List<Integer> MyVal = List.of(5, 10, 4, 3, 6, 2, 80, 6);
// Specifying the item
int FindItem = 80;
int Index = -1;
// Finding the index
for (int i = 0; i < MyVal.size(); i++) {
if (Objects.equals(MyVal.get(i), FindItem)) {
Index = i;
break;
}
}
// printing the index for the specific item
System.out.println("The index is: " + Index);
}
}
Output:
The index is: 6
Here, we first take a list named MyVal
and also take an item for which we want to find an index. Then we iterate over a list using a loop and match every element with the item we are looking for.
Once we find the required item, save the index in a variable and break the loop to avoid further iterations. We can break a look using the break
keyword.
Like the previous two examples, this approach returns the index if a specified item is found in a list otherwise returns -1
.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn