How to Filter List in Java
- Filter a List Using Java 7 and Above
- Filter a List Using Java 8
- Filter a List Using Google Guava
- Filter a List Using Apache Commons
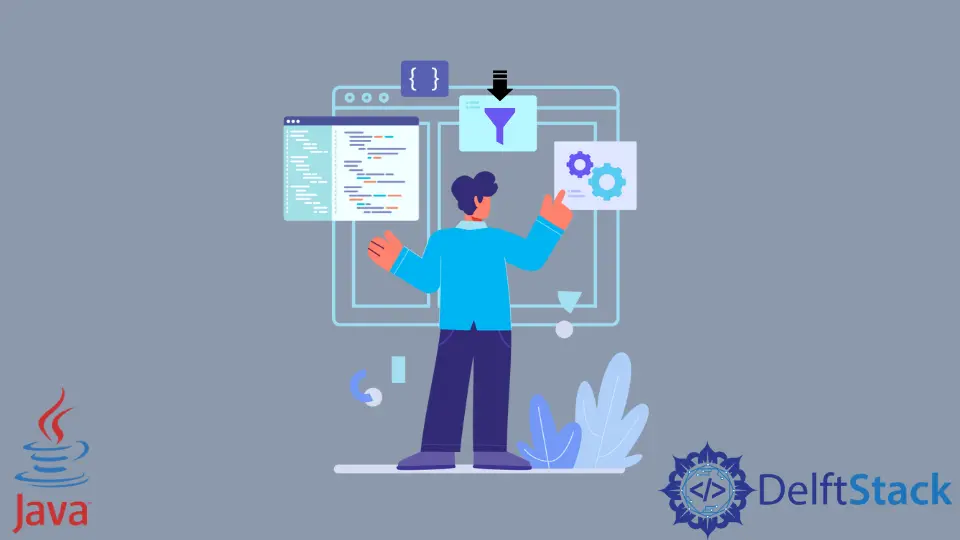
Filtering a list is frequently required while working in Java. Several methods to filter a list in Java use the core Java and different libraries.
This tutorial demonstrates how to filter a list in Java.
Filter a List Using Java 7 and Above
We can iterate through the list using Java 7 and the versions before to filter a list in Java.
Use the for
Loop
One method is to use the for
loop and conditional statement. Let’s see the example:
package delftstack;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<String> Demo_List = Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey");
// Using Java 7 and before:
// empty list
List<String> Filtered_List = new ArrayList<>();
// iterate through the list
for (String Demo_Entry : Demo_List) {
// filter values that start with `B`
if (Demo_Entry.startsWith("J")) {
Filtered_List.add(Demo_Entry);
}
}
System.out.println(Filtered_List);
}
}
The code above uses for
and if
to filter the names from the given list with the letter J
. See output:
[Jack, Jhon, Joey]
Use the remove()
Method
Another method is to use the remove()
method from the Iterator
class to filter the list. Let’s see the example:
package delftstack;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<String> Demo_List =
new ArrayList<>(Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey"));
// Using Java 7 and before:
// get an iterator to the list
Iterator<String> Demo_Iterator = Demo_List.iterator();
// iterate through the list
while (Demo_Iterator.hasNext()) {
String Demo = Demo_Iterator.next();
// filter values that start with `B`
if (!Demo.startsWith("J")) {
Demo_Iterator.remove();
}
}
System.out.println(Demo_List);
}
}
The code uses Iterator
with the remove()
method and the while
loop to filter the names with the letter J
from the given list. See output:
[Jack, Jhon, Joey]
Filter a List Using Java 8
To filter a list in Java 8, we can use the approach where we convert the list to a stream and then filter it. This is the recommended method to filter a list in Java 8.
See the example:
package delftstack;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> Demo_List =
new ArrayList<>(Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey"));
// Using Java 8 and above:
String Filtered_List = Demo_List.stream()
.filter(entry -> entry.startsWith("J"))
.collect(Collectors.joining(", ", "[", "]"));
System.out.println(Filtered_List);
}
}
The code above uses the stream()
to filter the list with the names with the letter J
. See output:
[Jack, Jhon, Joey]
As we can see, the above code converts the list to a string in the output. We can also convert the output to a List using the Stream
.
See the example:
package delftstack;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> Demo_List =
new ArrayList<>(Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey"));
// Using Java 8 and above:
List<String> Filtered_List =
Demo_List.stream().filter(entry -> entry.startsWith("J")).collect(Collectors.toList());
System.out.println(Filtered_List);
}
}
The code above will convert the filtered list output to a list. See output:
[Jack, Jhon, Joey]
Filter a List Using Google Guava
The Guava
library contains Iterable
and FluentIterable
that can be used to filter a list in Java. The Iterable
class includes the filter()
method, which filters the lists.
The Guava
library can be used by adding the following dependency to your Maven project:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>19.0</version>
</dependency>
Now let’s try an example using the Iterable
from the Google Guava library:
package delftstack;
import com.google.common.*;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> Demo_List =
new ArrayList<>(Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey"));
Iterable<String> Filtered_Iterable =
Iterables.filter(Demo_List, new com.google.common.base.Predicate<String>() {
@Override
public boolean apply(String s) {
return s.startsWith("J");
}
});
List<String> Filtered_List = Lists.newArrayList(Filtered_Iterable);
System.out.println(Filtered_List);
}
}
The code will filter the given list with names starting with the letter J
. See output:
[Jack, Jhon, Joey]
Let’s try an example with FluentIterable
:
package delftstack;
import com.google.common.*;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> Demo_List =
new ArrayList<>(Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey"));
List<String> Filtered_List = FluentIterable.from(Demo_List)
.filter(new com.google.common.base.Predicate<String>() {
@Override
public boolean apply(String s) {
return s.startsWith("J");
}
})
.toList();
System.out.println(Filtered_List);
}
}
The code above uses the FluentIterable
from the Google Guava library to filter the names from the list, which starts with the letter J
. See output:
[Jack, Jhon, Joey]
Filter a List Using Apache Commons
Apache Commons provides the class CollectionUtils
, which can be used to filter a list. To use Apache Commons, add the following dependency to your Maven project:
<dependency>
<groupId>commons-collections</groupId>
<artifactId>commons-collections</artifactId>
<version>3.2.2</version>
</dependency>
<dependency>
<groupId>commons-lang</groupId>
<artifactId>commons-lang</artifactId>
<version>2.6</version>
</dependency>
Now let’s try an example to filter a list using CollectionUtils
:
package delftstack;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import org.apache.commons.collections.CollectionUtils;
public class Example {
public static void main(String[] args) {
List<String> Demo_List =
new ArrayList<>(Arrays.asList("Jack", "Shawn", "Jhon", "Michelle", "Joey"));
List<String> Filtered_List = new ArrayList<>(Demo_List);
CollectionUtils.filter(Filtered_List, new Predicate<String>() {
@Override
public boolean evaluate(String s) {
return s.startsWith("J");
}
});
System.out.println(Filtered_List);
}
}
The code above uses the CollectionUtils
method to filter the list with the names starting with the letter J
. See output:
[Jack, Jhon, Joey]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook