List vs. Array in Java
- Differences in Syntax of Implementation in Java
-
ArrayList
Can Dynamically Grow While Arrays Are Static in Java -
ArrayList
Have Lots of Methods to Perform Operations While Array Does Not in Java
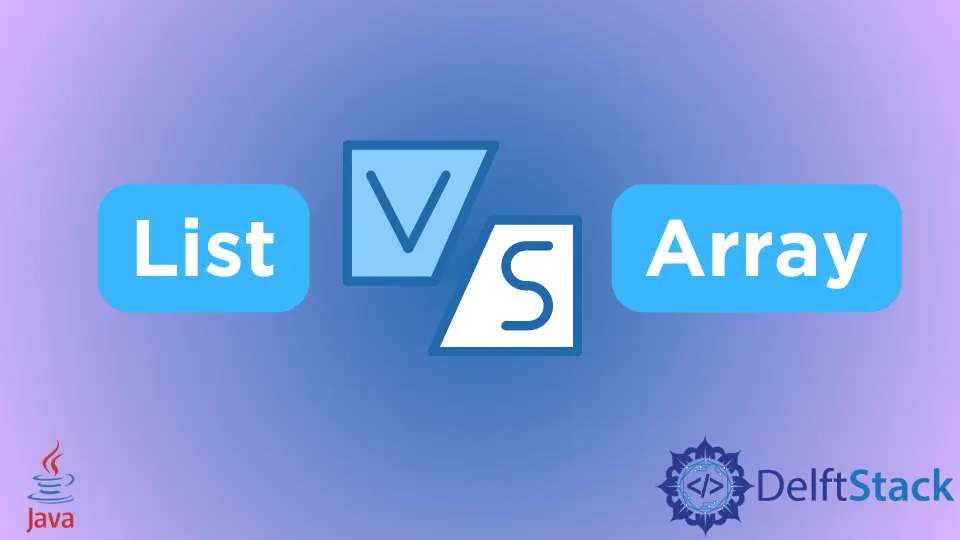
This article will show the differences between arrays and lists in Java. Both arrays and lists are widely used data structures in Java; hence, we need to understand when to use them.
ArrayList
is one of the most used lists, so, in the following sections, we will point out the differences between arrays and ArrayLists
.
Differences in Syntax of Implementation in Java
There are differences between the array and the ArrayList
when defining and initializing.
The syntax to declare and initialize an array is given below.
We first write the data type of elements to be stored in the array, and then we use brackets []
with the array name. We initialize the array using the new
keyword followed by the data type name and its size in the brackets.
type[] arrayName = new type[size];
Following is the syntax for declaring and initializing an ArrayList
.
We create an object of ArrayList
using the new
keyword and pass the type of data to be inserted that returns a List
that is an interface the ArrayList
uses.
List<Type> listName = new ArrayList<Type>();
The big difference between the two syntaxes is that the array uses primitive data types while ArrayLists
uses generic ones.
ArrayList
Can Dynamically Grow While Arrays Are Static in Java
When we declare an array, we need to specify the size that cannot be changed later, which means that arrays are static and have fixed sizes. In the following example, we create an array of int
type and specify the size as 4.
If we insert 4 items in the array, it will run, but adding another item (totaling five items) will throw an error in the output.
public class ExampleClass1 {
public static void main(String[] args) {
int[] intArray = new int[4];
intArray[0] = 1;
intArray[1] = 2;
intArray[2] = 3;
intArray[3] = 4;
intArray[4] = 5;
for (int i : intArray) {
System.out.println(i);
}
}
}
Output:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 4 out of bounds for length 4
at com.tutorial.ExampleClass1.main(ExampleClass1.java:13)
Unlike arrays, an ArrayList
can grow dynamically without any worry for size. Its size increases and decreases with the number of elements in it.
The below program contains an ArrayList
of type Integer
in which we add some items. The output shows all the elements printed from the list.
import java.util.ArrayList;
import java.util.List;
public class ExampleClass2 {
public static void main(String[] args) {
List<Integer> integerList = new ArrayList<>();
integerList.add(1);
integerList.add(2);
integerList.add(3);
integerList.add(4);
for (Integer integer : integerList) {
System.out.println(integer);
}
}
}
Output:
1
2
3
4
ArrayList
Have Lots of Methods to Perform Operations While Array Does Not in Java
We can add elements in an array, but there are limitations to performing other operations like removing. On the other hand, we can do several operations with an ArrayList
like removing, sorting, etc.
In the example, we create an ArrayList
and add some elements. Then we remove the element on the third index, the value 4.
The output shows the elements after removal. We need to use other classes to do this in an array, making it complicated.
public class ExampleClass2 {
public static void main(String[] args) {
List<Integer> integerList = new ArrayList<>();
integerList.add(1);
integerList.add(2);
integerList.add(3);
integerList.add(4);
integerList.remove(3);
for (Integer integer : integerList) {
System.out.println(integer);
}
}
}
Output:
1
2
3
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- Differences Between List and Arraylist in Java