How to Check if Int Is Null in Java
-
Can
int
Be Null in Java? -
Why Is It Important to Know if
Integer
Is Null? -
Can
int
Be Null in Java? - Best Practices for Handling Nullable Integers
- Conclusion
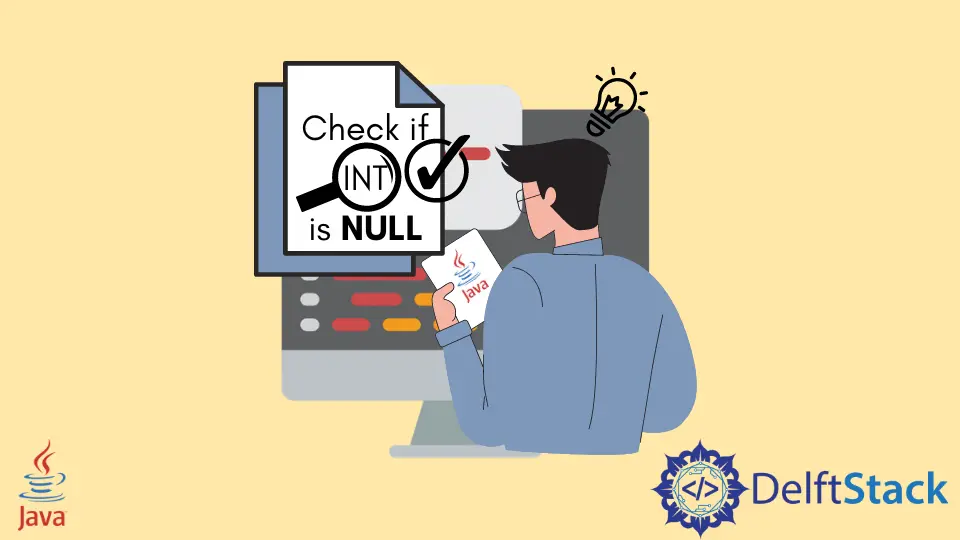
This article explores the method of checking for null values in Java’s int
type, delving into the utilization of the Integer
wrapper class. Additionally, it discusses essential best practices to ensure code reliability and robustness when dealing with nullable integers, offering insights into effective implementation strategies for developers.
Can int
Be Null in Java?
One thing that we need to understand first is that int
is a primitive data type.
Such data types store data in binary form in memory by default. That means they can’t be null.
In Java, the primitive data type int
cannot be assigned a value of null
because it is a value type, not an object. However, situations may arise where the need arises to represent the absence of a value for an integer-like entity.
Why Is It Important to Know if Integer
Is Null?
The Integer
class, which is a wrapper class for the int
primitive type, can be used to check if the value is null
. Understanding whether an Integer
object is null
becomes important in scenarios where the presence or absence of a value needs to be determined before performing operations on it.
Failing to check for null
before using the value of an Integer
object may lead to a NullPointerException
, a runtime exception in Java. By conscientiously checking for null
, developers can ensure the robustness and reliability of their code, preventing unexpected runtime errors and enabling the implementation of appropriate error-handling mechanisms for cases where a value is absent.
This practice contributes to the overall stability and correctness of Java programs, promoting a more secure and predictable software development process.
Can int
Be Null in Java?
In Java, the int
data type is a fundamental building block for numeric representations. Unlike its object-oriented counterparts, int
is a primitive data type and is not inherently nullable.
However, there are scenarios in which developers may need to handle the absence of a value for an integer-like entity.
This is where the Integer
wrapper class comes into play. You could also convert int
to Integer
.
In this section, we will delve into the concept of checking if an int
is null
using the Integer
wrapper class, exploring how it facilitates the representation of null values for integers.
Let’s begin with a complete working code example to illustrate the concept:
public class CheckIfIntIsNullExample {
public static void main(String[] args) {
// Part 1: Primitive int
int primitiveInt = 0;
System.out.println("Primitive int value: " + primitiveInt);
// Part 2: Nullable Integer
Integer nullableInt = null;
System.out.println("Nullable Integer value: " + nullableInt);
// Part 3: Checking for null
if (nullableInt != null) {
int intValue = nullableInt.intValue();
System.out.println("Value is: " + intValue);
} else {
System.out.println("Value is null");
}
}
}
In the first part of the code, we initialize a primitive int
variable named primitiveInt
with the value 0
, demonstrating a standard usage of a primitive integer. Moving on to the second part, we declare an Integer
object called nullableInt
and set it to null
, introducing the concept of nullable integers provided by the Integer
wrapper class.
Then, we employ an if
statement to check whether nullableInt
is null
.
If it is not null
, we extract the int
value using the intValue()
method and print it. On the other hand, if it is null
, we print a message indicating that the value is indeed null
.
This step-by-step explanation clarifies how the code utilizes the Integer
wrapper class to handle nullable integers and check for null values effectively.
Output:
The output showcases the int
value successfully printed, followed by the null
representation of the nullable Integer
, and finally, the acknowledgment of the null value through the conditional check. This reinforces the reliability and effectiveness of using the Integer
wrapper class to handle nullable integers in Java.
Best Practices for Handling Nullable Integers
Consistent Null Checking
Ensuring code reliability and robustness when handling nullable integers involves consistent and thorough null checking. Developers should diligently verify whether an Integer
object is null
before attempting to perform operations or extract its underlying int
value.
This practice minimizes the risk of encountering NullPointerExceptions
and enhances the overall stability of the code.
Clear Documentation
Documenting the handling of nullable integers is essential for code maintainability and collaboration. Clearly documenting where nullable integers are expected, how they should be handled, and the potential implications of null values provide valuable insights for other developers working on the codebase.
It also aids in preventing unintended misuse and promotes a better understanding of the code’s behavior.
Default Values and Initialization
When appropriate, consider providing default values for nullable integers to ensure that operations relying on them won’t unexpectedly fail. Initializing nullable integers to sensible defaults reduces the likelihood of null-related issues and enhances the predictability of the code’s behavior in different scenarios.
Use of Optional (Java 8 and Later)
In Java 8 and later versions, the Optional
class can be employed to handle nullable integers in a more expressive and functional manner. It offers methods for conditional execution of operations based on the presence or absence of a value, providing a clearer and more concise way to express intent in code.
Exception Handling
Implement robust exception handling mechanisms when dealing with nullable integers, especially in cases where the absence of a value is considered exceptional. By catching and handling exceptions appropriately, developers can prevent unexpected program termination and gracefully manage situations where nullable integers may lead to errors.
Conclusion
Leveraging the Integer
wrapper class for nullable integers enables effective null value checks, crucial for handling absences gracefully and preventing NullPointerExceptions
. Implementing best practices, such as consistent null checking, clear documentation, default values, and optional usage, ensures correct and resilient code, enhancing the reliability and robustness of Java applications.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn