How to Convert Int to Integer in Java
-
Importance of Converting
int
toInteger
in Java -
Convert
int
toInteger
in Java -
Best practices for Converting
int
toInteger
in Java - Conclusion
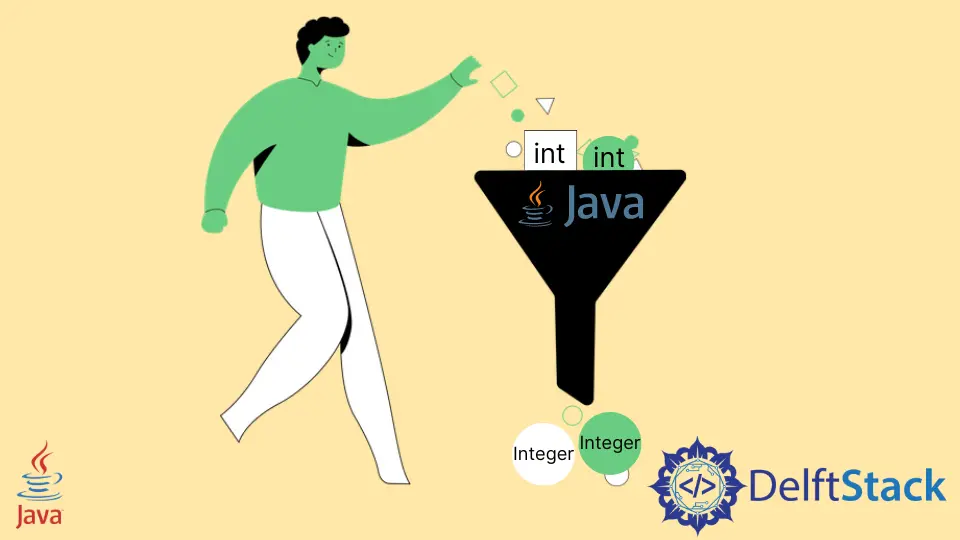
Explore the methods and best practices for converting int to Integer in Java, ensuring code readability and adherence to established conventions.
Java uses either a primitive int
type or Integer
wrapper class to hold integer values. If we want to convert a primitive int to an Integer
object, Java provides several methods.
Importance of Converting int
to Integer
in Java
Converting int
to Integer
in Java is crucial for bridging the gap between primitive data types and their corresponding wrapper classes, enabling compatibility with Java’s object-oriented features, collections, and APIs that often require objects rather than primitives. This conversion also facilitates enhanced functionality, such as utilizing null values and leveraging methods specific to the Integer
class, contributing to a more versatile and expressive code.
Convert int
to Integer
in Java
Auto-Boxing
Understanding auto-boxing is crucial for writing concise and readable Java code. By allowing seamless conversions between primitive types and their wrapper classes, auto-boxing simplifies the code and enhances its expressiveness.
Incorporating such practices in your codebase not only improves clarity but also contributes to a more maintainable and efficient code.
Let’s start with a practical example that demonstrates how to leverage auto-boxing to convert an int
to an Integer
.
public class IntToIntegerConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize an int primitive
int primitiveInt = 42;
// Step 2: Use auto-boxing to convert int to Integer
Integer wrapperInteger = primitiveInt;
// Step 3: Display the result
System.out.println("Primitive int: " + primitiveInt);
System.out.println("Converted Integer: " + wrapperInteger);
}
}
Let’s simplify the code breakdown into easy-to-understand steps.
In the first step, we declare and initialize an int
variable called primitiveInt
with the value 42
. This variable represents the primitive integer that we aim to convert to its wrapper class.
Moving on to the second step, we make use of auto-boxing. By directly assigning the int
variable primitiveInt
to an Integer
variable named wrapperInteger
, Java automatically wraps the primitive int
value in an Integer
object, simplifying the conversion process.
Finally, in the third step, we present the results. The initial println
statement outputs the original primitive int
, while the subsequent one showcases the Integer
object obtained through auto-boxing.
These three steps succinctly illustrate the conversion of an int
to an Integer
using auto-boxing in Java.
Output:
Primitive int: 42
Converted Integer: 42
The output confirms that the auto-boxing process successfully converted the int
primitive to its Integer
equivalent, highlighting the effectiveness of this approach in Java programming.
Integer Constructor
Utilizing the Integer
constructor method for converting int
to Integer
provides a direct and explicit approach to handle such conversions in Java. While it may involve a bit more verbosity compared to auto-boxing, this method offers control over the process and can be useful in scenarios requiring explicit object creation.
Let’s begin with a practical example showcasing the use of the Integer
constructor for the conversion.
public class IntToIntegerConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize an int primitive
int primitiveInt = 42;
// Step 2: Use Integer constructor to convert int to Integer
Integer wrapperInteger = new Integer(primitiveInt);
// Step 3: Display the result
System.out.println("Primitive int: " + primitiveInt);
System.out.println("Converted Integer: " + wrapperInteger);
}
}
Let’s break down the code into a simple narrative, examining each part sequentially.
In the initial step, we declare and initialize an int
variable named primitiveInt
with the value 42
. This variable signifies the primitive integer that we intend to transform into its wrapper class.
Moving on to the second step, we use the Integer
constructor. By instantiating a new Integer
object and passing the primitive int
value (primitiveInt
) as an argument, we perform an explicit conversion.
This construction allows us to convert the primitive int
to its corresponding Integer
object.
Lastly, we present the results. The first println
statement outputs the original primitive int
, and the subsequent one exhibits the Integer
object created through the constructor method.
This three-step process provides a clear overview of how the conversion from int
to Integer
unfolds using the Integer
constructor in Java.
Output:
Primitive int: 42
Converted Integer: 42
The output confirms the successful conversion of the int
primitive to its Integer
counterpart using the Integer
constructor method. This example illustrates the precision and clarity that this approach brings to the conversion process in Java programming.
valueOf()
Method
The valueOf
method, nestled within the Integer
class, streamlines the conversion of int
primitives to Integer
objects in Java. Beyond its simplicity, this method allows for optimizations such as caching commonly used values, contributing to improved performance.
This approach not only simplifies the conversion process but also offers additional functionalities.
Let’s dive into a practical example that demonstrates the application of the valueOf
method for converting an int
to an Integer
.
public class IntToIntegerConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize an int primitive
int primitiveInt = 42;
// Step 2: Use valueOf method to convert int to Integer
Integer wrapperInteger = Integer.valueOf(primitiveInt);
// Step 3: Display the result
System.out.println("Primitive int: " + primitiveInt);
System.out.println("Converted Integer: " + wrapperInteger);
}
}
Let’s walk through the code, breaking it down to enhance comprehension.
In the first step, we declare and initialize an int
variable named primitiveInt
with the value 42
. This variable represents the primitive integer that we aim to convert to its wrapper class.
Moving to the second step, we utilize the valueOf
method from the Integer
class. By providing the primitive int
value (primitiveInt
) as an argument, this method produces an Integer
object representing the same numeric value.
The valueOf
method serves as a concise and effective means for the conversion process.
Finally, in the third step, we display the results. The initial println
statement outputs the original primitive int
, while the subsequent one showcases the Integer
object obtained through the valueOf
method.
This three-step process succinctly demonstrates the conversion of an int
to an Integer
using the valueOf
method in Java.
Output:
Primitive int: 42
Converted Integer: 42
The output confirms the successful conversion of the int
primitive to its Integer
counterpart using the valueOf
method. This example illuminates the elegance and efficiency that this method brings to the table, showcasing its utility in Java programming.
Best practices for Converting int
to Integer
in Java
Code Readability
Ensure that the chosen conversion method aligns with the overall readability of your code. Opt for approaches that enhance clarity and maintainability.
Performance Impact
Be mindful of performance considerations, especially in scenarios involving frequent conversions. Evaluate the trade-offs between simplicity and efficiency.
Contextual Usage
Choose the conversion method based on the specific context of your code. Auto-boxing may be succinct, but explicit methods like the constructor or valueOf
offer more control in certain situations.
Consistency in Codebase
Promote consistency within your codebase by using a uniform approach for similar conversion tasks. This simplifies code reviews and makes the codebase more predictable.
Error Handling
Implement appropriate error handling mechanisms, especially when dealing with user inputs or external data. Ensure that the conversion method chosen is robust in handling potential errors.
Future Maintenance
Consider the long-term implications of your choice on future maintenance. Opt for the method that aligns with the project’s scalability and evolving requirements.
Performance Optimization
If performance is a critical concern, explore optimizations such as caching for repeated conversions. Utilize techniques that align with the specific performance needs of your application.
Coding Standards
Adhere to coding standards and best practices established within your development team or organization. Consistent coding standards contribute to a cohesive and collaborative coding environment.
Code Reviews
Engage in code reviews to gather feedback on the chosen conversion method. Leverage the collective expertise of the team to refine and improve the codebase.
Documentation
Provide clear documentation for conversion points, indicating the rationale behind the chosen method. This aids not only in code understanding but also facilitates knowledge transfer among team members.
Test Coverage
Ensure comprehensive test coverage for code sections involving conversions. Verify that the chosen method behaves as expected in various scenarios and edge cases.
Evaluate Language Features
Stay abreast of updates in Java and evaluate new language features that may impact conversion methods. Adopt new practices that align with evolving language capabilities.
Contextual Optimization
Optimize your approach based on the specific needs of your application. There is no one-size-fits-all solution, so tailor your method to the unique requirements of your code.
Continuous Learning
Foster a culture of continuous learning within the development team. Encourage exploration of new techniques and methods to improve code quality over time.
Conclusion
In Java, converting int
to Integer
is a common task with various methods available, including auto-boxing, the Integer
constructor, and the valueOf
method. Each method serves its purpose, but the simplicity of auto-boxing and the efficiency of valueOf
often make them preferable choices.
When converting, consider readability and performance, opting for the approach that aligns with your coding style and specific requirements. Embracing these techniques enhances code clarity and fosters better practices in Java development.