How to Handle Integer Overflow and Underflow in Java
- Overview of Integer Overflow and Underflow in Java
- Integer Overflow in Java
- Integer Underflow in Java
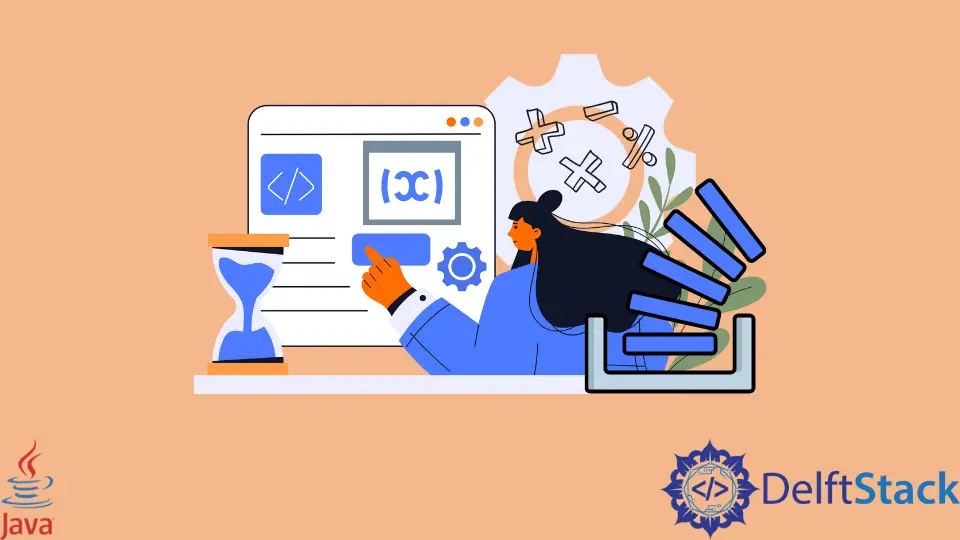
This article describes the overflow and underflow of an integer data type and the handling of this problem.
Overview of Integer Overflow and Underflow in Java
You may face an overflow or underflow error if you work with integer values. It happens when we declare a variable wrongly, like assigning a value that is out of range for the declared data type.
As we know, there are four levels of integer data types containing different sizes that are given below:
Data Type | Size |
---|---|
byte |
8-bits |
short |
16-bits |
int |
32-bits |
long |
64-bits |
If we consider the int
in Java, it has a range of values that we can assign. As we already know, that int
data type is 32-bits
in size; so, the range of accepted values in int
is between -2147483648
to 2147483647
.
You can quickly get the maximum and minimum value by using Integer.MAX_VALUE
and Integer.MIN_VALUE
.
Integer Overflow in Java
Here, we will see the occurrence of Integer overflow and its handling.
Occurrence of Integer Overflow
Example Code:
public class IntegerOverflow {
public static void main(String[] args) {
int Intvalue = Integer.MAX_VALUE - 1;
for (int i = 0; i < 4; i++) {
System.out.println(Intvalue);
Intvalue = Math.addExact(Intvalue, 1);
}
}
}
Now when you execute the above program, the console will show your the below output:
2147483646
2147483647
Exception in thread "main" java.lang.ArithmeticException: integer overflow
at java.base/java.lang.Math.addExact(Math.java:828)at IntegerOverflow.main(IntegerOverflow.java:9)
Handle Integer Overflow
We need to increase the value range to solve our example above. In our case, we typecast the integer
to long
. Our updated code for the above example will be as follows.
Example Code:
public class IntegerOverflow {
public static void main(String[] args) {
int Intvalue = Integer.MAX_VALUE - 1;
long NewValue = (long) Intvalue; // Upgrading to new type
for (int i = 0; i < 4; i++) {
System.out.println(NewValue);
NewValue = Math.addExact(NewValue, 1);
}
}
}
Now you will see the below output in your console.
2147483646
2147483647
2147483648
2147483649
Integer Underflow in Java
In this section, we will learn about Integer underflow in Java and how we can handle it.
Occurrence of Integer Underflow
Example Code:
public class IntegerUnderflow {
public static void main(String[] args) {
int value = -2147483699; // Creating underflow intentionally
System.out.println(value - 1);
}
}
Now when you execute the above program, the console will show your the below output:
/IntegerUnderflow.java:3: error: integer number too large
int value = -2147483699; // Creating underflow intentionally
^
1 error
Handle Integer Underflow
To solve the error above, we need to increase the range of the value again, and to do that, we can typecast the integer value to the next level. Have a look at the code shared below.
Example Code:
public class IntegerUnderflow {
public static void main(String[] args) {
long value = (long) -2147483638; // Type Casting
System.out.println(value - 1);
}
}
Now you will see the below output in your console.
-2147483639
So, we need to increase the range to the data type in case of solving the overflow or underflow problems.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack