How to Convert Int to Binary in Java
-
Convert Int to Binary Using
Integer.toBinaryString()
in Java -
Convert Int to Binary Using
Integer.toString()
in Java -
Convert Int to Binary Using
StringBuilder
and a Loop in Java
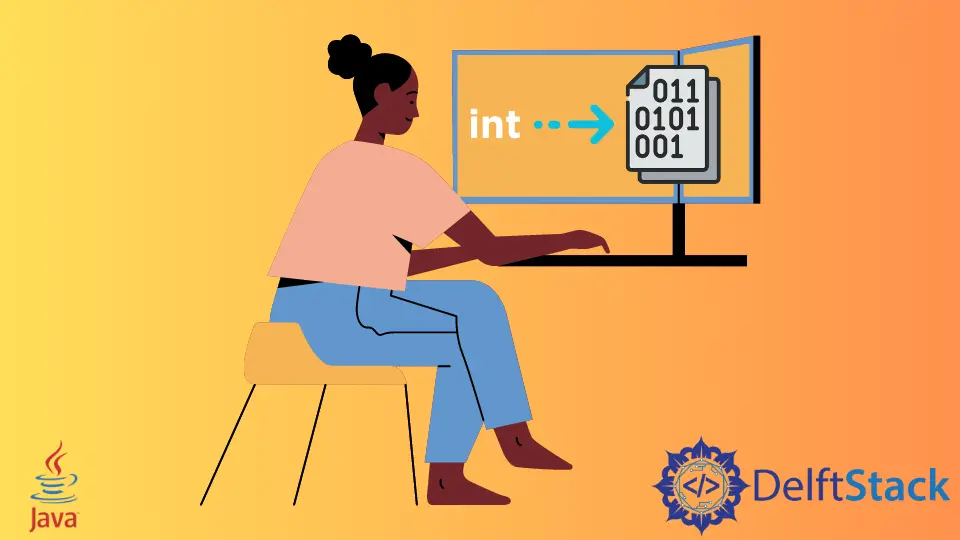
A Binary Number is represented with two binary digits, the 0
and 1
. We can convert an int
value to a binary value in Java using the three methods listed below.
Convert Int to Binary Using Integer.toBinaryString()
in Java
The most common and easiest way to convert an int
value to binary is to use the toBinaryString()
function of the Integer
class. Integer.toBinaryString()
takes an argument of int
type.
In the program, we store an int
value in a variable numInt
and then pass it as an argument in the Integer.toBinaryString()
method that returns a String
.
public class JavaExample {
public static void main(String[] args) {
int numInt = 150;
String binaryString = Integer.toBinaryString(numInt);
System.out.println(binaryString);
}
}
Output:
10010110
Convert Int to Binary Using Integer.toString()
in Java
In this example, we use another method of the Integer
class method: the toString()
method.
Integer.toString()
takes two arguments in which the second argument is optional. The first argument is the value to convert to a String
, and the second argument is the radix value for conversion.
For our program, we need to use both arguments of the toString()
function to specify the radix 2
, representing the binary digits 0
and 1
. In simple words, when we use radix 2
, the int
is converted to a String
value that represents 0
s and 1
s only.
We print the result that is a binary representation of numInt
.
public class JavaExample {
public static void main(String[] args) {
int numInt = 200;
String binaryString = Integer.toString(numInt, 2);
System.out.println(binaryString);
}
}
Output:
11001000
Convert Int to Binary Using StringBuilder
and a Loop in Java
The last program takes a traditional approach; instead of using an inbuilt function to convert the int
value to a binary, we create our function that does the same job.
In the below code, we create a function convertIntToBinary()
that receives the int
value as parameter to convert. We set the return type of the function as String.
Inside the convertIntToBinary()
method, we first check if the int
variable numInt
is holding a zero or not. If it is, we return 0
because the binary representation of 0
in int
is also 0
. If it is a non-zero integer value, we create a StringBuilder
class and a while
loop.
We run the loop until numInt
is greater than zero. In the loop, we perform three steps; the first is to find the remainder of the numInt
using numInt % 2
and then append the value of remainder
to the StringBuilder
.
For the last step, we divide the numInt
value with 2
and store it in numInt
itself. Once we have performed all the steps and are out of the loop, we reverse the stringBuilder
value to get the correct result and return the result after converting the stringBuilder
value to a String
.
In the main()
method, we take the user’s input and pass it to the convertIntToBinary()
method that returns the binary result.
import java.util.Scanner;
public class JavaExample {
public static void main(String[] args) {
System.out.println("Enter a number to convert it to a binary: ");
Scanner scanner = new Scanner(System.in);
int getIntNum = scanner.nextInt();
String getConvertedResult = convertIntToBinary(getIntNum);
System.out.println("Converted Binary: " + getConvertedResult);
}
static String convertIntToBinary(int numInt) {
if (numInt == 0)
return "0";
StringBuilder stringBuilder = new StringBuilder();
while (numInt > 0) {
int remainder = numInt % 2;
stringBuilder.append(remainder);
numInt /= 2;
}
stringBuilder = stringBuilder.reverse();
return stringBuilder.toString();
}
}
Output:
Enter a number to convert it to a binary:
150
Converted Binary: 10010110
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Integer
- How to Handle Integer Overflow and Underflow in Java
- How to Reverse an Integer in Java
- The Max Value of an Integer in Java
- Minimum and Maximum Value of Integer in Java
- How to Convert Integer to Int in Java