Binary Number Addition in Java
- Understanding Binary Numbers
- Method 1: Using Integer.parseInt and Integer.toBinaryString
- Method 2: Manual Binary Addition
- Conclusion
- FAQ
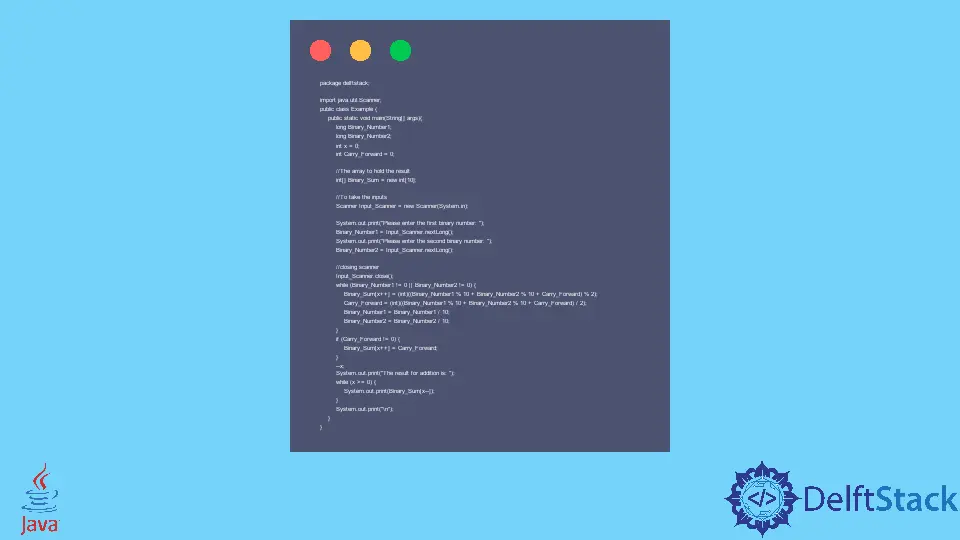
Binary addition is a fundamental concept in computer science and programming, especially when working with low-level data processing. In Java, performing binary addition allows developers to manipulate binary data directly, which can be crucial for applications that require efficiency or direct hardware interaction.
This tutorial will guide you through the process of binary number addition in Java, illustrating how to implement it effectively. Whether you’re a beginner looking to grasp the basics or an experienced programmer wanting to refresh your skills, this guide will provide clear examples and explanations to help you succeed. Let’s dive into the world of binary addition!
Understanding Binary Numbers
Before we jump into the code, it’s essential to understand what binary numbers are. Binary is a base-2 numeral system that uses only two digits: 0 and 1. Each digit in a binary number is referred to as a bit. For example, the binary number 1011
represents the decimal number 11
. In Java, numbers are typically represented in decimal format, but you can perform operations on them in binary format as well.
To illustrate binary addition, consider the following example:
1011 (11 in decimal)
+ 1101 (13 in decimal)
-------
11000 (24 in decimal)
In this example, binary addition follows the same principles as decimal addition, with the key difference being how carries are handled. Now, let’s see how to implement binary addition in Java.
Method 1: Using Integer.parseInt and Integer.toBinaryString
One straightforward way to perform binary addition in Java is to leverage built-in methods like Integer.parseInt
and Integer.toBinaryString
. This method allows you to convert binary strings into integers, perform addition, and then convert the result back into a binary string.
Here’s how you can do it:
public class BinaryAddition {
public static void main(String[] args) {
String binary1 = "1011";
String binary2 = "1101";
int sum = Integer.parseInt(binary1, 2) + Integer.parseInt(binary2, 2);
String binarySum = Integer.toBinaryString(sum);
System.out.println("Sum: " + binarySum);
}
}
Output:
Sum: 11000
In this code, we first define two binary strings, binary1
and binary2
. The Integer.parseInt
method converts these binary strings into integers by specifying the base as 2
. We then add these integers together and use Integer.toBinaryString
to convert the sum back into a binary string. Finally, we print the result, which in this case is 11000
.
This method is efficient and leverages Java’s built-in functionality for handling binary numbers. It is particularly useful for quick calculations without getting into the nitty-gritty of binary arithmetic.
Method 2: Manual Binary Addition
For those who prefer a more hands-on approach, you can implement binary addition manually. This method mimics the traditional method of adding binary numbers, handling carries as necessary. Here’s how to do it:
public class ManualBinaryAddition {
public static void main(String[] args) {
String binary1 = "1011";
String binary2 = "1101";
String result = addBinary(binary1, binary2);
System.out.println("Sum: " + result);
}
public static String addBinary(String a, String b) {
StringBuilder sb = new StringBuilder();
int carry = 0;
int i = a.length() - 1, j = b.length() - 1;
while (i >= 0 || j >= 0 || carry > 0) {
int sum = carry;
if (i >= 0) sum += a.charAt(i--) - '0';
if (j >= 0) sum += b.charAt(j--) - '0';
sb.append(sum % 2);
carry = sum / 2;
}
return sb.reverse().toString();
}
}
Output:
Sum: 11000
In this implementation, we define a method called addBinary
that takes two binary strings as input. We use a StringBuilder
to build the resulting binary number. We keep track of the current indices of the binary strings and a carry value. As we iterate through the strings from right to left, we calculate the sum of the corresponding bits and the carry. The result is appended to the StringBuilder
, which we reverse at the end to get the final binary sum.
This manual method provides a deeper understanding of how binary addition works and is a great exercise for those looking to strengthen their programming skills.
Conclusion
In this tutorial, we explored how to perform binary addition in Java through two distinct methods: using built-in Java methods and implementing manual binary addition. Both approaches have their merits, with the first being quick and efficient, while the latter offers a deeper understanding of binary arithmetic. Whether you’re working on low-level programming or just looking to enhance your Java skills, mastering binary addition is a valuable asset. We hope this guide has been helpful and encourages you to explore more about binary operations in Java.
FAQ
-
What is binary addition?
Binary addition is the process of adding two binary numbers together, similar to decimal addition but with only two digits: 0 and 1. -
Why is binary addition important in programming?
Binary addition is crucial in programming because computers operate using binary data. Understanding binary operations allows developers to work more efficiently with low-level data processing. -
Can I add more than two binary numbers in Java?
Yes, you can add more than two binary numbers by iteratively adding them using either method discussed in this tutorial. -
What are some common applications of binary addition?
Binary addition is commonly used in digital electronics, computer programming, and algorithms related to data processing and manipulation. -
Is there a built-in method for binary addition in Java?
While Java does not have a dedicated method for binary addition, you can useInteger.parseInt
andInteger.toBinaryString
to perform binary addition efficiently.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook