Java의 이진수 추가
Sheeraz Gul
2023년10월12일
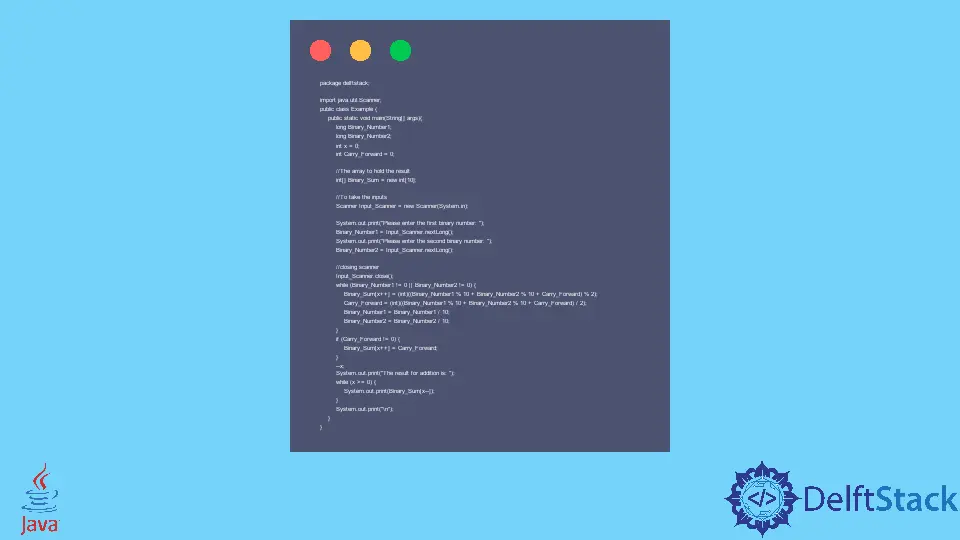
이 자습서는 Java에서 이진 추가를 수행하는 방법을 보여줍니다.
Java의 이진수 추가
이진수는 1과 0의 조합으로 산술수와 동일하게 더해지는 것이 아니다. 이진수의 덧셈 규칙은 다음과 같습니다.
0 + 0 = 0
0 + 1 = 1
1 + 0 = 1
1 + 1 = 10. For this, the 1 will carry forward.
1 (carry forwarded) + 1 + 1 = 11 and the 1 will carry forward again.
이진 추가를 수행할 수 있는 이러한 규칙을 기반으로 프로그램을 구현해 봅시다.
package delftstack;
import java.util.Scanner;
public class Example {
public static void main(String[] args) {
long Binary_Number1;
long Binary_Number2;
int x = 0;
int Carry_Forward = 0;
// The array to hold the result
int[] Binary_Sum = new int[10];
// To take the inputs
Scanner Input_Scanner = new Scanner(System.in);
System.out.print("Please enter the first binary number: ");
Binary_Number1 = Input_Scanner.nextLong();
System.out.print("Please enter the second binary number: ");
Binary_Number2 = Input_Scanner.nextLong();
// closing scanner
Input_Scanner.close();
while (Binary_Number1 != 0 || Binary_Number2 != 0) {
Binary_Sum[x++] = (int) ((Binary_Number1 % 10 + Binary_Number2 % 10 + Carry_Forward) % 2);
Carry_Forward = (int) ((Binary_Number1 % 10 + Binary_Number2 % 10 + Carry_Forward) / 2);
Binary_Number1 = Binary_Number1 / 10;
Binary_Number2 = Binary_Number2 / 10;
}
if (Carry_Forward != 0) {
Binary_Sum[x++] = Carry_Forward;
}
--x;
System.out.print("The result for addition is: ");
while (x >= 0) {
System.out.print(Binary_Sum[x--]);
}
System.out.print("\n");
}
}
위의 코드는 이진수의 두 입력을 받아 더합니다. 출력을 참조하십시오.
Please enter the first binary number: 1010101
Please enter the second binary number: 111111
The result for addition is: 10010100
작가: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook