Addition von Binärzahlen in Java
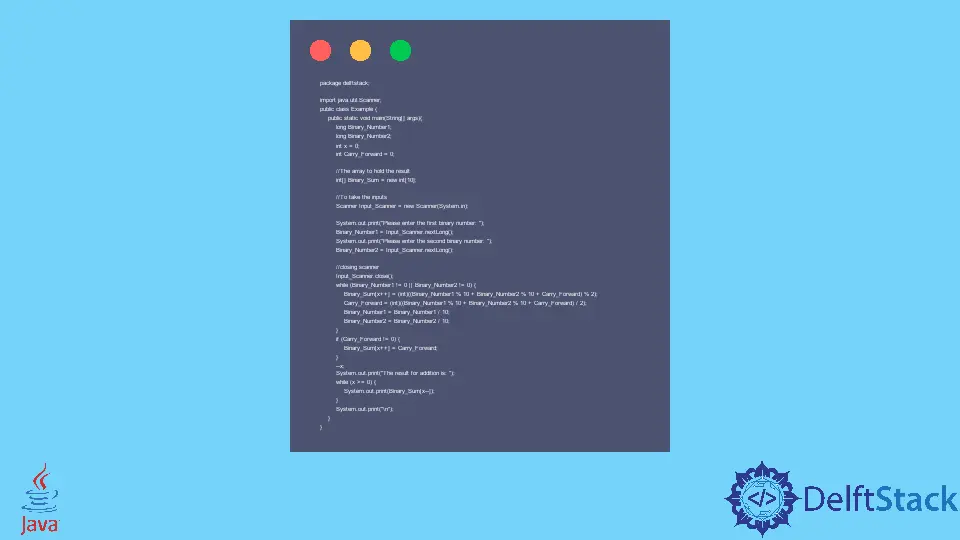
Dieses Tutorial zeigt, wie man eine binäre Addition in Java durchführt.
Addition von Binärzahlen in Java
Die Binärzahlen sind die Kombinationen von 1 und 0 und werden nicht wie die arithmetischen Zahlen addiert. Die Regeln für die Addition von Binärzahlen lauten:
0 + 0 = 0
0 + 1 = 1
1 + 0 = 1
1 + 1 = 10. For this, the 1 will carry forward.
1 (carry forwarded) + 1 + 1 = 11 and the 1 will carry forward again.
Lassen Sie uns versuchen, ein Programm basierend auf diesen Regeln zu implementieren, das eine binäre Addition durchführen kann:
package delftstack;
import java.util.Scanner;
public class Example {
public static void main(String[] args) {
long Binary_Number1;
long Binary_Number2;
int x = 0;
int Carry_Forward = 0;
// The array to hold the result
int[] Binary_Sum = new int[10];
// To take the inputs
Scanner Input_Scanner = new Scanner(System.in);
System.out.print("Please enter the first binary number: ");
Binary_Number1 = Input_Scanner.nextLong();
System.out.print("Please enter the second binary number: ");
Binary_Number2 = Input_Scanner.nextLong();
// closing scanner
Input_Scanner.close();
while (Binary_Number1 != 0 || Binary_Number2 != 0) {
Binary_Sum[x++] = (int) ((Binary_Number1 % 10 + Binary_Number2 % 10 + Carry_Forward) % 2);
Carry_Forward = (int) ((Binary_Number1 % 10 + Binary_Number2 % 10 + Carry_Forward) / 2);
Binary_Number1 = Binary_Number1 / 10;
Binary_Number2 = Binary_Number2 / 10;
}
if (Carry_Forward != 0) {
Binary_Sum[x++] = Carry_Forward;
}
--x;
System.out.print("The result for addition is: ");
while (x >= 0) {
System.out.print(Binary_Sum[x--]);
}
System.out.print("\n");
}
}
Der obige Code nimmt zwei Eingaben der Binärzahlen und addiert sie. Siehe die Ausgabe:
Please enter the first binary number: 1010101
Please enter the second binary number: 111111
The result for addition is: 10010100
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook