Java での 2 進数の加算
Sheeraz Gul
2023年10月12日
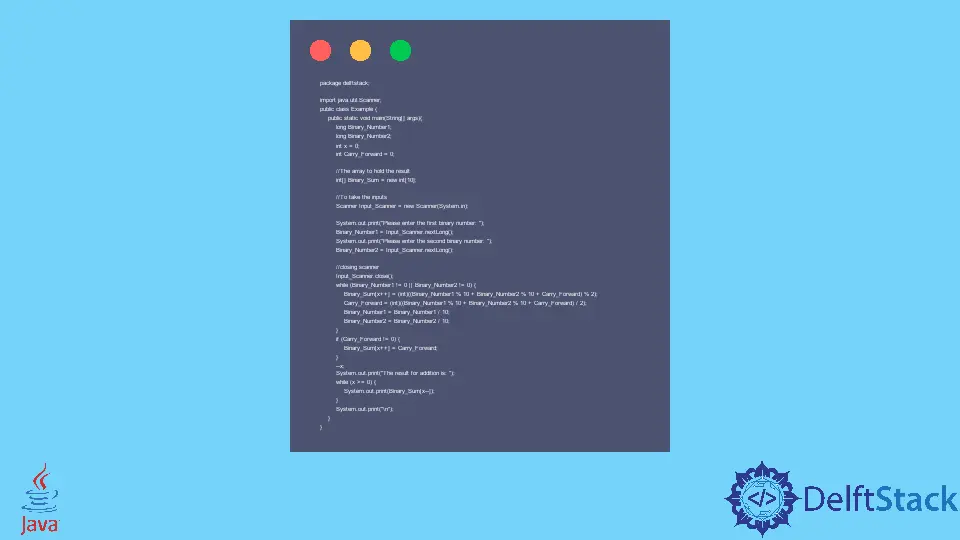
このチュートリアルでは、Java でバイナリ加算を実行する方法を示します。
Java での 2 進数の加算
2 進数は 1 と 0 の組み合わせであり、算術数と同じように加算されません。 2 進数の足し算のルールは次のとおりです。
0 + 0 = 0
0 + 1 = 1
1 + 0 = 1
1 + 1 = 10. For this, the 1 will carry forward.
1 (carry forwarded) + 1 + 1 = 11 and the 1 will carry forward again.
バイナリ加算を実行できるこれらのルールに基づいてプログラムを実装してみましょう。
package delftstack;
import java.util.Scanner;
public class Example {
public static void main(String[] args) {
long Binary_Number1;
long Binary_Number2;
int x = 0;
int Carry_Forward = 0;
// The array to hold the result
int[] Binary_Sum = new int[10];
// To take the inputs
Scanner Input_Scanner = new Scanner(System.in);
System.out.print("Please enter the first binary number: ");
Binary_Number1 = Input_Scanner.nextLong();
System.out.print("Please enter the second binary number: ");
Binary_Number2 = Input_Scanner.nextLong();
// closing scanner
Input_Scanner.close();
while (Binary_Number1 != 0 || Binary_Number2 != 0) {
Binary_Sum[x++] = (int) ((Binary_Number1 % 10 + Binary_Number2 % 10 + Carry_Forward) % 2);
Carry_Forward = (int) ((Binary_Number1 % 10 + Binary_Number2 % 10 + Carry_Forward) / 2);
Binary_Number1 = Binary_Number1 / 10;
Binary_Number2 = Binary_Number2 / 10;
}
if (Carry_Forward != 0) {
Binary_Sum[x++] = Carry_Forward;
}
--x;
System.out.print("The result for addition is: ");
while (x >= 0) {
System.out.print(Binary_Sum[x--]);
}
System.out.print("\n");
}
}
上記のコードは、2 進数の 2つの入力を取り、それらを加算します。 出力を参照してください。
Please enter the first binary number: 1010101
Please enter the second binary number: 111111
The result for addition is: 10010100
著者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook