How to Read Binary Files in Java
-
Read Binary File Using
FileInputStream
in Java -
Read Binary File Using
BufferedInputStream
in Java -
Read Binary File Using
InputStreamReader
in Java
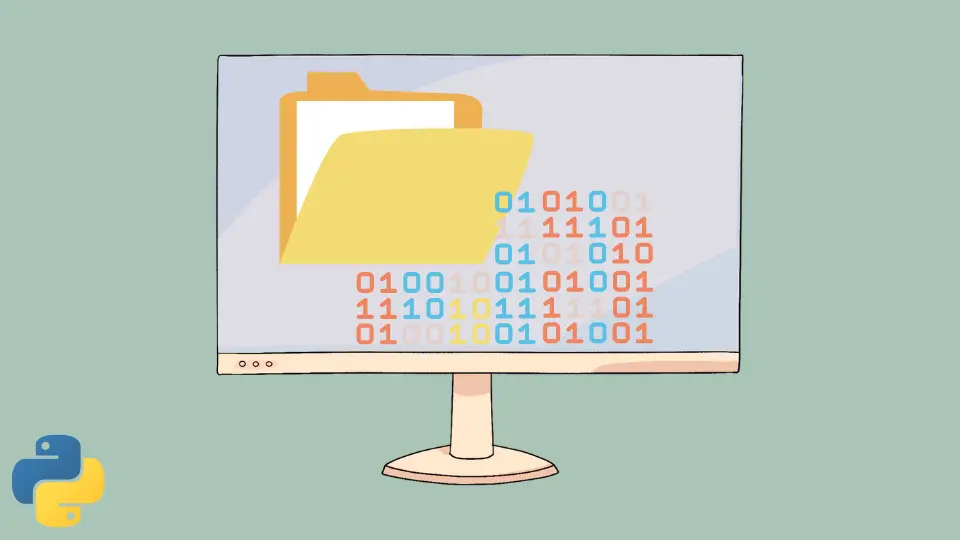
Reading a binary file can be difficult while trying to open it in software, but we can read Binary files using Java.
This tutorial demonstrates different ways of reading binary files in Java.
Read Binary File Using FileInputStream
in Java
Using the FileInputStream
class in Java, we can easily read the binary files in Java.
Code:
package Delfstack;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class Read_Binary {
public static void main(String[] args) {
try {
// create a reader for data file
FileInputStream read = new FileInputStream(new File("input.dat"));
// the variable will be used to read one byte at a time
int byt;
while ((byt = read.read()) != -1) {
System.out.print((char) byt);
}
read.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
We created an input.dat
binary and then tried to read it through Java.
Output:
default: (
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% Test input.dat file from Delftstack
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% check = true
check = false
)
The program above only reads one byte at a time, so that it may take more time for the large binary files.
Read Binary File Using BufferedInputStream
in Java
As mentioned above, FileInputStream
can only read one byte at a time, which may take more time to read large files. To solve that problem, we use the BufferedInputStream
class.
The BufferedInputStream
class reads a set of bytes at a time into an array buffer.
Code:
package Delfstack;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class Read_Binary {
public static void main(String[] args) {
try {
int Size_Buffer = 16 * 1024; // 16kb
// create a reader for data file
FileInputStream read = new FileInputStream(new File("input.dat"));
BufferedInputStream buffered_reader = new BufferedInputStream(read, Size_Buffer);
int byt;
while ((byt = buffered_reader.read()) != -1) {
System.out.print((char) byt);
}
buffered_reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The default internal buffer size is 8 kb which can be changed by passing as a parameter to the BufferedInputStream
.
Output:
default: (
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% Test input.dat file from Delftstack
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% check = true
check = false
)
Read Binary File Using InputStreamReader
in Java
The InputStreamReader
is used to read binary files with encoding different than our operating system. The encoding of the binary file is passed as a parameter to InputStreamReader
.
Code:
package Delfstack;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
public class Read_Binary {
public static void main(String[] args) {
try {
FileInputStream read = new FileInputStream(new File("input.dat"));
// pass the UTF_8 character encoding
InputStreamReader stream_reader = new InputStreamReader(read, StandardCharsets.UTF_8);
int byt;
while ((byt = stream_reader.read()) != -1) {
System.out.print((char) byt);
}
stream_reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above can read the binary files with a particular encoding. Pass that encoding to the InputStreamReader
as a parameter.
Output:
default: (
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% Test input.dat file from Delftstack
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% check = true
check = false
)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook