How to Convert Integer to Int in Java
-
Use Auto-Unboxing to Convert
Integer
toint
in Java -
Use the
intValue()
Method to ConvertInteger
toint
in Java 1.4 or Lower -
Use the Ternary Operator With the
intValue()
Method for Safe Conversion ofInteger
toint
in Java -
Use the
parseInt()
Method to ConvertInteger
toint
in Java -
Use the
valueOf()
Method and Auto-Unboxing to ConvertInteger
toint
in Java -
Use Casting to Convert
Integer
toint
in Java -
Use
Objects.requireNonNull()
With Auto-Unboxing orintValue()
to ConvertInteger
toint
in Java - Conclusion
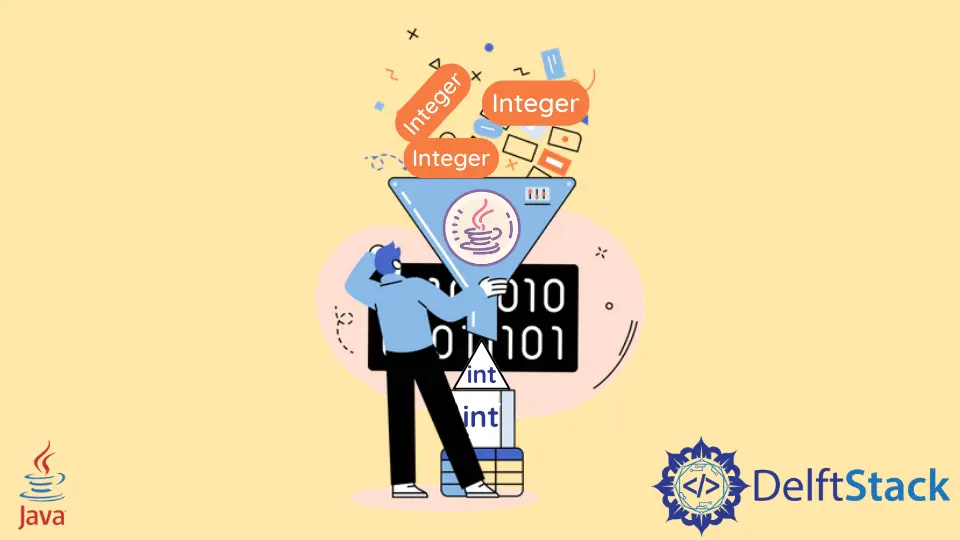
Java, being a versatile and object-oriented programming language, provides a variety of ways to work with numeric data types. One common scenario developers encounter is the need to convert an Integer
object to a primitive int
data type.
In this article, we will explore different methods to achieve this conversion in Java.
Use Auto-Unboxing to Convert Integer
to int
in Java
Before diving into the conversion methods, it’s essential to understand the distinction between Integer
and int
in Java.
Integer
is a wrapper class that encapsulates an int value, providing additional methods and functionality. On the other hand, int
is a primitive data type representing a 32-bit signed integer.
In Java, we can convert an Integer
object to a primitive int
type using auto-unboxing. It is a feature introduced in Java 5 that allows the automatic conversion of an Integer
object to its corresponding primitive int
type.
When an Integer
object is assigned to an int
variable, Java automatically extracts the primitive value from the object, making the conversion seamless. This process enhances code readability and simplifies the syntax for converting between wrapper classes and primitive types.
Let’s consider a simple Java program that demonstrates the conversion of an Integer
object to an int
primitive using auto-unboxing:
public class AutoUnboxingExample {
public static void main(String[] args) {
Integer integerValue = 42;
int intValue = integerValue;
System.out.println("Integer value: " + integerValue);
System.out.println("int value: " + intValue);
}
}
In this example, we begin by creating an Integer
object named integerValue
with the value 42
. The auto-unboxing process takes place when we assign the Integer
object to an int
variable (intValue
).
Instead of explicitly calling a method or using casting, the conversion occurs automatically during the assignment. The program then prints the original Integer
value and the resulting int
value.
When the above code is executed, the output will be as follows:
Integer value: 42
int value: 42
Here, you can observe that the auto-unboxing successfully converted the Integer
object to its primitive int
counterpart, resulting in the same numerical value being displayed for both the Integer
and int
variables.
Use the intValue()
Method to Convert Integer
to int
in Java 1.4 or Lower
While Java’s auto-unboxing simplifies the process of converting an Integer
object to a primitive int
type, sometimes explicit methods are needed. If you’re using the Java 1.4 version or a lower one, then you can use the intValue()
method for this purpose.
The intValue()
method is a member of the Integer
class and is designed specifically for converting an Integer
object to its corresponding primitive int
type.
The syntax is straightforward:
int intValue = integerValue.intValue();
Here, integerValue
is the Integer
object that we want to convert, and intValue
is the resulting primitive int
variable.
Let’s explore a Java program that demonstrates how to use the intValue()
method to convert an Integer
object into an int
primitive:
public class IntValueExample {
public static void main(String[] args) {
Integer integerValue = new Integer(55);
int intValue = integerValue.intValue();
System.out.println("Integer value: " + integerValue);
System.out.println("int value: " + intValue);
}
}
In this example, we begin by defining an Integer
object named integerValue
with the value 55
. To convert this Integer
object to an int
primitive, we use the intValue()
method.
The method is called on the integerValue
object, and its result is assigned to the intValue
variable. The program then prints both the original Integer
value and the resulting int
value.
Unlike auto-unboxing, the conversion here is explicit and involves calling a method designed for this purpose.
Output:
Integer value: 55
int value: 55
While auto-unboxing is more concise, using the intValue()
method provides an explicit and controlled approach to converting Integer
objects to primitive int
types in Java.
Use the Ternary Operator With the intValue()
Method for Safe Conversion of Integer
to int
in Java
In Java, when dealing with an Integer
object, it’s essential to consider the possibility of a null
value. To perform a safe conversion from Integer
to int
and avoid potential runtime errors or exceptions, we can use a conditional approach.
Given that an Integer
object can potentially be null
, a safe conversion involves checking for null
before performing the conversion. This can be achieved using conditional operators to assign a default value in case the Integer
object is null
.
Let’s delve into a Java program that demonstrates safe conversion from Integer
to int
:
public class SafeConversionExample {
public static void main(String[] args) {
Integer integerValue = null;
int intValue = (integerValue != null) ? integerValue.intValue() : 0;
System.out.println("Integer value: " + integerValue);
System.out.println("Safe int value: " + intValue);
}
}
In this example, we start with an Integer
object named integerValue
, intentionally set to null
to demonstrate the safe conversion process. To convert this potentially null
object to an int
primitive, we use a ternary operator.
The condition checks if the integerValue
is not null
. If it is not null
, the intValue()
method is called to perform the conversion; otherwise, the default value of 0
is assigned to the intValue
variable.
The program then prints both the original Integer
value (which may be null
) and the resulting int
value, ensuring a safe conversion even in the presence of null
values.
Output:
Integer value: null
Safe int value: 0
Here, you can observe that the safe conversion successfully handled the null
value by assigning the default value of 0
to the int
variable. This approach ensures the code’s robustness, preventing potential NullPointerExceptions
in scenarios where the Integer
object might be null
.
Use the parseInt()
Method to Convert Integer
to int
in Java
Another method for converting an Integer
object to a primitive int
type involves using the parseInt()
method provided by the Integer
class. This method is particularly useful when dealing with Integer
objects representing numeric strings.
The parseInt()
method is a static method of the Integer
class, and its primary purpose is to convert a string representation of an integer into an int
primitive.
Its syntax is as follows:
int intValue = Integer.parseInt(integerValue.toString());
Here, integerValue
is the Integer
object that we want to convert, and the toString()
method is used to obtain its string representation.
Let’s take a look at a Java program that demonstrates the use of the parseInt()
method for converting an Integer
object to an int
.
public class ParseIntExample {
public static void main(String[] args) {
Integer integerValue = new Integer("42");
int intValue = Integer.parseInt(integerValue.toString());
System.out.println("Integer value: " + integerValue);
System.out.println("int value: " + intValue);
}
}
In this example, we initiate an Integer
object named integerValue
representing the numeric string 42
. To convert this Integer
object to an int
primitive, we utilize the parseInt()
method.
The toString()
method is employed to obtain the string representation of the integerValue
, and parseInt()
processes this string to provide the desired int
result.
The program then prints both the original Integer
value and the resulting int
value, showcasing the use of parseInt()
in the conversion process.
Output:
Integer value: 42
int value: 42
Here, you can observe that the parseInt()
method successfully converted the Integer
object to its primitive int
equivalent. This method is particularly beneficial when working with Integer
objects that hold numeric string values.
Use the valueOf()
Method and Auto-Unboxing to Convert Integer
to int
in Java
The combination of the valueOf()
method and auto-unboxing provides a concise and effective way to convert an Integer
object to a primitive int
type.
The valueOf()
method in the Integer
class is used to create an Integer
object representing a specific int
value. When assigned to an int
variable, auto-unboxing extracts the primitive value automatically, achieving the conversion.
The syntax is as follows:
Integer integerValue = Integer.valueOf(42);
int intValue = integerValue; // Auto-unboxing
Here, 42
is the int
value we want to convert, and integerValue
is the resulting Integer
object.
Take a look at an example Java program that uses the valueOf()
method and auto-unboxing to convert an Integer
object to an int
.
public class ValueOfAutoUnboxingExample {
public static void main(String[] args) {
Integer integerValue = Integer.valueOf(42);
int intValue = integerValue; // Auto-unboxing
System.out.println("Integer value: " + integerValue);
System.out.println("int value: " + intValue);
}
}
In this example, we directly employ the valueOf()
method to create an Integer
object integerValue
representing the int
value 42
. The subsequent assignment to the int
variable intValue
utilizes auto-unboxing, extracting the primitive value from the Integer
object.
The program then prints both the original Integer
value and the resulting int
value, showcasing the efficiency of the valueOf()
method combined with auto-unboxing.
Output:
Integer value: 42
int value: 42
Use Casting to Convert Integer
to int
in Java
While Java provides convenient methods for converting Integer
objects to primitive int
types, it’s worth noting that direct casting is an option. However, this method is not recommended, especially when there’s a risk of encountering null
values.
Casting involves explicitly specifying the type of conversion and can be employed to convert an Integer
object to an int
primitive. The syntax is as follows:
Integer integerValue = new Integer(42);
int intValue = (int) integerValue;
Here, integerValue
is the Integer
object, and (int)
indicates the casting operation.
Let’s see an example demonstrating the use of casting for converting an Integer
object to an int
. Note that this method is not recommended when there’s a risk of encountering null
values:
public class CastingExample {
public static void main(String[] args) {
Integer integerValue = new Integer(42);
int intValue = (int) integerValue;
System.out.println("Integer value: " + integerValue);
System.out.println("int value: " + intValue);
}
}
In this example, we create an Integer
object named integerValue
with the value 42
. The subsequent use of casting, (int) integerValue
, attempts to convert the Integer
object to an int
primitive.
The program then prints both the original Integer
value and the resulting int
value, illustrating the use of casting for conversion.
Output:
Integer value: 42
int value: 42
In this output, you can observe that the casting operation successfully converted the Integer
object to its primitive int
equivalent. However, it’s crucial to emphasize that casting introduces a risk, especially when dealing with null
values.
Using methods like auto-unboxing or intValue()
is generally safer and more idiomatic in Java.
Use Objects.requireNonNull()
With Auto-Unboxing or intValue()
to Convert Integer
to int
in Java
In situations where handling potential null
values is necessary during the conversion of Integer
objects to primitive int
types, using Objects.requireNonNull()
can provide a robust solution. This method is part of the java.util
package and can be employed to check for null
values before performing the conversion.
When combined with auto-unboxing or the intValue()
method, this approach ensures a safe and robust conversion. The syntax for auto-unboxing is as follows:
Integer integerValue = /* ... */;
int intValue = Objects.requireNonNull(integerValue);
And for intValue()
:
Integer integerValue = /* ... */;
int intValue = Objects.requireNonNull(integerValue).intValue();
Here, Objects.requireNonNull()
checks for null
, and if the value is non-null, auto-unboxing or the intValue()
method is applied.
Here’s a Java program demonstrating the use of Objects.requireNonNull()
with auto-unboxing for converting an Integer
object to an int
:
import java.util.Objects;
public class RequireNonNullAutoUnboxingExample {
public static void main(String[] args) {
Integer integerValue = 42;
int intValue = Objects.requireNonNull(integerValue);
System.out.println("Integer value: " + integerValue);
System.out.println("int value: " + intValue);
}
}
In this example, we create an Integer
object named integerValue
with the value 42
. To ensure a robust conversion and handle the possibility of a null
value, we utilize Objects.requireNonNull()
with auto-unboxing.
The method checks whether the integerValue
is non-null, and if so, the auto-unboxing operation extracts the primitive int
value. The program then prints both the original Integer
value and the resulting int
value, illustrating a secure conversion process.
Output:
Integer value: 42
int value: 42
Conclusion
We have seen the diverse set of methods for converting Integer
to int
, each catering to specific needs. Auto-unboxing stands out for its simplicity and readability, while explicit methods like intValue()
and parseInt()
provide control in different scenarios.
Safe conversion and handling of potential null
values are addressed through methods like the ternary operator and Objects.requireNonNull()
. Although direct casting is concise, it carries risks, making it less recommended.
You can choose the most suitable method based on code requirements, readability, and safety, ensuring a flexible and effective approach to Integer
to int
conversions.
Related Article - Java Integer
- How to Handle Integer Overflow and Underflow in Java
- How to Reverse an Integer in Java
- How to Convert Int to Binary in Java
- The Max Value of an Integer in Java
- Minimum and Maximum Value of Integer in Java