Minimum and Maximum Value of Integer in Java
- Understanding Integer Limits in Java
- Using Java to Find Integer Limits
- Practical Applications of Integer Limits
- Conclusion
- FAQ
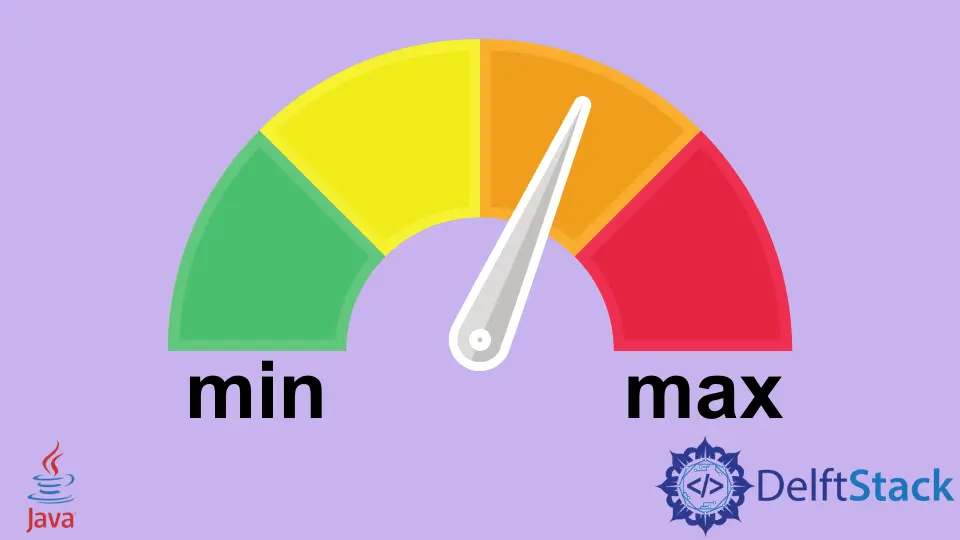
Understanding the minimum and maximum values of integers in Java is crucial for developers, especially when dealing with numerical data. Java provides various data types, but the int
type is one of the most commonly used for storing whole numbers. Knowing the boundaries of these values can help prevent errors and optimize performance in your applications.
In this article, we’ll explore how to find the minimum and maximum values of integers in Java, along with practical examples and explanations. Whether you’re a beginner or an experienced programmer, this guide will enhance your understanding of integer limits in Java.
Understanding Integer Limits in Java
In Java, the int
data type is a 32-bit signed integer. This means it can represent a wide range of values, but it also has its limits. The minimum value an int
can hold is -2,147,483,648, and the maximum is 2,147,483,647. These limits are defined by the constants Integer.MIN_VALUE
and Integer.MAX_VALUE
, which are part of the Integer
class in the Java standard library.
To retrieve these values, you can simply access these constants directly in your code. Here’s a quick example:
public class IntegerLimits {
public static void main(String[] args) {
System.out.println("Minimum Integer Value: " + Integer.MIN_VALUE);
System.out.println("Maximum Integer Value: " + Integer.MAX_VALUE);
}
}
Output:
Minimum Integer Value: -2147483648
Maximum Integer Value: 2147483647
In this code, we are using the Integer.MIN_VALUE
and Integer.MAX_VALUE
constants to print out the minimum and maximum values of integers in Java. This is a straightforward way to access these values without needing to perform any calculations.
Using Java to Find Integer Limits
Another way to find the minimum and maximum values of integers in Java is through arithmetic operations. By creating a simple program that increments and decrements a variable, you can demonstrate how the limits are reached. Here’s an example:
public class IntegerLimitsArithmetic {
public static void main(String[] args) {
int minValue = 0;
int maxValue = 0;
while (minValue > Integer.MIN_VALUE) {
minValue--;
}
while (maxValue < Integer.MAX_VALUE) {
maxValue++;
}
System.out.println("Minimum Integer Value: " + minValue);
System.out.println("Maximum Integer Value: " + maxValue);
}
}
Output:
Minimum Integer Value: -2147483648
Maximum Integer Value: 2147483647
In this example, two while
loops are used to decrement and increment the values until they reach the defined limits. This approach illustrates how the int
type behaves when it approaches its boundaries. However, it is worth noting that this method is not the most efficient way to determine the limits, as it involves unnecessary iterations.
Practical Applications of Integer Limits
Understanding the minimum and maximum values of integers in Java can significantly impact application performance and reliability. For instance, when performing calculations, you must ensure that your results do not exceed these limits. If they do, it can lead to overflow errors, resulting in unexpected behavior or even application crashes.
Moreover, when working with databases or APIs, knowing these limits can help you validate data before processing it. For example, if you expect an integer input from a user, you can check if the input falls within the defined range before proceeding with any calculations. This validation step can save you from potential runtime exceptions and ensure a smoother user experience.
Here’s a simple validation example:
import java.util.Scanner;
public class IntegerValidation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
int userInput = scanner.nextInt();
if (userInput < Integer.MIN_VALUE || userInput > Integer.MAX_VALUE) {
System.out.println("Input is out of range.");
} else {
System.out.println("Input is within range.");
}
}
}
Output:
Enter an integer: 100
Input is within range.
In this example, the program prompts the user to enter an integer and checks if it falls within the acceptable range. This kind of validation is crucial in ensuring that your applications handle user input gracefully.
Conclusion
In conclusion, understanding the minimum and maximum values of integers in Java is essential for any developer. By utilizing the built-in constants Integer.MIN_VALUE
and Integer.MAX_VALUE
, you can easily access these limits without performing complex calculations. Additionally, implementing validation checks can help prevent errors and improve the overall user experience. Whether you’re building simple applications or complex systems, keeping integer limits in mind will help you write more robust and efficient code.
FAQ
-
What is the range of values for an integer in Java?
The range of values for an integer in Java is from -2,147,483,648 to 2,147,483,647. -
How can I find the minimum and maximum values of an integer in Java?
You can find the minimum and maximum values using the constants Integer.MIN_VALUE and Integer.MAX_VALUE. -
What will happen if I exceed the maximum integer value in Java?
If you exceed the maximum integer value, it will cause an overflow, leading to unexpected results or negative numbers. -
Can I use other data types for larger numbers in Java?
Yes, you can use long, BigInteger, or other data types to handle larger numbers in Java. -
Why is it important to validate integer input in Java?
Validating integer input helps prevent runtime exceptions and ensures that your application handles user data correctly.