The Max Value of an Integer in Java
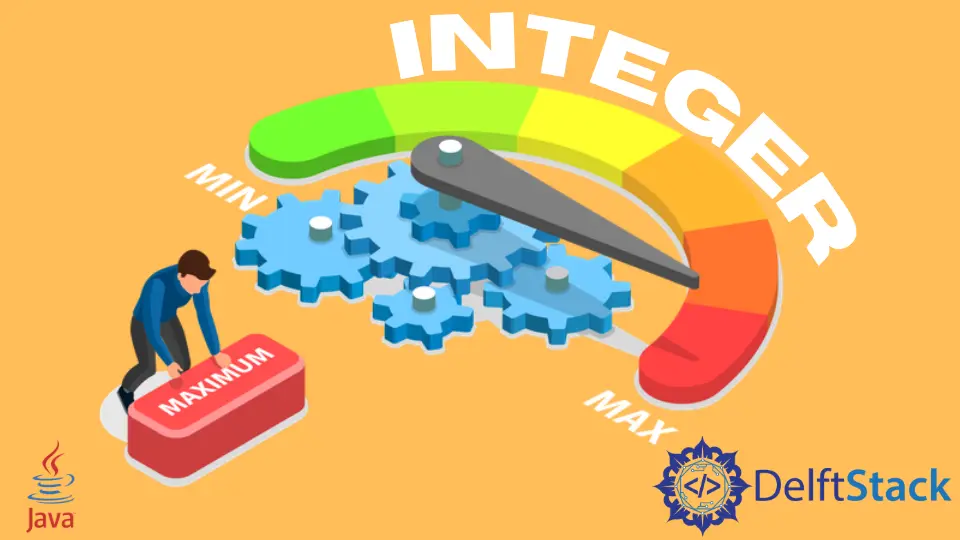
This tutorial introduces the maximum value of an integer in Java and how to get it.
In Java, int
is considered a primitive data type used to store numeric values and takes 4 bytes to store data into memory. Java supports signed values, so the int
range lies between negative and positive values.
See the below table.
Integer Range in Java
Integer | Value |
---|---|
Min | -2147483648 |
Max | 2147483647 |
int
Data Type in Java
We can store any positive and negative integer values in Java, but the value should lie in between its range. See a simple example below.
public class SimpleTesting {
public static void main(String[] args) {
int a = 230;
System.out.println("Positive integer value " + a);
int b = -3423;
System.out.println("Negative integer value " + b);
}
}
Output:
Positive integer value 230
Negative integer value -3423
Max Value of int
in Java
To determine the max value of an integer variable hold, use the MAX_VALUE
constant.
Java Integer
wrapper class provides two constants, MAX_VALUE
and MIN_VALUE
, to get max and min values. It is an easy way to know the integer max value in Java.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int a = 230;
System.out.println("Positive integer value " + a);
int b = ((Integer) a).MAX_VALUE;
System.out.println("Max integer value " + b);
}
}
Output:
Positive integer value 230
Max integer value 2147483647
Java is a strict language and does not allow storing any value outside the range (2147483647). Here, we tried to store a value larger than the max value and see the Java compiler throws a compile error and stops program execution.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int a = 2147483648;
System.out.println("Max integer value+1 " + a);
}
}
Output:
The literal 2147483648 of type int is out of range