How to Convert Int to Double in Java
-
int
in Java -
double
in Java - Implicitly Convert Int to Double Without Utilizing Typecasting in Java
- Implicitly Convert Int to Double by Utilizing Typecasting in Java
- Convert Int to Double Using the Double Wrapper Class in Java
- Conversion Reliant on Java’s Automatic Type Recognition
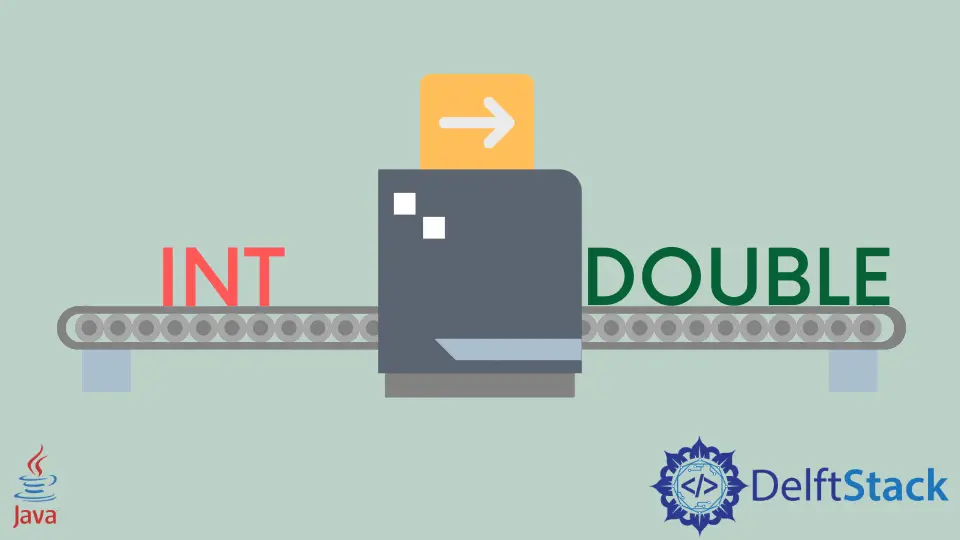
The difference between int and double is that double is used to store 64-bit double-precision floating-point value, and int
is used in the storage of a 32-bit integer.
int
in Java
int
is a primitive data type that is allocated 4 bytes of memory by most systems. It is used to store integer values, and its operations are faster than those of doubles. See the code below.
public class intOperation {
public static void main(String[] args) {
int a = 20;
int b = 3;
int c = a + b;
System.out.println(c);
}
}
Output:
25
In the above code, a
and b
are variables of type int
that store 20 and 3, respectively. The sum of the two int variables results in another int variable 23.
double
in Java
double
is a primitive data type that is allocated 8 bytes of memory by most systems. It is used to store floating-point values (values that are either a fraction or end with a fraction). The arithmetic computation of doubles by the system is slower than that of int. See the code example below.
public class doubleOperation {
public static void main(String[] args) {
double a = 20.5;
double b = 5.0;
double c = a + b;
System.out.println(c);
}
}
Output:
25.5
In the above code, a
and b
are variables of type double that store 20.5 and 5.0, respectively. The sum of the two double variables results in another double variable 25.5.
Implicitly Convert Int to Double Without Utilizing Typecasting in Java
The implicit conversion of int to double relies on the fact that double data types have a bigger memory size and a wider range. See the code below:
public class intToDouble {
public static void main(String args[]) {
// the int value
int a = 55;
// conversion of int to double
double b = a;
System.out.println(b);
}
}
Output:
55.0
Implicitly Convert Int to Double by Utilizing Typecasting in Java
Like we have done in the previous method, we use an assignment operator, but we cast it to type double. See the code below:
public class intToDouble {
public static void main(String args[]) {
// the int value
int a = 55;
// conversion of int to double
double b = (double) a;
System.out.println(b);
}
}
Output:
55
Convert Int to Double Using the Double Wrapper Class in Java
In this method, we use the double
wrapper class’ valueOf()
method. This method is direct and easy to implement repeatedly. It takes the int
as a parameter and returns a double
value. See the code below.
public class intToDouble {
public static void main(String args[]) {
// the int value
int a = 55;
// conversion of int to double
double b = Double.valueOf(a);
System.out.println(b);
}
}
Output:
55.0
Conversion Reliant on Java’s Automatic Type Recognition
This is a direct method where we multiply the int
variable with a double
value to get the result as a double. See the code below.
public class intToDouble {
public static void main(String args[]) {
// the int value
int a = 55;
// conversion of int to double
double b = 1.0 * a;
System.out.println(b);
}
}
Output:
55.0
Related Article - Java Int
- How to Convert Int to Char in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java
- How to Convert Int to Byte in Java