Float and Double Data Type in Java
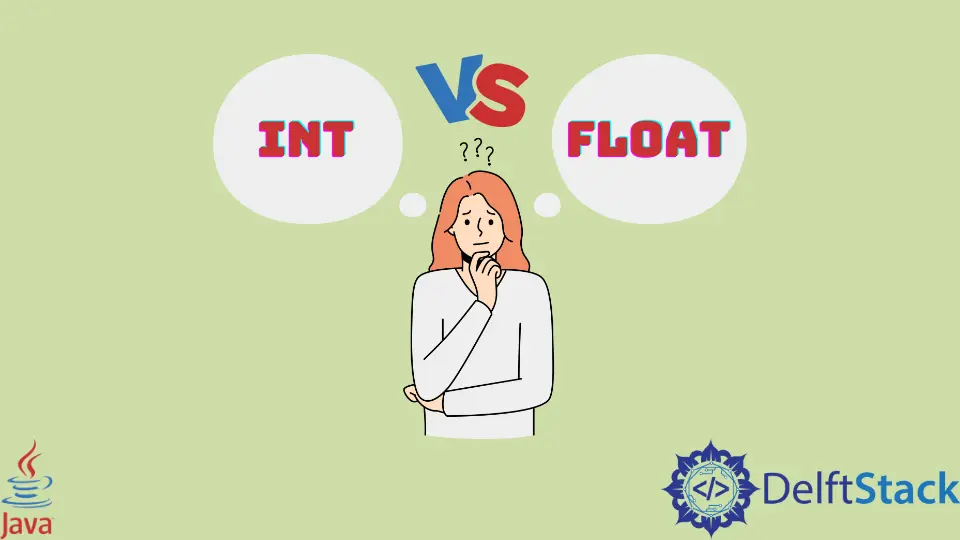
Float and double data types are used to store real or floating-point numbers in Java, but a double data type is more precise than float. double
is the default data type for floating-point numbers.
To Store Floating-Point Numbers With Precision
A float
is a 32 bit IEEE 754 floating-point while a double is a 64 bit IEEE 754 floating-point. Float
has a lower range when compared to double.
In the example given below, we have d
of double
data type, which is obtained by dividing two double
variables d1
and d2
. Similarly, we have f1
resulted when two float
variables f1
and f2
are divided. In the case of double
, there is no need to use the suffix d
or D
, whereas for float
type data we need to use the suffix f
or F
as by default, all real numbers are considered as double
in Java.
In the output, we can see that the precision and accuracy of the double
variable d
is more than the float
variable f
. We know double
is a bigger data type than float
so we need to down-cast it. To typecast double
to float
we need to mention the float
keyword in brackets before the decimal value.
It is visible in the output that by converting the double
variable to float
it loses data and precision as we are storing a larger data type into a smaller data type. Hence double
takes more memory to store double-precision floating numbers and gives accurate results.
public class Test {
public static void main(String args[]) {
double d1 = 10.0;
double d2 = 3.0;
double d = d1 / d2;
System.out.println("double d : " + d);
float f1 = 10.0f;
float f2 = 3.0f;
float f = f1 / f2;
System.out.println("float f : " + f);
float f3 = (float) d;
System.out.println("float f3 : " + f3);
double d3 = f;
System.out.println("double d3 : " + d3);
}
}
Output:
double d : 3.3333333333333335
float f : 3.3333333
float f3 : 3.3333333
double d3 : 3.3333332538604736
The difference between the two data types can be illustrated with the given tabular data.
DataType | Precision | Size | Default DataType | Default Value | Suffix | Wrapper Class | Loss Of Data | Keyword |
---|---|---|---|---|---|---|---|---|
Float | Single Precision (6-7 decimal digits) | 32 bit | No | 0.0f | Use f or F. We need to add this suffix as by default float numbers explicitly are treated as double | java.lang.Float | No data loss when converting float to double | The keyword float is used for float type |
Double | Double Precision (15-16 decimal digits) | 64 bit | Yes | 0.0d | Use d or D. Optional to use suffix | java.lang.Double | Data loss when converting double to float | The keyword double is used to define a double-precision number |
Float and double are used to represent real numbers. Both data types are not precise; they are approximate types. When we need an accurate and precise result, we should go for double. If there is any memory and space constraint, then float should be considered.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Double
- How to Convert Int to Double in Java
- Double in Java
- How to Compare Doubles in Java
- How to Convert Double to String in Java
- How to Convert Double to Float in Java