How to Convert Double to String in Java
- Convert Double to String in Java
-
Convert Double to String by Using the
toString()
Method in Java -
Convert Double to String by Using the
format()
Method in Java -
Convert Double to String by Using the
Decimalformat
Class in Java -
Convert Double to String by Using the
+
Operator in Java
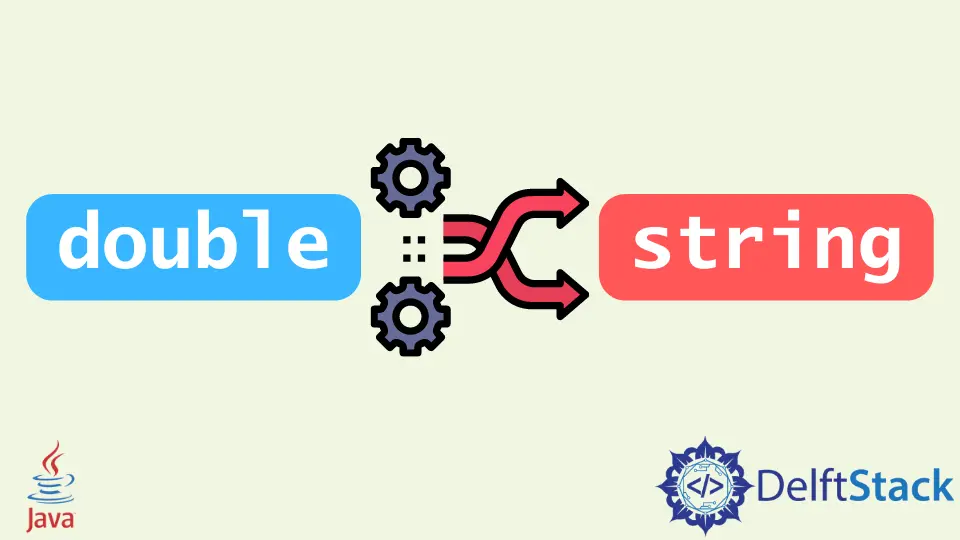
This tutorial introduces how to convert a double type into a string object in Java. We’ve also listed some example codes to help you understand this topic better.
The term double is a data type in Java used to store real numbers or floating-point numbers. On the other hand, a string is a class that holds a sequence of characters enclosed in double quotes.
This article teaches you ways on how to convert a double object to a string object by using some built-in methods such as valueOf()
, toString()
, and valueOf()
. Let’s get started!
Convert Double to String in Java
This first method is one of the simple examples where we have a variable price of a double type that holds a floating value. Using the valueOf()
method of the string, we convert this value to a string
object. See the example below.
public class Main {
public static void main(String[] args) {
double price = 44.22;
String val = String.valueOf(price);
System.out.println(val);
}
}
Output:
44.22
Convert Double to String by Using the toString()
Method in Java
The Java Double
wrapper class provides a toString()
method that takes a double type argument and returns a string object. We can use this method to convert a double type to a string object in Java. See the example below.
public class Main {
public static void main(String[] args) {
double price = 44.22;
String val = Double.toString(price);
System.out.println(val);
}
}
Output:
44.22
Convert Double to String by Using the format()
Method in Java
Java features the format()
method that belongs to the String
class and is used to format string objects; we can use this process to convert a double value to a string object.
It takes two arguments: the first is a value format, or you can better say format specifier, and the second is a value that we want to get converted. See the example below.
public class Main {
public static void main(String[] args) {
double price = 44.22;
String val = String.format("%.2f", price);
System.out.println(val);
}
}
Output:
44.22
Convert Double to String by Using the Decimalformat
Class in Java
The Java DecimalFormat
class is used to format decimal data. We will use the format()
method of this class to convert a double data type into a string in Java. See the example below.
import java.text.DecimalFormat;
public class Main {
public static void main(String[] args) {
double price = 44.22;
DecimalFormat decimalFormat = new DecimalFormat("#,##0.00");
String val = decimalFormat.format(price);
System.out.println(val);
}
}
Output:
44.22
Convert Double to String by Using the +
Operator in Java
This is one of the simplest way to convert any data type into string in Java. Here, if we concatenate any value to the string, the result will be a string type. So, we will use the same concept here to convert a double type into a string in Java. See the example below.
public class Main {
public static void main(String[] args) {
double price = 44.22;
String val = price + "";
System.out.println(val);
}
}
Output:
44.22
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java