Double in Java
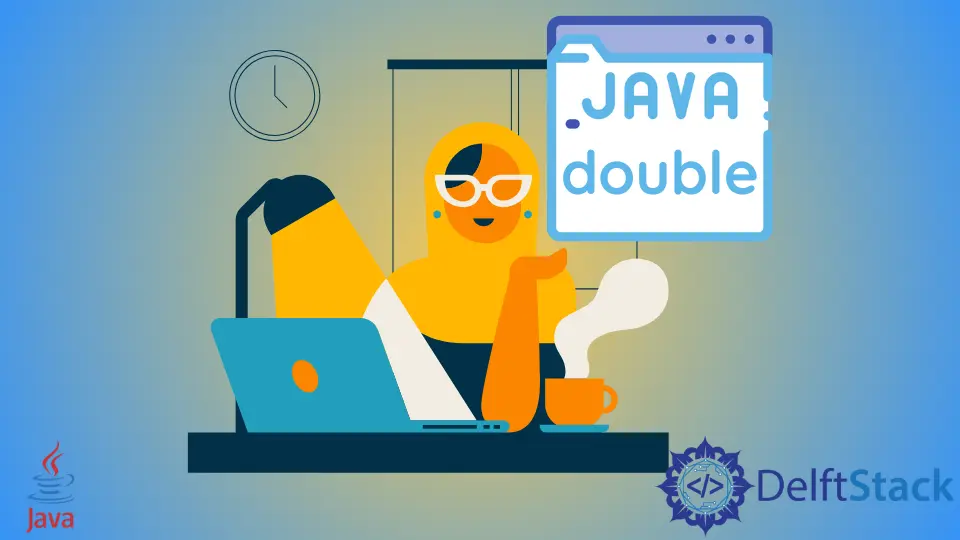
This tutorial introduces the double and its uses in Java.
Double is a data type in Java used to store floating-point values. Java provides a rich set of data types such as int, float, double, boolean, etc. The int data type stores an integer value like -1
, 2
, -3
, and 4
.
The int data type can store values between -2,147,483,648 (-231) to 2,147,483,647 (231-1). It cannot store fractional values such as 1.5
and 2.88
. The second primitive argument called float can store fractional values.
Java has another primitive data type to store fractional values called double. This tutorial will discuss double and the difference between double and Double.
Double is a primitive data type in Java, whereas Double
is a wrapper class that can create double object value. Let’s understand with some examples.
Double VS Float Type in Java
- The double has a bigger range than that of float type in Java.
- It is because double takes 8 bytes, whereas float only uses 4 bytes.
- The double data type is slower than that involving float data type.
- The double data type has greater precision than the float data type.
- The double can precision of about 15 digits, whereas float can have a precision of only six to seven digits.
Primitive Double Type in Java
In this example, we created a variable d of the double type that holds a floating-point value.
See, this code works fine due to double type. We cannot store such values using the int type. This is how we can declare double type in Java.
See the example below.
public class SimpleTesting {
public static void main(String args[]) {
double d = 34354.232;
System.out.println("d = " + d);
}
}
Output:
d = 34354.232
Adding Two Double Values in Java
Let’s now calculate the sum of two double values.
For this, we created three double variables and held the result into a double variable. If we store the result into int type, the result will be truncated due to limited memory.
Look at the code below.
public class SimpleTesting {
public static void main(String args[]) {
double d1 = 34354.232;
double d2 = 4333.32313;
double sum = d1 + d2;
System.out.println("sum = " + sum);
}
}
Output:
sum = 38687.55513
Double Wrapper Class in Java
Just like int has Integer, the float has Float
in the same way double has the Double
class to create objects of primitive double values. In other words, Double
is the wrapper class of the double data type.
The syntax of the Double class is:
public final class Double extends Number implements Comparable<Double>
Wrapping the double data type into the Double wrapper class allows us to use various in-built methods. Let’s understand with code example.
Creating Double Object in Java
Let’s first create an example to create a Double
object. Look at the code below.
public class SimpleTesting {
public static void main(String args[]) {
Double d1 = 34354.232;
System.out.println(d1);
}
}
Output:
34354.232
Adding Two Double Values Using the sum()
Method in Java
Java Double class provides several built-in methods to perform on floating values. Java added a sum()
method to Java 8 version to get an addition of two floating values.
Here, we used this method and saw the code below.
public class SimpleTesting {
public static void main(String args[]) {
Double d1 = 34354.232;
Double d2 = 4333.32313;
Double sum = Double.sum(d1, d2);
System.out.println(sum);
}
}
Output:
38687.55513
Conclusion
In this tutorial, we saw what double means in Java. We then learned the difference between double and float. We also learned about the Double wrapper class and its methods.