How to Convert Double to Float in Java
-
Use Direct Casting to Convert
double
tofloat
in Java -
Use the
Double.floatValue()
Method to Convertdouble
tofloat
in Java -
Use the
float
Wrapper Class to Convertdouble
toFloat
in Java - Conclusion
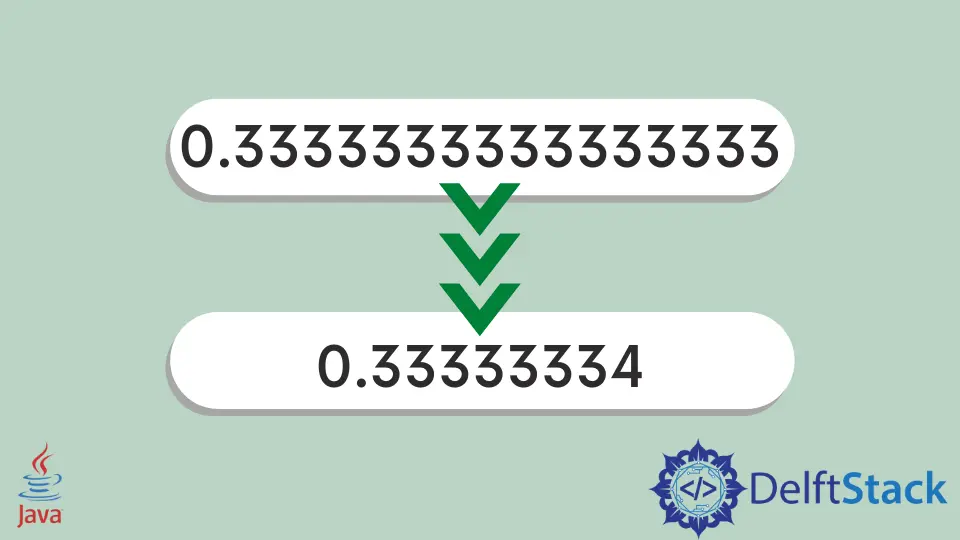
Java provides several methods for converting a double
value to a float
. This process is necessary when you need to work with data that requires a smaller memory footprint or when interfacing with APIs that expect float
values.
However, it’s important to understand that converting from double
to float
may result in a loss of precision due to the inherent differences in their representations.
Let’s explore various methods for converting a double
to a float
in Java.
Use Direct Casting to Convert double
to float
in Java
Converting a double
value to a float
in Java can be achieved using direct casting. This method involves explicitly specifying the target type in parentheses, allowing you to perform the conversion concisely.
To further elaborate, direct casting is a straightforward process where you inform the compiler to treat a variable of one type as if it were another type. In the context of converting a double
to a float
, the (float)
syntax indicates to Java that you want to treat the double
value as a float
.
This method is quick and easy but comes with a caveat – potential loss of precision due to the smaller representation range and fewer significant digits of the float
type.
Here’s an example code to see how this works:
public class DoubleToFloatExample {
public static void main(String[] args) {
double doubleValue = 1.0 / 3.0;
float floatValue = (float) doubleValue;
System.out.println("Original double value: " + doubleValue);
System.out.println("Converted float value: " + floatValue);
}
}
Output:
Original double value: 0.3333333333333333
Converted float value: 0.33333334
In this example, we start by declaring a double
variable named doubleValue
and assigning it the value 1.0 / 3.0
.
To convert this double
value to a float
using direct casting, we employ the (float)
syntax before the doubleValue
variable. This informs the compiler to treat doubleValue
as a float
, allowing us to store the result in a float
variable named floatValue
.
The two print statements at the end of the program display the original double
value and the converted float
value. The output will demonstrate the conversion process in action.
It’s essential to note that while direct casting is a convenient way to convert between numeric types, developers should exercise caution, especially when working with types that have differing precision. The potential loss of precision should be considered based on the specific requirements of the application.
Always test and validate your code thoroughly to ensure that the conversion meets your expectations.
Use the Double.floatValue()
Method to Convert double
to float
in Java
Another method for converting a double
value to a float
involves using the floatValue()
method provided by the Double
class. This method is particularly useful when dealing with instances of the Double
wrapper class.
The floatValue()
method is a member of the Double
class, which is part of Java’s standard library. When applied to a Double
object, this method extracts and returns the equivalent float
value.
This allows for a seamless conversion from double
to float
in scenarios where the input is an object rather than a primitive data type. It provides a more object-oriented approach to conversion compared to direct casting.
Let’s have an example:
public class DoubleToFloatUsingMethod {
public static void main(String[] args) {
double doubleValue = 1.0 / 3.0;
Double doubleObject = new Double(doubleValue);
float floatValue = doubleObject.floatValue();
System.out.println("Original double value: " + doubleValue);
System.out.println("Converted float value: " + floatValue);
}
}
Output:
Original double value: 0.3333333333333333
Converted float value: 0.33333334
In this example, we begin by declaring a double
variable named doubleValue
with the same value as the previous example. To utilize the floatValue()
method, we create a Double
object named doubleObject
and pass the doubleValue
as an argument to its constructor.
This object-oriented approach allows us to access the floatValue()
method associated with the Double
class.
The line float floatValue = doubleObject.floatValue();
invokes the floatValue()
method on our Double
object, effectively converting the encapsulated double
value to a float
. The converted result is then stored in the float
variable named floatValue
.
Finally, the program concludes with two print statements displaying both the original double
value and the converted float
value.
Use the float
Wrapper Class to Convert double
to Float
in Java
Converting a double
value to a float
can also be accomplished using the Float
wrapper class. This method involves creating a new Float
object and then extracting the float
value.
While this approach is less common compared to direct casting, it provides an alternative when dealing with object-oriented scenarios or when you specifically want to utilize the wrapper class functionality.
The Float
class is part of Java’s standard library and serves as a wrapper for the float
primitive type. It includes various methods, one of which is floatValue()
.
When applied to a Float
object, this method returns the primitive float
value encapsulated by the object. In the context of converting a double
to a float
, this involves creating a new Float
object with the double
value as an argument and then using floatValue()
to obtain the equivalent float
representation.
Here’s a code example:
public class DoubleToFloatUsingWrapperClass {
public static void main(String[] args) {
double doubleValue = 1.0 / 3.0;
Float floatObject = new Float(doubleValue);
float floatValue = floatObject.floatValue();
System.out.println("Original double value: " + doubleValue);
System.out.println("Converted float value: " + floatValue);
}
}
Output:
Original double value: 0.3333333333333333
Converted float value: 0.33333334
In this example, the conversion process involves using the Float
wrapper class. We create a new Float
object named floatObject
by passing the doubleValue
as an argument to its constructor.
Next, we use the floatValue()
method on the floatObject
to extract the primitive float
value. This step completes the conversion from double
to float
, and the result is stored in the float
variable named floatValue
.
The output provides a clear representation of the conversion process using the Float
wrapper class.
Conclusion
Converting a double
value to a float
in Java offers multiple approaches, each tailored to specific scenarios and considerations. Whether through direct casting, the floatValue()
method, or the Float
wrapper class, we must weigh the trade-offs of simplicity against potential precision loss.
Direct casting is concise but may result in reduced accuracy due to the smaller range of float
. The floatValue()
method and Float
wrapper class, while less common, provide an object-oriented alternative and should be chosen based on the specific needs of the application.
Regardless of the method chosen, thorough testing and consideration of precision requirements are imperative to ensure the successful conversion of double
to float
in Java.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Double
- How to Convert Int to Double in Java
- Double in Java
- How to Compare Doubles in Java
- Float and Double Data Type in Java
- How to Convert Double to String in Java