How to Convert Float to String and String to Float in Java
-
Convert String to Float Using the
valueOf()
Method -
Convert String to Float Using the
parseFloat()
Method -
Convert String to Float Using the
Float()
Method -
Convert Float to String Using
toString()
Method -
Convert Float to String Using the
+
Operator -
Convert Float to String Using the
valueOf()
Method -
Convert Float to String Using the
format()
Method
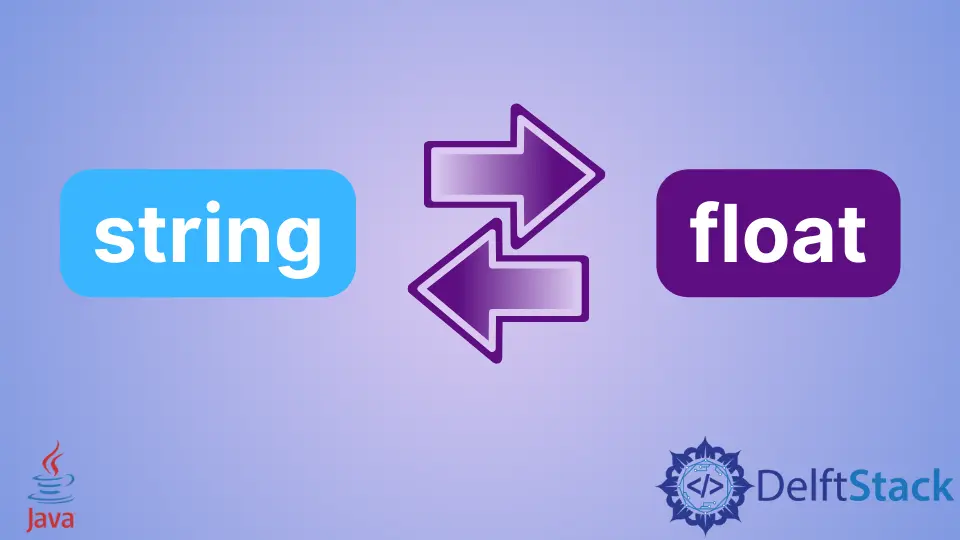
This tutorial introduces how to convert float to string and string to float in Java.
Convert String to Float Using the valueOf()
Method
We can use the valueOf()
method of the Float
class to convert string to float in Java. The valueOf()
method takes an argument and returns a float type value. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
System.out.println("String value: " + str);
float f_Val = Float.valueOf(str);
System.out.println("Float value: " + f_Val);
}
}
Output:
String value: 123
Float value: 123.0
Convert String to Float Using the parseFloat()
Method
The Float
class contains a parseFloat()
method that parses a string type value to float type. It takes a single argument and returns a float value. See the below example.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
System.out.println("String value: " + str);
float f_Val = Float.parseFloat(str);
System.out.println("Float value: " + f_Val);
}
}
Output:
String value: 123
Float value: 123.0
Convert String to Float Using the Float()
Method
In this example, we use the Float()
constructor that takes a string type argument and returns a primitive type float value. We can use this to convert string to float value in Java. See the below example.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
System.out.println("String value: " + str);
float f_Val = new Float(str);
System.out.println("Float value: " + f_Val);
}
}
Output:
String value: 123
Float value: 123.0
Convert Float to String Using toString()
Method
Here, we used toString()
method of the Float
class to get the string type of the float value. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = Float.toString(fVal);
System.out.println("String Value: " + str);
}
}
Output:
Float Value: 23.25
String Value: 23.25
Convert Float to String Using the +
Operator
In Java, plus operator can be used to convert float to string. The plus operator is used to concatenate any type value to the string and returns a string. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = "" + fVal;
System.out.println("String Value: " + str);
}
}
Output:
Float Value: 23.25
String Value: 23.25
Convert Float to String Using the valueOf()
Method
To Convert float to string, we used the valueOf()
method of the String
class that takes a float type argument and returns a string to the caller. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = String.valueOf(fVal);
System.out.println("String Value: " + str);
}
}
Output:
Float Value: 23.25
String Value: 23.25
Convert Float to String Using the format()
Method
This is useful when we want to get a formatted string in the specified format such as two digits after the decimal point. So, we can use the DecimalFormat
class and its format()
method to get a string object. See the example below.
import java.text.DecimalFormat;
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = new DecimalFormat("#.00").format(fVal);
System.out.println("String Value: " + str);
}
}
Output:
Float Value: 23.25
String Value: 23.25
Related Article - Java Float
- Float and Double Data Type in Java
- How to Convert Int to Float in Java
- How to Convert Double to Float in Java
- How to Print a Float With 2 Decimal Places in Java