How to Print a Float With 2 Decimal Places in Java
-
Print Float With 2 Decimal Places Using the
printf()
Method in Java -
Print Float With 2 Decimal Places Using
DecimalFormat
Class in Java -
Print Float With 2 Decimal Places Using
format()
Method in Java -
Print Float With 2 Decimal Places Using
DecimalFormat
Class in Java
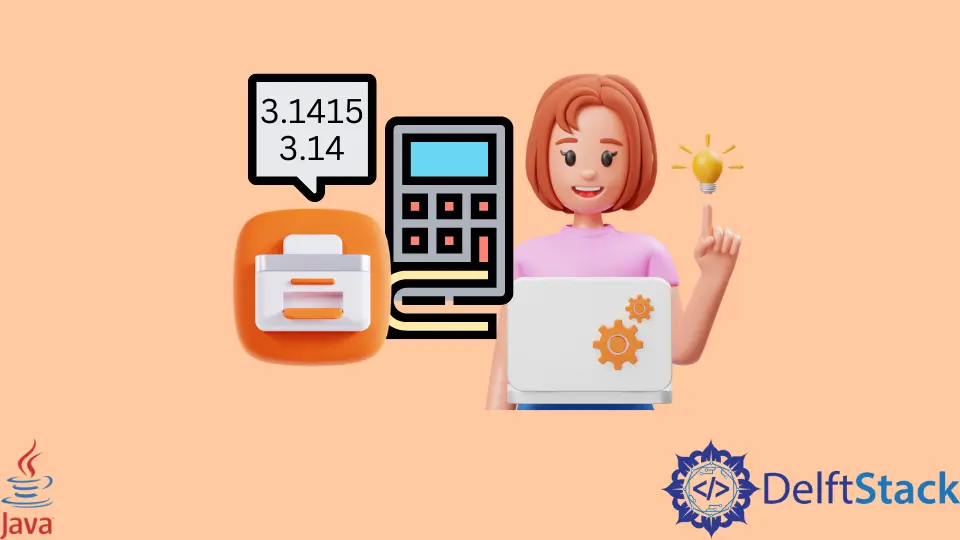
This tutorial introduces how to get float value with 2 decimal places. There are several ways to get float value with 2 decimal places like using the printf()
method, the DecimalFormat
class, the format()
method of String
class, etc. Let’s take a close look at the examples.
Print Float With 2 Decimal Places Using the printf()
Method in Java
The printf()
is a method to print formatted output to the console. It is similar to printf()
in C language. Here, we can specify the limit of digits for the output. "%.2f"
specifies that it has 2 decimal places. See the example below.
public class SimpleTesting {
public static void main(String args[]) {
float Pi = 3.1415f;
System.out.println(Pi);
// Get only 2 decimal points
System.out.printf("%.2f", Pi);
}
}
Output:
3.1415
3.14
Print Float With 2 Decimal Places Using DecimalFormat
Class in Java
The DecimalFormat
is a Java class that provides utility methods to format decimal values in Java. Here, we use the setMaximumFractionDigits()
method to set decimal limits and format()
method to get formatted output.
import java.text.DecimalFormat;
public class SimpleTesting {
public static void main(String args[]) {
float Pi = 3.1415f;
System.out.println(Pi);
DecimalFormat frmt = new DecimalFormat();
frmt.setMaximumFractionDigits(2);
// Get only 2 decimal points
System.out.println(frmt.format(Pi));
}
}
Output:
3.1415
3.14
Print Float With 2 Decimal Places Using format()
Method in Java
Java String
class provides a method format()
to get formatted output, but it returns a String
object rather than a float value. So, we need to convert from string to float to get the final output in the float. See the example below.
public class SimpleTesting {
public static void main(String args[]) {
float Pi = 3.1415f;
System.out.println(Pi);
// Get only 2 decimal points
String str = String.format("%.02f", Pi);
Pi = Float.valueOf(str);
System.out.println(Pi);
}
}
Output:
3.1415
3.14
Print Float With 2 Decimal Places Using DecimalFormat
Class in Java
We can use the constructor of the DecimalFormat
class to specify the limit for decimal places. Here we use #
char to inside the constructor and format()
method to get formatted output.
import java.text.DecimalFormat;
public class SimpleTesting
{
public static void main(String args[])
{
float Pi = 3.1415f;
System.out.println(Pi);
DecimalFormat frmt = new DecimalFormat("#.##");
// Get only 2 decimal points
System.out.println(frmt.format(Pi));
}
}
Output:
3.1415
3.14