Convertir Float en String et String en Float en Java
-
Convertir une chaîne en flottant en utilisant la méthode
valueOf()
-
Convertir une chaîne en flottant en utilisant la méthode
parseFloat()
-
Convertir une chaîne en flottant en utilisant la méthode
Float()
-
Convertir Float en String en utilisant la méthode
toString()
-
Convertir Float en String à l’aide de l’opérateur
+
-
Convertir Float en String en utilisant la méthode
valueOf()
-
Convertir Float en String en utilisant la méthode
format()
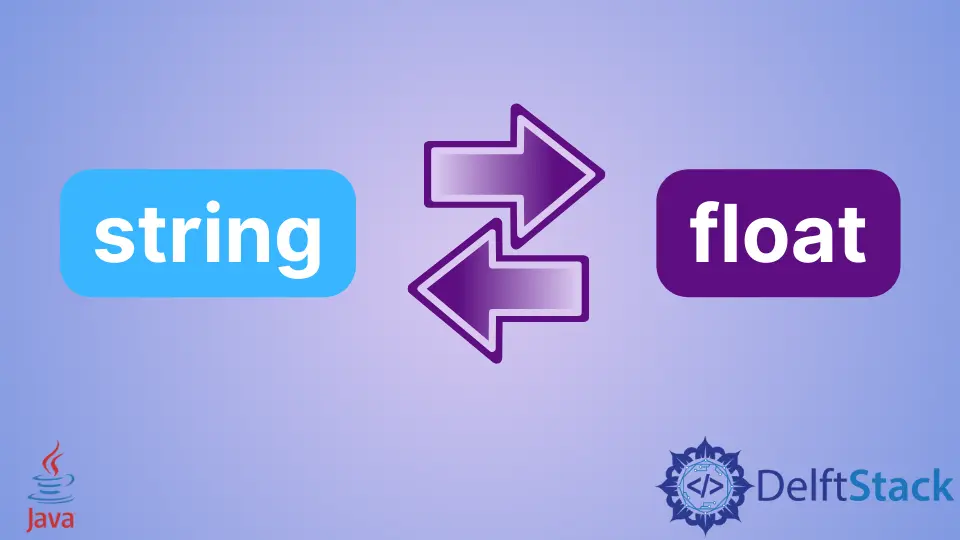
Ce didacticiel explique comment convertir un float en chaîne et une chaîne en float en Java.
Convertir une chaîne en flottant en utilisant la méthode valueOf()
Nous pouvons utiliser la méthode valueOf()
de la classe Float
pour convertir une chaîne en float en Java. La méthode valueOf()
prend un argument et renvoie une valeur de type float. Voir l’exemple ci-dessous.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
System.out.println("String value: " + str);
float f_Val = Float.valueOf(str);
System.out.println("Float value: " + f_Val);
}
}
Production:
String value: 123
Float value: 123.0
Convertir une chaîne en flottant en utilisant la méthode parseFloat()
La classe Float
contient une méthode parseFloat()
qui analyse une valeur de type chaîne en type float. Il prend un seul argument et renvoie une valeur flottante. Voir l’exemple ci-dessous.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
System.out.println("String value: " + str);
float f_Val = Float.parseFloat(str);
System.out.println("Float value: " + f_Val);
}
}
Production:
String value: 123
Float value: 123.0
Convertir une chaîne en flottant en utilisant la méthode Float()
Dans cet exemple, nous utilisons le constructeur Float()
qui prend un argument de type chaîne et renvoie une valeur de type primitif float. Nous pouvons l’utiliser pour convertir une chaîne en valeur flottante en Java. Voir l’exemple ci-dessous.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
System.out.println("String value: " + str);
float f_Val = new Float(str);
System.out.println("Float value: " + f_Val);
}
}
Production:
String value: 123
Float value: 123.0
Convertir Float en String en utilisant la méthode toString()
Ici, nous avons utilisé la méthode toString()
de la classe Float
pour obtenir le type de chaîne de la valeur float. Voir l’exemple ci-dessous.
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = Float.toString(fVal);
System.out.println("String Value: " + str);
}
}
Production:
Float Value: 23.25
String Value: 23.25
Convertir Float en String à l’aide de l’opérateur +
En Java, l’opérateur plus peut être utilisé pour convertir float en chaîne. L’opérateur plus est utilisé pour concaténer toute valeur de type à la chaîne et renvoie une chaîne. Voir l’exemple ci-dessous.
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = "" + fVal;
System.out.println("String Value: " + str);
}
}
Production:
Float Value: 23.25
String Value: 23.25
Convertir Float en String en utilisant la méthode valueOf()
Pour convertir float en string, nous avons utilisé la méthode valueOf()
de la classe String
qui prend un argument de type float et renvoie une chaîne à l’appelant. Voir l’exemple ci-dessous.
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = String.valueOf(fVal);
System.out.println("String Value: " + str);
}
}
Production:
Float Value: 23.25
String Value: 23.25
Convertir Float en String en utilisant la méthode format()
Ceci est utile lorsque nous voulons obtenir une chaîne formatée au format spécifié, par exemple deux chiffres après la virgule décimale. Ainsi, nous pouvons utiliser la classe DecimalFormat
et sa méthode format()
pour obtenir un objet string. Voir l’exemple ci-dessous.
import java.text.DecimalFormat;
public class SimpleTesting {
public static void main(String[] args) {
float fVal = 23.25f;
System.out.println("Float Value: " + fVal);
String str = new DecimalFormat("#.00").format(fVal);
System.out.println("String Value: " + str);
}
}
Production:
Float Value: 23.25
String Value: 23.25
Article connexe - Java Float
- Type de données flottant et double en Java
- Convertir Int en Float en Java
- Convertir Double en Flottant en Java
- Comment imprimer un float avec 2 décimales en Java