How to Fix the Missing Server JVM Error in Java
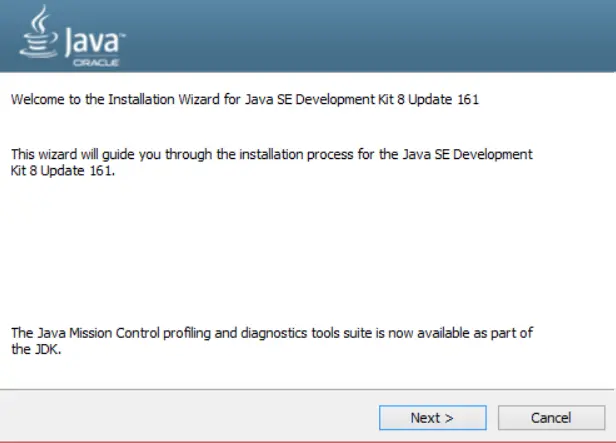
Java Virtual Machine (JVM) is an essential component of the Java ecosystem, providing a runtime environment for executing Java code. The JVM is responsible for loading class files, executing methods, and managing memory.
In a server environment, the JVM is typically installed on the server and is used by Java applications to run.
However, when you try to run a Java application, you may encounter an error that says, Could not find the server JVM
. This error occurs when the JVM cannot be found on the system where the application is being run.
Reasons for the Error
There are several reasons why you may encounter this error, including:
- The JVM is not installed: If the JVM is not installed on the system, the application will not be able to find it and will throw the error.
- The JVM is installed but not in the
PATH
: If the JVM is installed but is not in thePATH
environment variable, the application will not be able to find it and will throw the error. - The JVM version is incorrect: The error may occur if the application uses a JVM version different from the version installed on the system.
- The JVM is damaged or corrupted: If the JVM is damaged or corrupted, the application may not be able to use it, resulting in an error.
Install Java JDK to Fix the Missing Server JVM
Error
One solution to fix the Missing 'server' JVM
error in Java is to install the Java Development Kit (JDK), which includes the 'server' JVM
component. Here are the steps to install Java JDK.
-
Uninstall Java JRE
Before installing Java JDK, uninstall any existing Java Runtime Environment (JRE) on your system. Here’s how:
- Go to the Control Panel and select
Programs and Features
(orAdd or Remove Programs
on older versions of Windows). - Find Java in the list of installed programs and select it.
- Click the
Uninstall
button and follow the prompts to uninstall Java from your system.
- Go to the Control Panel and select
-
Download Java JDK
Once you’ve uninstalled Java JRE, you can download Java JDK from the official Oracle website. Select the appropriate version for your operating system.
-
Install Java JDK
After downloading Java JDK, run the installer and follow the prompts to install it on your system. Select the
Custom
installation option and check the box next toServer JRE
to install the'server' JVM
component. -
Set Environment Variables
Finally, you need to set the
JAVA_HOME
environment variable to point to the location of the Java JDK installation directory. Here’s how:- Right-click on
Computer
(orThis PC
) and selectProperties
. - Click
Advanced system settings
and select theEnvironment Variables
button. - Under
System Variables
, clickNew
and enterJAVA_HOME
as the variable name and the path to the Java JDK installation directory as the variable value. - Click
OK
to save the environment variable.
- Right-click on
Example of the Error
Here’s an example of how Java’s missing server JVM
error can occur.
public class Main {
public static void main(String[] args) {
System.out.println("Starting Java application");
try {
System.out.println(System.getProperty("java.version"));
} catch (Exception e) {
System.out.println("Error: Could not find server JVM");
}
}
}
In this example, the System.getProperty
method is used to retrieve the version of the Java Virtual Machine (JVM) being used. If the JVM is not installed or found, the method will throw an exception, which the catch
block will catch.
In the catch
block, we print the message "Error: Could not find server JVM"
to indicate that the JVM is missing. If the JVM is found and the application can retrieve the version, the version will be printed to the console.
This example demonstrates how a Java application can detect the missing server JVM
error.
Click here to check the working of the above code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack