What Is a Null Pointer Exception in Java
-
What Is
NullPointerException
in Java? -
Causes of the
NullPointerException
in Java -
Avoid the
NullPointerException
in Java -
Handle the
NullPointerException
Class in Java -
Encounters With
NullPointerException
in Command-Line Arguments in Java
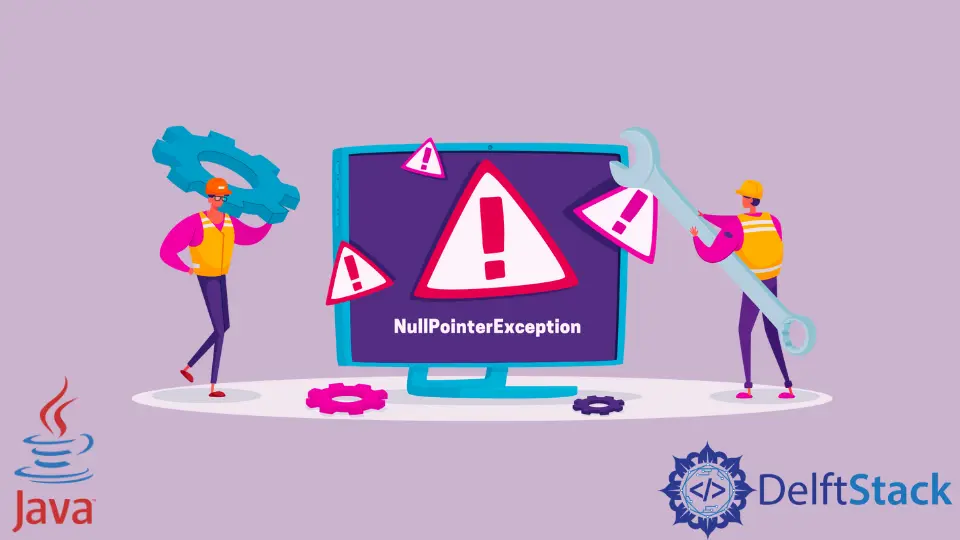
This tutorial introduces the Java null pointer exception and how you can handle it. We’ve included a few sample programs for you to follow.
In Java, the default value of any reference variable is a null value that points to a memory location but does not have any associate value. When a programmer tries to perform any operation with this reference variable, it throws a null pointer exception due to null conditions.
This is a scenario where this exception can occur, but this exception is the most occurred in Java - which breaks the code and leads to abnormal execution flows. The following points can raise NullPointerException
in a Java code:
- Calling a null object’s instance method.
- Modyifying a null object’s field.
- Treating the length of null as if it were an array.
To handle this exception, Java provides a class NullPointerException
located in the java.lang
package. We can use this class in the catch
block to specify the exception type and then catch it to avoid code break.
What Is NullPointerException
in Java?
The most basic example to understand the NullPointerException
class is to create a string
object and then apply a method like toUpperCase()
to convert the string into upper case. Look at the example below and notice that it throws an exception because the string
object is empty:
public class SimpleTesting {
static String str;
public static void main(String[] args) {
String newStr = str.toUpperCase();
System.out.println(newStr);
}
}
Output:
Exception in thread "main" java.lang.NullPointerException
Causes of the NullPointerException
in Java
We understood that the NullPointerException
occurs when an uninitialized (null
) object/variable is accessed.
Let’s see in what scenarios this exception occurs. Some of the most common scenarios are:
- When trying to synchronize on a
null
object, - When passing a
null
argument, - When invoking or calling a function using a
null
object, - When throwing
null
from a function that throws an exception, - When trying to access or modify the element using indices (in the case of an array) for a
null
object.
Example code:
public class Demo {
public static void main(String[] args) {
String mystr = null;
System.out.println(mystr.length());
}
}
Output:
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.length()" because "mystr" is null
at com.example.myJavaProject.Demo.main(Demo.java:18)
In the above code, NullPointerException
is thrown as the length()
method is called for a null
string object without performing any null
check before calling the method.
Avoid the NullPointerException
in Java
To avoid the NullPointerException
, we must ensure all the objects/variables are initialized properly. Whenever we use a reference variable, we have to make a null
check to ensure that the object is not null
.
Let’s look at different scenarios to understand them better.
Check the Arguments of a Method Before Use
When executing a method, we must first check its arguments for null
and properly throw an IllegalArgumentException
to notify the programmer that something is wrong with the arguments passed to the method.
public class Demo {
public static int getLength(String str) {
if (str == null)
throw new IllegalArgumentException("The arguments cannot be null in a method");
return str.length();
}
public static void main(String[] args) {
String str1 = "hero";
String str2 = null;
try {
System.out.println(getLength(str1));
System.out.println(getLength(str2));
} catch (IllegalArgumentException e) {
System.out.println("IllegArgumentException is caught");
System.out.println(e.getMessage());
}
}
}
Output:
4
IllegArgumentException is caught
The arguments cannot be null in a method
Comparison of Strings With Literal
This is a common scenario where the NullPointerException
exception is thrown. A literal can be a String or the element of an Enum.
Example code:
public class Demo {
public static void main(String[] args) {
String str = null;
try {
if (str.equals("hero"))
System.out.print("Both the strings are the same");
else
System.out.print("Not equal");
} catch (NullPointerException e) {
System.out.print("NullPointerException Caught");
}
}
}
Output:
NullPointerException Caught
The above NullPointerException
can be avoided by calling the equals()
method using literals rather than the string variable.
Example code:
public class Demo {
public static void main(String[] args) {
String str = null;
try {
if ("hero".equals(str))
System.out.print("Both the strings are the same");
else
System.out.print("Not equal");
} catch (NullPointerException e) {
System.out.print("NullPointerException Caught");
}
}
}
Output:
Not equal
Handle the NullPointerException
Class in Java
To handle exceptions, Java provides a try-catch block. Here, we can use these blocks to catch NullPointerException
and avoid abnormal program termination. Check the example below:
public class SimpleTesting {
static String str;
public static void main(String[] args) {
try {
String newStr = str.toUpperCase();
System.out.println(newStr);
} catch (NullPointerException e) {
System.out.println("Null Pointer: " + e.getMessage());
}
}
}
Output:
{One=1, Two=2, Three=3}
If you already know about the null reference, you can use an if-else block to validate the variable first before applying any operation. This method is an excellent solution, but it only works when you know the null reference before. See the code here:
public class SimpleTesting {
static String str;
public static void main(String[] args) {
if (str != null) {
String newStr = str.toUpperCase();
System.out.println(newStr);
} else
System.out.println("String is null");
}
}
Output:
String is null
Encounters With NullPointerException
in Command-Line Arguments in Java
This is another scenario where a null pointer exception can occur. The args[]
array of the main()
method contains command-line arguments, and if during the runtime no argument is provided, then this points to a null reference. Getting its length will return NullPointerException
. See the example below:
public class SimpleTesting {
static String[] arr;
public static void main(String[] args) {
int length = arr.length;
System.out.println("Array length : " + length);
}
}
Output:
Exception in thread "main" java.lang.NullPointerException