How to Throw Runtime Exception in Java
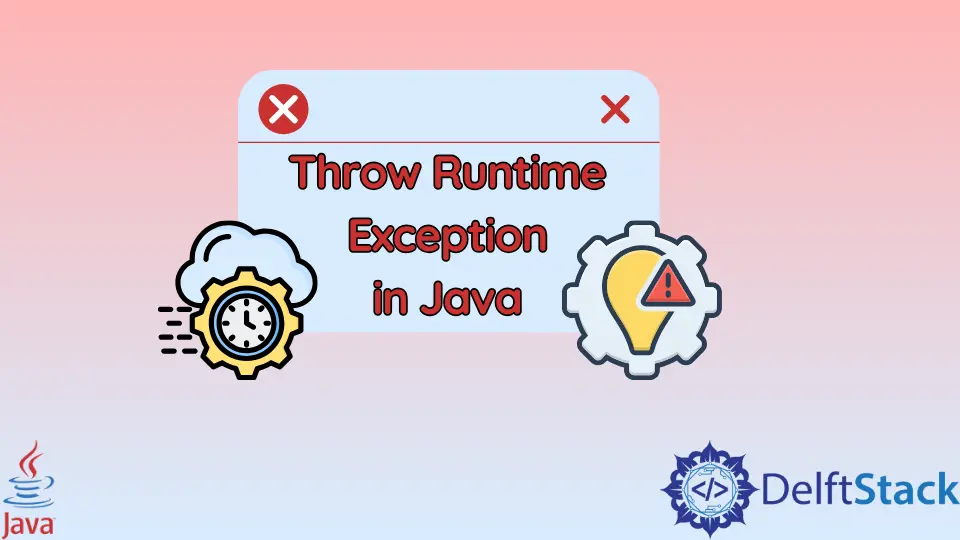
This tutorial demonstrates how to throw runtime exceptions in Java.
Throw Runtime Exception in Java
The Runtime Exception
is the parent class for all the Java exceptions that cause the program’s crash or breakdown when they occur. The Runtime Exceptions
are never checked.
The Runtime Exception
is used to show the programmer’s error. These exceptions can also be used when a condition can’t happen.
Java has some of the most used built-in Runtime Exceptions
, which include:
ArrayIndexOutOfBoundsException
- This runtime exception is thrown when we are trying to access an index of the array which doesn’t exist.InvalidArgumentException
- This runtime exception is thrown when we try to pass an invalid argument to a method of the server connection.NullPointerException
- This runtime exception is thrown when we try to access or use a null value in our code.
The above exception is only a few of the built-in runtime exceptions in Java. Java also has the functionality to create our runtime exception.
Let’s see how to create a runtime exception and throw it into our code.
- Create a class that extends the
RuntimeException
. - Create a constructor method in the class, which will run automatically when our runtime exception is thrown.
- Create another class that will be used as the driver class to throw the runtime exception.
Let’s try to implement the above scenario in Java.
package delftstack;
class DemoException extends RuntimeException {
public DemoException() {
System.out.println("This is the Demo Runtime Exception!");
}
}
public class Example {
public void Throw_RuntimeException() {
throw new DemoException();
}
public static void main(String[] args) {
try {
new Example().Throw_RuntimeException();
} catch (Exception e) {
e.printStackTrace();
}
}
}
The code above creates a custom exception by extending the RuntimeException
, and the driver class throws it. See output:
This is the Demo Runtime Exception!
delftstack.DemoException
at delftstack.Example.Throw_RuntimeException(Example.java:11)
at delftstack.Example.main(Example.java:15)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook