Runtime Exception in Java
-
Understanding
java.lang.RuntimeException
in Java -
Difference Between
java.lang.RuntimeException
andjava.lang.Exception
- Conclusion
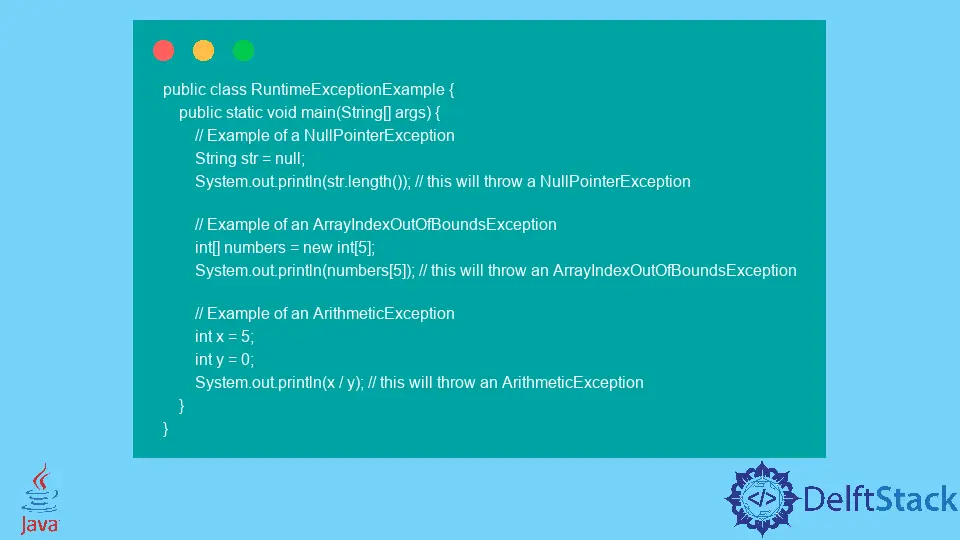
In this article, we will learn about java.lang.RuntimeException
in Java.
Understanding java.lang.RuntimeException
in Java
Java runtime exceptions are a type of exception that occurs during the execution of a program. They are typically caused by programming errors, such as an attempt to divide by zero or access an array index that is out of bounds.
Unlike checked exceptions, which are intended to be handled by the developer, runtime exceptions are considered unanticipated and therefore do not need to be explicitly caught or declared in a method’s signature.
One of Java’s most common runtime exceptions is the NullPointerException
, which occurs when an application attempts to call a method or access a field on a null object reference. This can occur when a developer forgets to initialize an object or when an object is assigned a null
value.
Another common runtime exception is the ArrayIndexOutOfBoundsException
, which occurs when an application attempts to access an array index outside the valid range for that array. This can occur when a developer incorrectly calculates the size of an array or when a loop iterates through an array using an index that is too large.
Developers need to understand the causes of these and other runtime exceptions to prevent them from occurring in their code. This can be done through careful testing and debugging and using tools such as code analysis tools to identify potential issues.
In general, runtime exceptions should be considered a sign of a bug in the program and should be fixed as soon as they are discovered. Developers should always strive to write robust, reliable code that can gracefully handle unexpected situations.
Example
Let’s see a small example to understand it better.
public class RuntimeExceptionExample {
public static void main(String[] args) {
// Example of a NullPointerException
String str = null;
System.out.println(str.length()); // this will throw a NullPointerException
// Example of an ArrayIndexOutOfBoundsException
int[] numbers = new int[5];
System.out.println(numbers[5]); // this will throw an ArrayIndexOutOfBoundsException
// Example of an ArithmeticException
int x = 5;
int y = 0;
System.out.println(x / y); // this will throw an ArithmeticException
}
}
In the above example, a NullPointerException
is thrown because the str
variable is null
and the length()
method is called on it.
In the second example, an ArrayIndexOutOfBoundsException
is thrown because the array numbers only have 5 elements, and we are trying to access the 6th element. In the third example, an ArithmeticException
is thrown because we try to divide by zero.
It’s important to note that in practice, these examples should be handled properly and not let the exception be thrown. For example, in the first example, the null
pointer check should be done before calling the length
method.
public class RuntimeExceptionExample {
public static void main(String[] args) {
// Example of a NullPointerException
String str = null;
if (str != null)
System.out.println(str.length());
else
System.out.println("String is null");
// Example of an ArrayIndexOutOfBoundsException
int[] numbers = new int[5];
if (numbers.length > 5)
System.out.println(numbers[5]);
else
System.out.println("Array index is out of bound");
// Example of an ArithmeticException
int x = 5;
int y = 0;
if (y != 0)
System.out.println(x / y);
else
System.out.println("Cannot divide by zero");
}
}
It’s important to handle these exceptions properly to prevent the application from crashing.
Now let’s see the difference between java.lang.RuntimeException
and java.lang.Exception
, one common place where many developers get confused.
Difference Between java.lang.RuntimeException
and java.lang.Exception
In Java, java.lang.RuntimeException
and java.lang.Exception
are both types of exceptions that can be thrown during the execution of a program. However, there are some key differences between the two.
-
Checked vs. Unchecked:
java.lang.Exception
is a checked exception, meaning that the compiler requires the programmer to handle it or declare it in athrows
clause. On the other hand,java.lang.RuntimeException
is an unchecked exception, meaning that the compiler does not require the programmer to handle it. -
Types of errors:
java.lang.Exception
typically represents errors resulting from external factors, such as issues with input/output operations or issues with the environment. On the other hand,java.lang.RuntimeException
typically represents errors resulting from programming errors, such as null pointer exceptions or illegal arguments. -
Use:
java.lang.Exception
is generally used for exceptional cases that you expect to occur and can be handled in some way. For example, when you are reading a file, you expect that the file might not exist, and you handle theFileNotFoundException
accordingly.On the other hand,
java.lang.RuntimeException
is generally used for programming errors that you don’t expect to occur and that you can’t handle. For example, a null pointer exception results from a programming error, it should not occur in normal operation, and you can’t handle it. -
Inheritance:
java.lang.RuntimeException
is a subclass ofjava.lang.Exception
, so all runtime exceptions are exceptions. But not all exceptions are runtime exceptions.
In summary, java.lang.RuntimeException
is a type of exception that represents programming errors and is not required to be handled by the programmer, while java.lang.Exception
represents exceptional cases that the programmer can handle.
Conclusion
In conclusion, java.lang.RuntimeException
is a type of exception that represents programming errors in Java. These errors are typically caused by null pointer exceptions, illegal arguments, or index out-of-bounds errors.
Unlike checked exceptions, which the programmer requires, runtime exceptions are not required to be handled, and the program will terminate if one is thrown.
It’s important to note that runtime exceptions are not the only type of exception that can be thrown in a Java program, and it’s important to be familiar with both checked
and unchecked
exceptions to write robust and reliable code.
To handle these exceptions, it’s good practice to use the try-catch
block, which allows the program to catch and handle the exception before it terminates the program.