How to Throw New Exception in Java
- Understanding Exceptions in Java
- Creating a Custom Exception Class
- Throwing Built-in Exceptions
- Conclusion
- FAQ
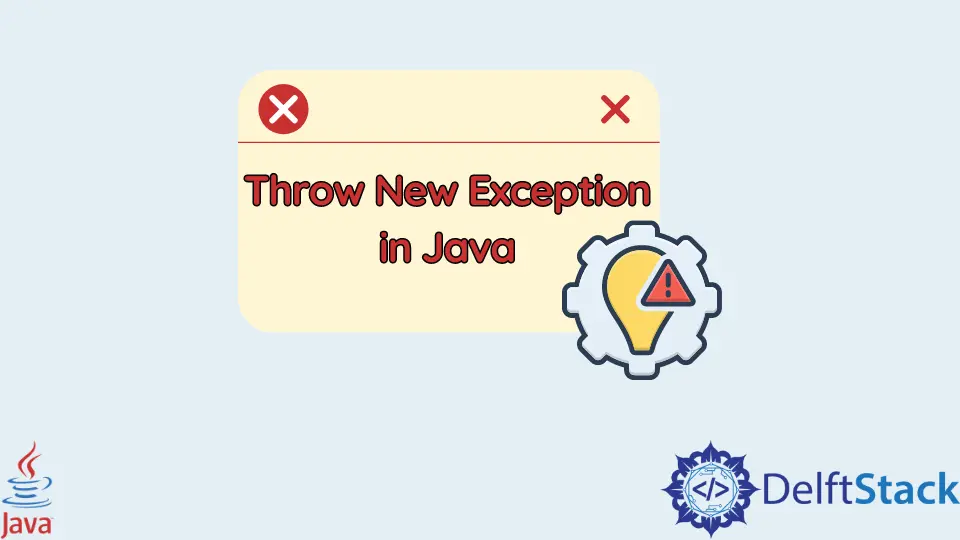
When working with Java, understanding exceptions is crucial for writing robust and error-free code. Exceptions are events that disrupt the normal flow of a program, and handling them effectively can save you from unexpected crashes.
In this tutorial, we will explore how to throw a new exception in Java, including the different types of exceptions and practical examples. By the end of this guide, you will have a clear understanding of how to create and throw exceptions, making your Java applications more resilient and easier to debug. Let’s dive in!
Understanding Exceptions in Java
In Java, exceptions are categorized into two main types: checked exceptions and unchecked exceptions. Checked exceptions are those that the compiler forces you to handle, while unchecked exceptions, such as NullPointerException
, do not require explicit handling. Throwing a new exception allows you to create custom error messages and handle specific scenarios in your code.
Checked Exceptions
Checked exceptions are part of the method signature. For instance, if you are working with file operations, you might encounter IOException
. To throw a checked exception, you must declare it in the method signature using the throws
keyword.
Unchecked Exceptions
Unchecked exceptions do not need to be declared. These include runtime exceptions, which can occur at any point during program execution. For example, you can throw a new IllegalArgumentException
when a method receives an invalid argument.
Creating a Custom Exception Class
Creating a custom exception class in Java is straightforward. You can extend the Exception
class or any of its subclasses. This allows you to define specific behavior and messages for your exception.
public class MyCustomException extends Exception {
public MyCustomException(String message) {
super(message);
}
}
In this code snippet, we define a custom exception called MyCustomException
. It extends the Exception
class and takes a message as a parameter. This message will be passed to the superclass constructor, which sets the exception message.
Once you have defined your custom exception, you can use it in your application. For instance, you might want to throw this exception when a specific condition is met, such as an invalid input.
public void validateInput(int number) throws MyCustomException {
if (number < 0) {
throw new MyCustomException("Input cannot be negative.");
}
}
In this example, the validateInput
method checks if the input number is negative. If it is, it throws our custom exception with a descriptive message. This ensures that the calling method is aware of the issue and can handle it appropriately.
Throwing Built-in Exceptions
In many cases, you may want to throw built-in exceptions provided by Java. This is useful when you want to leverage Java’s standard error handling mechanisms. For example, you can throw an IllegalArgumentException
when a method receives an inappropriate argument.
public void setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative.");
}
}
Here, the setAge
method throws an IllegalArgumentException
if the provided age is negative. By using built-in exceptions, you can communicate issues effectively without having to create custom classes for common scenarios.
Catching Exceptions
It’s important to handle exceptions properly to ensure your application remains stable. You can use a try-catch
block to catch exceptions and take appropriate actions.
try {
validateInput(-1);
} catch (MyCustomException e) {
System.out.println("Caught an exception: " + e.getMessage());
}
In this code, we attempt to validate an input of -1. Since this will throw a MyCustomException
, we catch it and print the exception message. This way, your program can continue running even when an error occurs.
Output:
Caught an exception: Input cannot be negative.
Conclusion
Throwing and handling exceptions in Java is a vital skill for any developer. By creating custom exceptions and utilizing built-in ones, you can manage errors effectively and improve the robustness of your applications. Whether you are working on a small project or a large enterprise application, understanding how to throw new exceptions will make your code cleaner and easier to maintain. Remember to always catch exceptions where necessary to keep your application running smoothly. Happy coding!
FAQ
-
What are the two main types of exceptions in Java?
Checked exceptions and unchecked exceptions. -
How do I create a custom exception in Java?
Create a new class that extends the Exception class and define a constructor that accepts a message. -
Can I throw built-in exceptions in Java?
Yes, you can throw built-in exceptions like IllegalArgumentException and IOException. -
What is the purpose of the try-catch block?
The try-catch block is used to handle exceptions gracefully without crashing the program. -
Can I throw multiple exceptions from a single method?
Yes, a method can throw multiple exceptions, and you can declare them using the throws keyword.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn