Java Throwable VS Exception Class
-
Introduction to
Throwable
Class in Java -
Introduction to
Exception
Class in Java -
Which Class Should We Use,
Throwable
orException
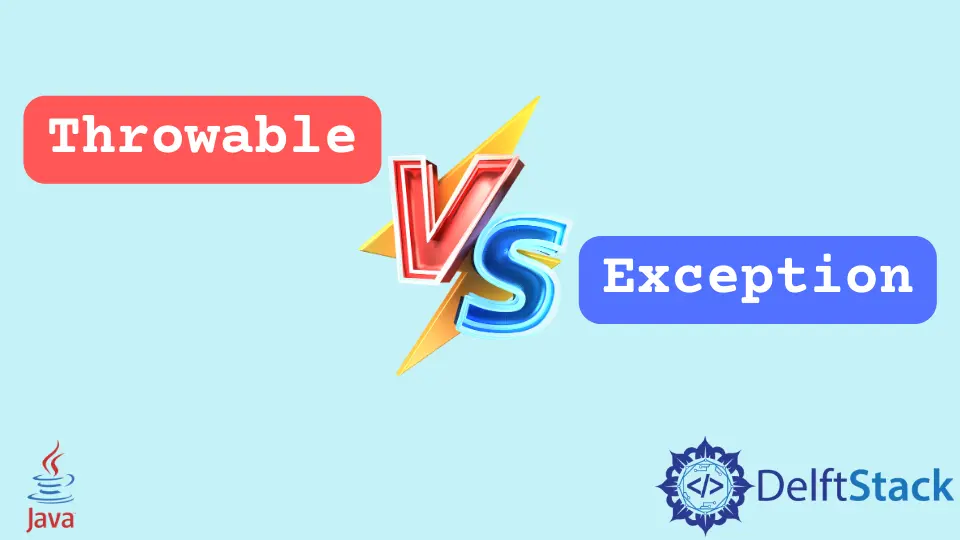
This article will teach us when to use the Throwable
and Exception
classes in Java. Both classes have their constructors and methods to handle errors and exceptions.
First, we will see the introduction of Throwable
and Exception
of both classes, and after that, we will learn the difference between both.
Introduction to Throwable
Class in Java
In the hierarchy of the java.lang
classes, the Throwable
class is the parent or superclass of the Error
and Exception
classes. It means the Error
and Exception
are inherited by the Throwable
class in Java.
It concludes that the Throwable
class can catch all the errors and exceptions produced by the Java Virtual Machine, either stack trace errors or compile or run time exceptions.
Furthermore, the Throwable
class implements the Serializable
interface in the java.lang
library where it is defined.
Users can see the definition of the Throwable
class below.
public class Throwable extends Object implements Serializable
In the above class definition, users can see that the Throwable
class inherits the Object
class and implements the Serializable
interface.
Syntax of Throwable
:
try {
// some code
} catch (Throwable e) {
// handle errors or exceptions
}
The Throwable
class contains the overloaded constructors, which are explained below.
Constructors
Throwable() |
This constructor creates a new instance of Throwable with a default error message. |
Throwable(String errorMessage) |
This constructor creates a new instance of Throwable with an errorMessage message. |
Throwable(String errorMessage, Throwable errorCause) |
This constructor also takes the cause of the error message and creates a new instance of Throwable with errorMessage and errorCause . |
Throwable(Throwable errorCause) |
This constructor creates the new instance of Throwable with a specific cause passed as a parameter. |
Methods
Here we have explained some methods of the Throwable
class.
toString() |
It returns the description of the error in string format. |
getMessage() |
It returns the error message in string format. |
getCause() |
It returns the cause of the current instance of a Throwable . |
getLocalizedMessage() |
It returns the localized description of Throwable . |
getStackTrace() |
It provides information about the stack trace. |
printStackTrace() |
It prints the current instance of Throwable . |
setStackTrace(StackTraceElement[] stackTrace) |
It allows setting the element of stack trace returned by the getStackTrace() method. |
initCause(Throwable cause) |
It allows users to initialize the cause of the current Throwable . |
Errors
The Errors
class includes errors that stop the program’s execution.
StackOverflowError |
It occurs when stack or heap overflow occurs. |
AssertionError |
It happens due to assertion failure. |
VirtualMachineError |
It caches all the errors in Java Virtual Machine. |
LinkageError |
It occurs due to a Linkage problem. |
OutOfMemoryError |
It occurs due to low memory on your device or JVM running out of memory. |
Introduction to Exception
Class in Java
In Java, exceptions are unwanted events that occur at runtime or compile time. The exceptions can be caught at the compile time, called the checked exceptions, and exceptions can be caught only at the run time, called the unchecked exceptions.
Syntax of Throwable
:
try {
// some code
} catch (Exception e) {
// handle Exceptions only
}
Here, we have defined some types of exceptions in Java.
RuntimeException |
All classes of unchecked exceptions inherit it. |
NullPointerException |
It occurs due to a null or undefined value. |
ArithmeticException |
It occurs due to the failure of an arithmetic operation. |
ArrayIndexOutOfBoundsException |
It occurs when we try to access an array index greater than its length. |
NoSuchMethodException |
It occurs when the method is not defined. |
IOException |
It occurs due to the failure of input/output operations. |
ClassNotFoundException |
It occurs when the class is not defined. |
SQLException |
The failure of database operation is the cause of this exception. |
Programmers can also define exceptions by inheriting Java’s Exception
class.
Which Class Should We Use, Throwable
or Exception
When we look at the syntax of the Throwable
and Exception
classes, it looks similar at first sight as we are using both with the try-catch
block.
The instance of the Throwable
class can catch the errors and exceptions as they are a subclass of Throwable
, but the instance of Exceptions
can only catch the exceptions that occur in the program.
In normal Java applications, rarely, errors such as LinkageError
, VirtualMachineError
, OutOfMemoryError
, etc., occur. Mainly exception occurs due to the user’s mistake.
So, it is a best practice to use the instance of the Exception
class rather than the Throwable
class.