在 Java 中计算字符串中的字符数
-
使用
String.length()
来计算 Java 字符串中的总字符数 - 使用 Java 8 流来计算 Java 字符串中的字符数
-
使用循环和
charAt()
来计算一个 Java 字符串中的特定字符
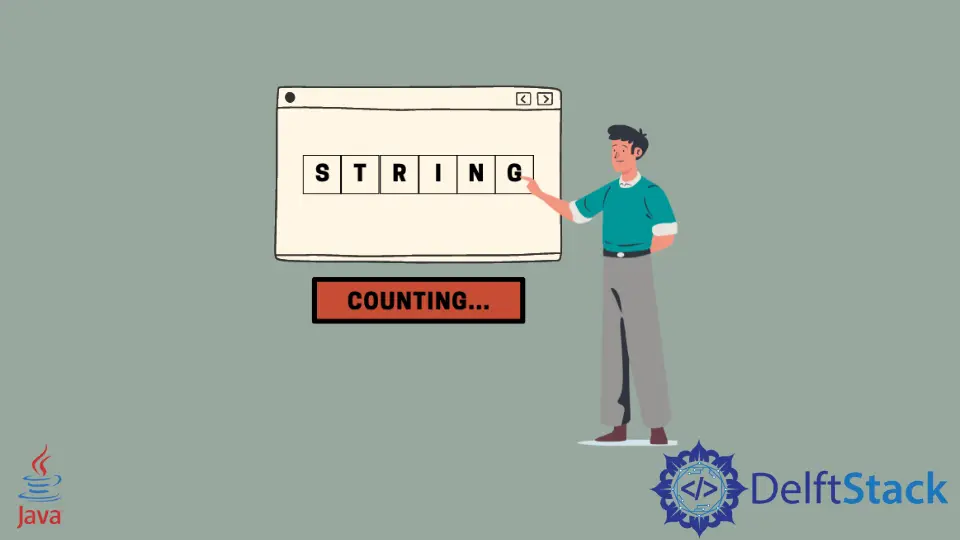
今天,我们将介绍多种方法来计算给定 Java 字符串中的字符数量。我们将对总字符和特定字符进行计数。
使用 String.length()
来计算 Java 字符串中的总字符数
最常见的做法是使用 length()
方法来获取一个 Java 字符串的总字符数。在下面的代码中,我们有一个字符串 exampleString
,将使用 exampleString.length()
来获取这个字符串的总长度。
输出结果显示,exampleString
中有 28 个字符,而它其实只有只有 23 个字符。这是因为 String.length()
也计算了空白字符。为了解决这个问题,我们可以使用 replace()
函数将所有的空白字符替换成一个不被计算的空字符。最后,我们可以得到不含空格的字符串的长度,即 23。
public class CountCharsInString {
public static void main(String[] args) {
String exampleString = "This is just a sample string";
int stringLength = exampleString.length();
System.out.println("String length: " + stringLength);
int stringLengthWithoutSpaces = exampleString.replace(" ", "").length();
System.out.println("String length without counting whitespaces: " + stringLengthWithoutSpaces);
}
}
输出:
String length: 28
String length without counting whitespaces: 23
使用 Java 8 流来计算 Java 字符串中的字符数
另一种计算字符串中所有字符的方法是使用 String.chars().count()
方法,该方法返回字符串中包含空格的总字符数格。由于 chars()
是一个流,我们可以使用 filter()
方法来忽略空格。filter(ch -> ch != '')
检查每一个字符,如果发现有空格,就将其过滤掉。
public class CountCharsInString {
public static void main(String[] args) {
String exampleString = "This is just a sample string";
long totalCharacters = exampleString.chars().filter(ch -> ch != ' ').count();
System.out.println("There are total " + totalCharacters + " characters in exampleString");
}
}
输出:
There are total 23 characters in exampleString
使用循环和 charAt()
来计算一个 Java 字符串中的特定字符
我们一直在计算一个字符串中的总字符数,但下面的例子显示了计算字符串中一个特定字符的方法。我们的目标是得到 exampleString
中的 i
的数量。为了实现这个目标,我们使用了一个循环,一直运行到字符串结束为止。
我们创建了两个额外的变量:totalCharacters
将保存计数,temp
将使用 exampleString.charAt(i)
保存每个单独的字符。为了检查我们的字符的出现,我们将把 temp
和我们的字符进行比较,检查它们是否匹配。如果发现匹配,那么 totalCharacters
将增加 1。循环结束后,我们可以看到字符在字符串中的总出现次数。
public class CountCharsInString {
public static void main(String[] args) {
String exampleString = "This is just a sample string";
int totalCharacters = 0;
char temp;
for (int i = 0; i < exampleString.length(); i++) {
temp = exampleString.charAt(i);
if (temp == 'i')
totalCharacters++;
}
System.out.println("i appears " + totalCharacters + " times in exampleString");
}
}
输出:
i appears 3 times in exampleString
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn相关文章 - Java String
- 如何从 Java 中的字符串中删除子字符串
- 如何将 Java 字符串转换为字节
- 如何在 Java 中以十六进制字符串转换字节数组
- 如何在 Java 中执行字符串到字符串数组的转换
- 用 Java 生成随机字符串
- Java 中的交换方法