在 Java 中計算字串中的字元數
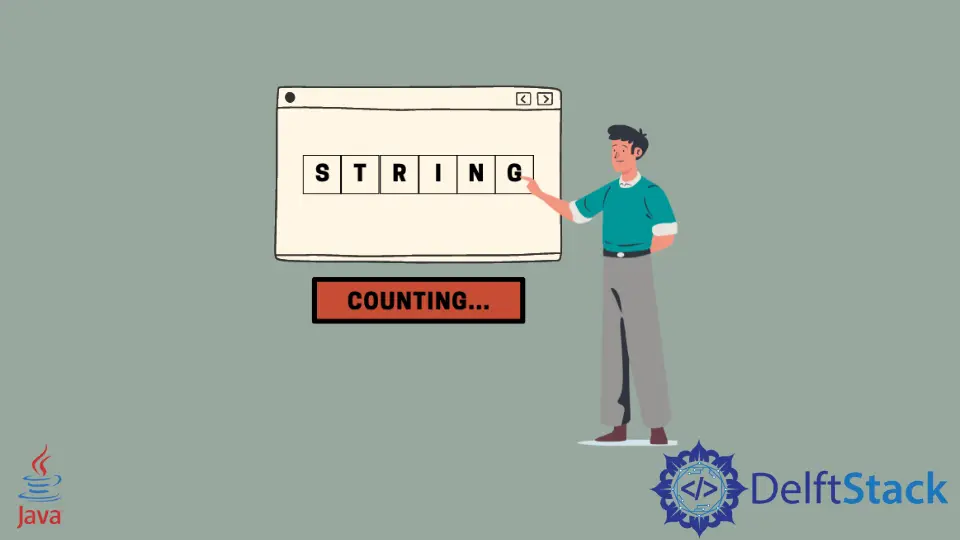
今天,我們將介紹多種方法來計算給定 Java 字串中的字元數量。我們將對總字元和特定字元進行計數。
使用 String.length()
來計算 Java 字串中的總字元數
最常見的做法是使用 length()
方法來獲取一個 Java 字串的總字元數。在下面的程式碼中,我們有一個字串 exampleString
,將使用 exampleString.length()
來獲取這個字串的總長度。
輸出結果顯示,exampleString
中有 28 個字元,而它其實只有只有 23 個字元。這是因為 String.length()
也計算了空白字元。為了解決這個問題,我們可以使用 replace()
函式將所有的空白字元替換成一個不被計算的空字元。最後,我們可以得到不含空格的字串的長度,即 23。
public class CountCharsInString {
public static void main(String[] args) {
String exampleString = "This is just a sample string";
int stringLength = exampleString.length();
System.out.println("String length: " + stringLength);
int stringLengthWithoutSpaces = exampleString.replace(" ", "").length();
System.out.println("String length without counting whitespaces: " + stringLengthWithoutSpaces);
}
}
輸出:
String length: 28
String length without counting whitespaces: 23
使用 Java 8 流來計算 Java 字串中的字元數
另一種計算字串中所有字元的方法是使用 String.chars().count()
方法,該方法返回字串中包含空格的總字元數格。由於 chars()
是一個流,我們可以使用 filter()
方法來忽略空格。filter(ch -> ch != '')
檢查每一個字元,如果發現有空格,就將其過濾掉。
public class CountCharsInString {
public static void main(String[] args) {
String exampleString = "This is just a sample string";
long totalCharacters = exampleString.chars().filter(ch -> ch != ' ').count();
System.out.println("There are total " + totalCharacters + " characters in exampleString");
}
}
輸出:
There are total 23 characters in exampleString
使用迴圈和 charAt()
來計算一個 Java 字串中的特定字元
我們一直在計算一個字串中的總字元數,但下面的例子顯示了計算字串中一個特定字元的方法。我們的目標是得到 exampleString
中的 i
的數量。為了實現這個目標,我們使用了一個迴圈,一直執行到字串結束為止。
我們建立了兩個額外的變數:totalCharacters
將儲存計數,temp
將使用 exampleString.charAt(i)
儲存每個單獨的字元。為了檢查我們的字元的出現,我們將把 temp
和我們的字元進行比較,檢查它們是否匹配。如果發現匹配,那麼 totalCharacters
將增加 1。迴圈結束後,我們可以看到字元在字串中的總出現次數。
public class CountCharsInString {
public static void main(String[] args) {
String exampleString = "This is just a sample string";
int totalCharacters = 0;
char temp;
for (int i = 0; i < exampleString.length(); i++) {
temp = exampleString.charAt(i);
if (temp == 'i')
totalCharacters++;
}
System.out.println("i appears " + totalCharacters + " times in exampleString");
}
}
輸出:
i appears 3 times in exampleString
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn相關文章 - Java String
- 如何在 Java 中以十六進位制字串轉換位元組陣列
- 如何在 Java 中執行字串到字串陣列的轉換
- 如何將 Java 字串轉換為位元組
- 如何從 Java 中的字串中刪除子字串
- 用 Java 生成隨機字串
- Java 中的交換方法