How to Add String to an Array in C#
-
Add String to Array With the
List.Add()
Method inC#
-
Add String to Array With the
Array.Resize()
Method inC#
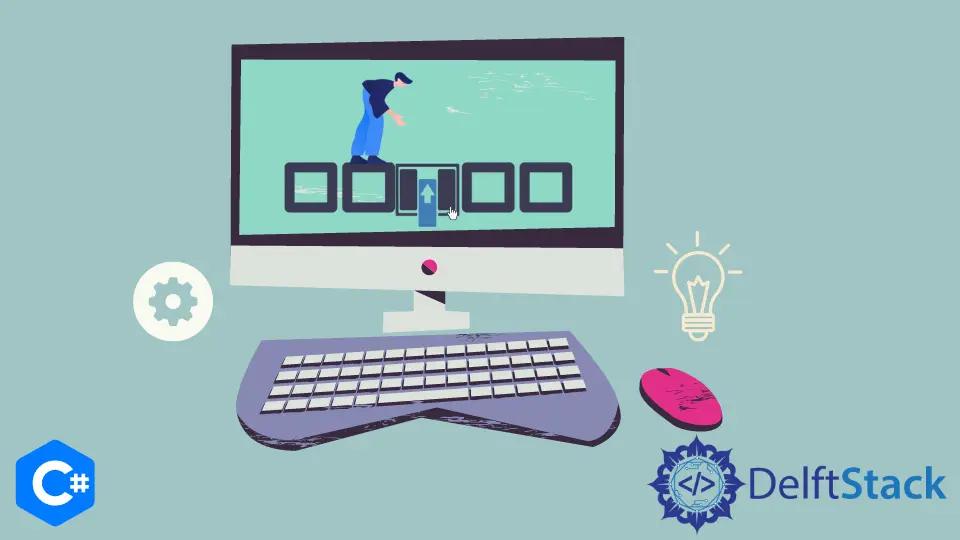
This tutorial will discuss methods to add new strings to a wholly filled array in C#.
Add String to Array With the List.Add()
Method in C#
Unfortunately, there is no built-in method for adding new values to an array in C#. The List data structure should be used for dynamic allocation and de-allocation of values in C#. But, if we have a filled array containing some important data and want to add a new element to the array, we can follow the following procedure. The Linq is used to integrate query functionality of the SQL on data structures in C#. We can use the ToList()
method of Linq to convert our array to a list and then add values to the list with the List.Add()
method in C#. In the end, we can convert the list back to our array with the List.ToArray()
method. The following code example shows us how we can add a new element to a completely filled array with the List.Add()
method in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace Array_Add {
class Program {
static void Main(string[] args) {
string[] arr = { "One", "Two", "Three" };
string newElement = "New Element";
List<string> list = new List<string>(arr.ToList());
list.Add(newElement);
arr = list.ToArray();
foreach (var e in arr) {
Console.WriteLine(e);
}
}
}
}
Output:
One
Two
Three
New Element
We initialized the array of strings arr
and the string variable newElement
in the above code. We converted the arr
array to the list list
with the arr.ToList()
method. We then added the newElement
to the list
with the list.Add(newElement)
method. In the end, we converted the list
list back to the arr
array with the list.ToArray()
method in C#.
Add String to Array With the Array.Resize()
Method in C#
We can also use the following to add a new element to a completely filled array in C#. The Array.Resize()
method changes the number of elements of a one-dimensional array in C#. The Array.Resize()
method takes a reference to the array and its new length as parameters and resizes that array. We can increase our array size by one element each time we have to add a new element to it. The following code example shows us how to add a new element to a completely filled array with the Array.Resize()
method in C#.
using System;
using System.Collections.Generic;
namespace Array_Add {
class Program {
static void Main(string[] args) {
string[] arr = { "One", "Two", "Three" };
string newElement = "New Element";
Array.Resize(ref arr, arr.Length + 1);
arr[arr.Length - 1] = newElement;
foreach (var e in arr) {
Console.WriteLine(e);
}
}
}
}
Output:
One
Two
Three
New Element
We initialized the array of strings arr
and the newElement
string in the above code. We increased the size of the arr
array by one element with the Array.Resize()
function and assigned newElement
to the last index of the arr
array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Array
- How to Get the Length of an Array in C#
- How to Sort an Array in C#
- How to Sort an Array in Descending Order in C#
- How to Remove Element of an Array in C#
- How to Convert a String to a Byte Array in C#
- How to Adding Values to a C# Array
Related Article - Csharp List
- How to Convert an IEnumerable to a List in C#
- How to Remove Item From List in C#
- How to Join Two Lists Together in C#
- How to Get the First Object From List<Object> Using LINQ
- How to Find Duplicates in a List in C#
- The AddRange Function for List in C#