How to Convert Int to String in C#
-
C#
int
tostring
Conversion -Int16.ToString()
/Int32.ToString()
/Int64.ToString()
Method -
C#
int
tostring
Conversion -Convert.ToString()
Method -
C#
int
tostring
Conversion -String.Format()
Method -
C#
int
tostring
Conversion -StringBuilder
Method -
C#
int
tostring
Conversion - With+
Operator
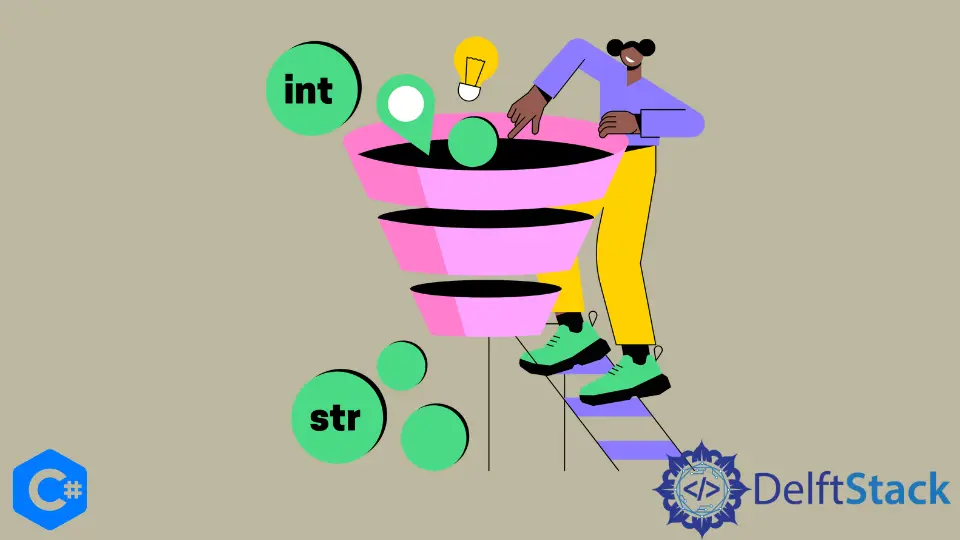
C# has different methods to convert int to string.
This article introduces methods like the ToString
method, Convert.ToString
method, string formatting, and StringBuilder
method.
C# int
to string
Conversion - Int16.ToString()
/ Int32.ToString()
/ Int64.ToString()
Method
The ToString()
method of the Int16/32/64
data type converts the integer to the string representation and is mainly for the purpose of the display.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = num.ToString();
System.Console.WriteLine(numString);
}
}
Output:
80
C# int
to string
Conversion - Convert.ToString()
Method
Convert
class in the System
namespace converts a data type to another data type. Convert.ToString()
method converts the given value to its string representation.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = Convert.ToString(num);
System.Console.WriteLine(numString);
}
}
Output:
80
C# int
to string
Conversion - String.Format()
Method
The String.Format
method converts the given objects to strings by following the specified formats.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = string.Format("{0}", num);
System.Console.WriteLine(numString);
}
}
Here, {0}
is the format item, 0
is the start index of the object whose string representation is inserted at that position.
C# int
to string
Conversion - StringBuilder
Method
StringBuilder
from the System.Text
namespace is a mutable string of characters. A StringBuilder
object keeps a buffer to append more characters to the string.
using System;
using System.Text;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = new StringBuilder().Append(num).ToString();
System.Console.WriteLine(numString);
}
}
When the argument of StringBuilder
is empty, it instantiates a StringBuilder
with the value of String.Empty
.
Append(num)
appends the string representation of num
to the StringBuilder
.
ToString()
methods converts the StringBuilder
type to string
.
C# int
to string
Conversion - With +
Operator
If a string
variable and an int
variable are added by the +
operator, it will automatically call the int.ToString()
method to convert the integer to the string that will be concatenated with the given string
variable.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = "" + num;
System.Console.WriteLine(numString);
}
}
Output:
80
string numString = "" + num;
It equivalently converts int
to string
only if the other variable of the +
operator is an empty string - ""
or String.Empty
.
""
could be either before int
or after int
. Both are the same in the behavior.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = num + "";
System.Console.WriteLine(numString);
}
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Csharp Integer
- How to Convert Int to Enum in C#
- Random Int in C#
- Random Number in a Range in C#
- How to Convert String to Int in C#
- Integer Division in C#