How to Convert a String to Float in C#
-
Use the
Parse()
Method to Convert a String to Float inC#
-
Use the
ToDouble()
Method to Convert a String to Float inC#
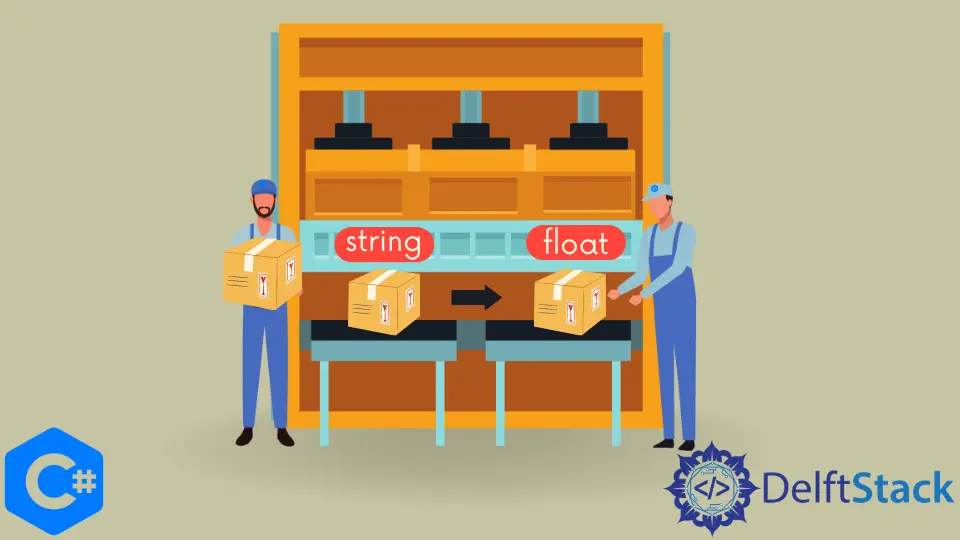
This article will introduce different methods to convert a string to float in C#, like the Parse()
and ToDouble()
method.
Use the Parse()
Method to Convert a String to Float in C#
In C#, we can use the Parse()
method to convert a string to a float value. There are multiple overloads of this method. The overload that we will use will have two parameters. One of the parameters will be the CultureInfo
object. We will use the following overload in this case. The correct syntax to use this method is as follows.
float.Parse(String stringName, CultureInfo objectName);
This overload of the method Parse()
has two parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
stringName |
mandatory | This is the string that we want to convert to float. |
objectName |
mandatory | It is the CultureInfo object that will provide the format of the float value. |
This function returns a float value representing the value given in the string.
The program below shows how we can use the Parse()
method to convert a string to float
.
using System;
using System.Globalization;
class StringToFloat {
static void Main(string[] args) {
string mystring = "134.4365790132273892";
float value = float.Parse(mystring, CultureInfo.InvariantCulture.NumberFormat);
Console.WriteLine(value);
}
}
Output:
134.4365790132273892
Use the ToDouble()
Method to Convert a String to Float in C#
In C#, we can also use the ToDouble()
method to convert a string to a float value. This method gives a more precise answer. There are multiple overloads of this method. The overload that we will use will have only one parameter. We will use the following overload in this case. The correct syntax to use this method is as follows.
Convert.ToDouble(String stringName);
This overload of the method ToDouble()
has one parameter only. The detail of its parameter is as follows.
Parameters | Description | |
---|---|---|
stringName |
mandatory | This is the string that we want to convert to float. |
This function returns a float value representing the value given in the string.
The program below shows how we can use the ToDouble()
method to convert a string to float.
using System;
using System.Globalization;
class StringToFloat {
static void Main(string[] args) {
string mystring = "134.4365790132273892";
double value = Convert.ToDouble(mystring);
Console.WriteLine(value);
}
}
Output:
134.4365790132273892
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to a Byte Array in C#