How to Convert Float to Int in C#
-
Convert Float to Int With Explicit Typecasting in
C#
-
Convert Float to Int With
Math.Ceiling()
Function inC#
-
Convert Float to Int With
Math.Floor()
Function inC#
-
Convert Float to Int With
Math.Round()
Function inC#
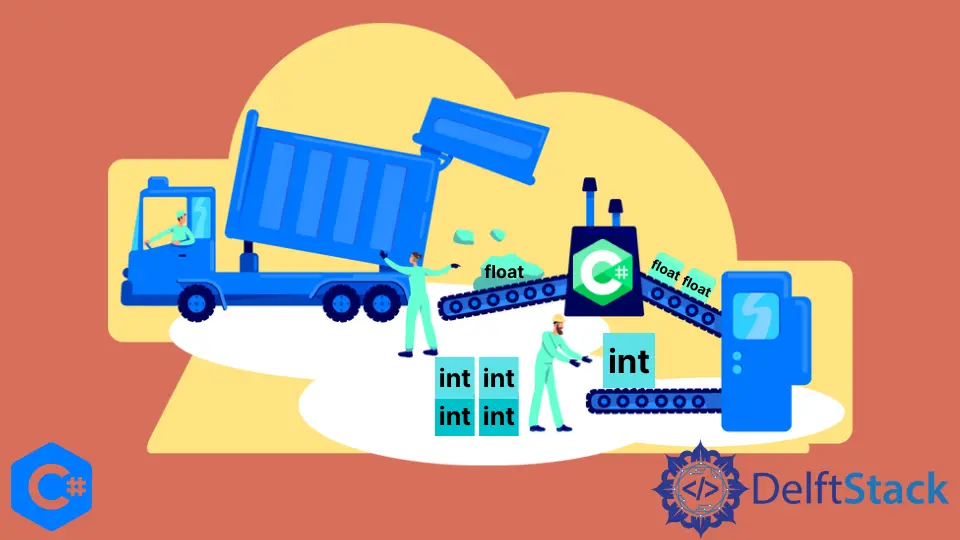
This tutorial will introduce methods to convert a float value to an integer value in C#.
Convert Float to Int With Explicit Typecasting in C#
Typecasting is a way of converting a value from one data type to another data type. The Float data type takes more bytes than the Int data type. So, we have to use explicit typecasting to convert the float value to an Int value. The following code example shows us how to convert a float value to an integer value with explicit typecasting in C#.
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.2f;
int i = (int)f;
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
Output:
Converted float 10.2 to int 10
We converted the float variable f
to the integer variable i
with explicit typecasting in C#. The (int)
is used to cast f
to i
. The problem with this approach is that it ignores all the values after the decimal point. For example, the float value 10.9
is also converted to the integer value 10
.
Convert Float to Int With Math.Ceiling()
Function in C#
If we want to consider the values after the decimal point, we have to use some approach other than explicit typecasting. The Math.Ceiling()
function converts a decimal value to the next integer value. The Math.Ceiling()
function returns a double value which can be converted to an integer value using explicit typecasting. The following code example shows us how we can convert a float value to an integer value with the Math.Ceiling()
function in C#.
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.2f;
int i = (int)Math.Ceiling(f);
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
Output:
Converted float 10.8 to int 11
We converted the float variable f
to the integer variable i
with Math.Ceiling()
function in C#. The (int)
is used to cast the double value returned by the Math.Ceiling()
function to an integer value. The problem with this approach is that it always returns the next integer value. For example, the float value 10.1
is also converted to the integer value 11
.
Convert Float to Int With Math.Floor()
Function in C#
The Math.Floor()
function can also be used along with explicit typecasting to convert a float value to an integer value in C#. The Math.Floor()
function is used to convert the decimal value to the previous integer value. The Math.Floor()
function returns a double value that can be converted to an integer value with explicit typecasting. The following code example shows us how we can convert a float value to an integer value with the Math.Floor()
function in C#.
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.2f;
int i = (int)Math.Floor(f);
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
Output:
Converted float 10.2 to int 10
We converted the float variable f
to the integer variable i
with the Math.Floor()
function in C#. The (int)
is used to cast the double value returned by the Math.Floor()
function to an integer value. The problem with this approach is that it always returns the previous integer value. For example, the float value 10.9
is also converted to the integer value 10
.
Convert Float to Int With Math.Round()
Function in C#
The approaches discussed above do work, but there is some drawback of every approach. If we want to convert a float value to an integer value but do not want our data to change, we can use the Math.Round()
function in C#. The Math.Round()
function, as the name suggests, is used to round up decimal values to the closest integer value. The Math.Round()
returns a double value, which can be converted to the integer data type with explicit typecasting. The following code example shows us how we can convert a float value to an integer value with the Math.Round()
function in C#.
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.8f;
int i = (int)Math.Round(f);
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
Output:
Converted float 10.8 to int 11
We converted the float variable f
to the integer variable i
with the Math.Round()
function in C#. The (int)
is used to cast the double value returned by the Math.Round()
function to an integer value. This is the optimal approach for converting a float value to an integer value in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Float
- How to Convert a String to Float in C#
- How to Convert Int to Float in C#
- Float vs Double vs Decimal in C#
- How to Generate a Random Float in C#