在 C# 中把浮点数转换为 Int
- 使用 C# 中的显式类型转换将 Float 转换为 Int
-
在 C# 中使用
Math.Ceiling()
函数将 Float 转换为 Int -
在 C# 中使用
Math.Floor()
函数将 Float 转换为 Int -
在 C# 中使用
Math.Round()
函数将 Float 转换为 Int
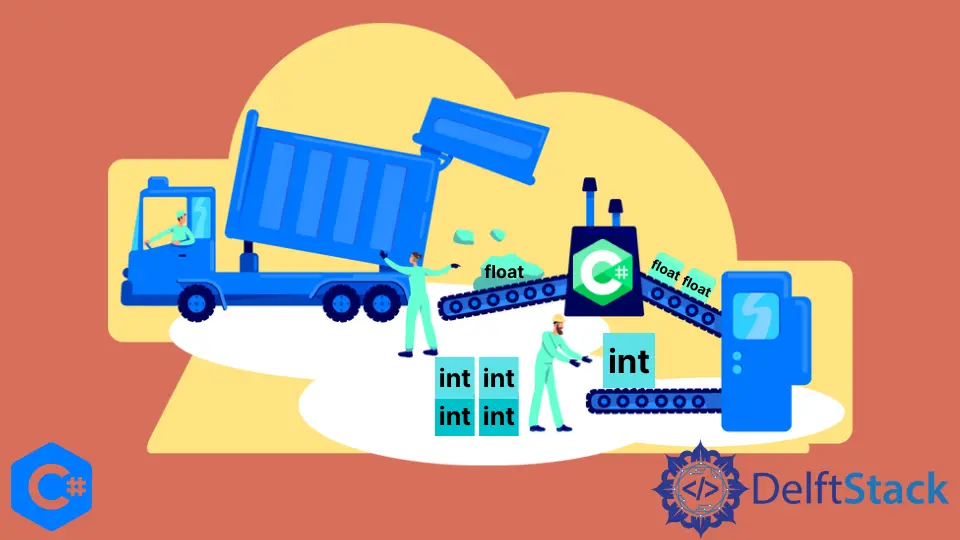
本教程将介绍在 C# 中将浮点数转换为整数值的方法。
使用 C# 中的显式类型转换将 Float 转换为 Int
类型转换是一种将值从一种数据类型转换为另一种数据类型的方法。Float 数据类型比 Int 数据类型占用更多的字节。因此,我们必须使用显式类型转换将 float 值转换为 Int 值。以下代码示例向我们展示了如何使用 C# 中的显式类型转换将浮点数转换为整数值。
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.2f;
int i = (int)f;
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
输出:
Converted float 10.2 to int 10
我们使用 C# 中的显式类型转换将 float 变量 f
转换为整数变量 i
。(int)
用于将 f
转换为 i
。这种方法的问题在于它会忽略小数点后的所有值。例如,浮点数 10.9
也将转换为整数值 10
。
在 C# 中使用 Math.Ceiling()
函数将 Float 转换为 Int
如果要考虑小数点后的值,则必须使用除显式类型转换之外的其他方法。Math.Ceiling()
函数用于将十进制值转换为下一个整数值。Math.Ceiling()
函数返回一个双精度值,可以使用显式类型转换将其转换为整数值。以下代码示例向我们展示了如何使用 C# 中的 Math.Ceiling()
函数将浮点数转换为整数值。
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.2f;
int i = (int)Math.Ceiling(f);
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
输出:
Converted float 10.8 to int 11
我们使用 C# 中的 Math.Ceiling()
函数将浮点变量 f
转换为整数变量 i
。(int)
用于将 Math.Ceiling()
函数返回的双精度值转换为整数值。这种方法的问题在于,它总是返回下一个整数值。例如,浮点数 10.1
也将转换为整数值 11
。
在 C# 中使用 Math.Floor()
函数将 Float 转换为 Int
Math.Floor()
函数也可以与显式类型转换一起使用,以将浮点数转换为 C# 中的整数值。Math.Floor()
函数用于将十进制值转换为前一个整数值。Math.Floor()
函数返回一个双精度值,可以通过显式类型转换将其转换为整数值。以下代码示例向我们展示了如何使用 C# 中的 Math.Floor()
函数将浮点数转换为整数值。
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.2f;
int i = (int)Math.Floor(f);
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
输出:
Converted float 10.2 to int 10
我们使用 C# 中的 Math.Floor()
函数将浮点变量 f
转换为整数变量 i
。(int)
用于将 Math.Floor()
函数返回的双精度值转换为整数值。这种方法的问题在于,它总是返回前一个整数值。例如,浮点数 10.9
也将转换为整数值 10
。
在 C# 中使用 Math.Round()
函数将 Float 转换为 Int
上面讨论的方法确实有效,但是每种方法都有一些缺点。如果要将浮点数转换为整数值,但又不想更改数据,可以在 C# 中使用 Math.Round()
函数。顾名思义,Math.Round()
函数用于将十进制值舍入到最接近的整数值。Math.Round()
返回一个双精度值,可以通过显式类型转换将其转换为整数数据类型。以下代码示例向我们展示了如何使用 C# 中的 Math.Round()
函数将浮点数转换为整数值。
using System;
namespace convert_float_to_int {
class Program {
static void Main(string[] args) {
float f = 10.8f;
int i = (int)Math.Round(f);
Console.WriteLine("Converted float {0} to int {1}", f, i);
}
}
}
输出:
Converted float 10.8 to int 11
我们使用 C# 中的 Math.Round()
函数将浮点变量 f
转换为整数变量 i
。(int)
用于将 Math.Round()
函数返回的双精度值转换为整数值。这是在 C# 中将浮点数转换为整数值的最佳方法。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn