在 C# 中将布尔值转换为整数
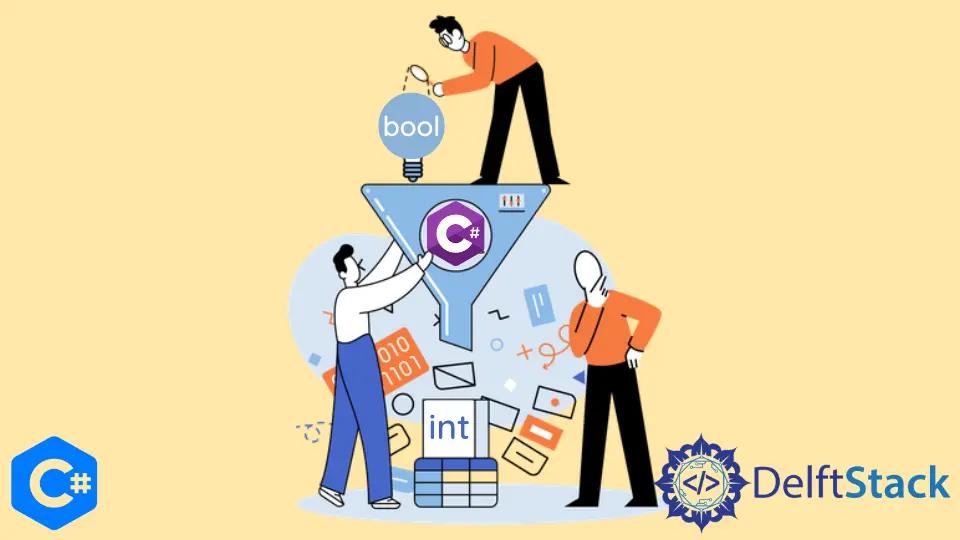
本文将介绍在 C# 中将布尔数据类型转换为整数。
在 C# 中使用 ConvertToInt32
语句将布尔值转换为整数
传统上,没有将数据类型从布尔值隐式转换为整数。但是,Convert.ToInt32()
方法将指定值转换为 32 位有符号整数。
值得一提的是,Convert.ToInt32()
方法类似于 int.Parse()
方法,但 int.Parse()
方法只接受字符串数据类型作为参数并抛出错误当一个 null 作为参数传递时。
相比之下,Convert.ToInt32()
方法不受这些限制的影响。
进一步的讨论可通过本参考文献获得。
下面是使用 Convert.ToInt32()
方法的代码示例。
// C# program to illustrate the
// use of Convert.ToInt32 statement
// and Convert.ToBoolean
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = Convert.ToInt32(boolinput);
bool boolRresult = Convert.ToBoolean(intRresult);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
输出:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
下面是使用 int.Parse()
方法的代码示例。
// C# program to illustrate the
// use of int.Parse statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = int.Parse(boolinput);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
}
}
输出:
program.cs(12,30): error CS1503: Argument 1: cannot convert from 'bool' to 'string'
使用 int.Parse
方法将 Boolean
数据类型转换为 integer
会显示上述错误。可以看出,该方法需要一个字符串
作为参数,而不是一个布尔
数据类型。
在 C# 中使用 switch
语句将布尔值转换为整数
switch
语句用于在程序执行期间有条件地分支。它决定要执行的代码块。将表达式的值与每个案例的值进行比较,
如果匹配,则执行相关的代码块。switch
表达式只计算一次。
下面是使用 switch
语句的代码示例。
// C# program to illustrate the
// use of switch statement
using System;
namespace Test {
class Program {
static void Main(string[] args) {
int i = 1;
bool b = true;
switch (i) {
case 0:
b = false;
Console.WriteLine("When integer is 0, boolean is:");
Console.WriteLine(b);
break;
case 1:
b = true;
Console.WriteLine("When integer is 1, boolean is:");
Console.WriteLine(b);
break;
}
switch (b) {
case true:
i = 1;
Console.WriteLine("When boolean is true, integer is:");
Console.WriteLine(i);
break;
case false:
i = 0;
Console.WriteLine("When boolean is false, integer is:");
Console.WriteLine(i);
break;
}
}
}
}
输出:
When an integer is 1, boolean is:
True
When boolean is true, integer is:
1
在 C# 中使用三元条件运算符将布尔值转换为整数
条件运算符 ?:
,也称为三元条件运算符,类似于 if
语句。它计算一个布尔表达式并返回两个表达式之一的结果。
如果布尔表达式为真,则返回第一条语句(即 ?
之后的语句);返回第二个语句(即 :
之后的语句)。进一步的讨论可通过本参考文献获得。
下面是一个代码示例。
// C# program to illustrate the
// use of ternary operator
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = boolinput ? 1 : 0;
bool boolRresult = (intRresult == 1) ? true : false;
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
输出:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
在 C# 中使用 if
语句将布尔值转换为整数
if
语句在执行某些逻辑表达式后检查特定条件是真还是假。每当表达式返回 true
时,都会返回数值 1,即 0。
类似地,当数值为 1 时,返回布尔值 true
。否则,返回 false
。
下面是一个代码示例。
// C# program to illustrate the
// use of if statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intResult;
if (boolinput) {
intResult = 1;
} else {
intResult = 0;
}
bool boolResult;
if (intResult == 1) {
boolResult = true;
} else {
boolResult = false;
}
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intResult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolResult);
}
}
输出:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe