在 C# 中将 Int 转换为字节
-
在
C#
中使用ToByte(String)
方法将Int
转换为Byte[]
-
在
C#
中使用ToByte(UInt16)
方法将Int
转换为Byte[]
-
在
C#
中使用ToByte(String, Int32)
方法将Int
转换为Byte[]
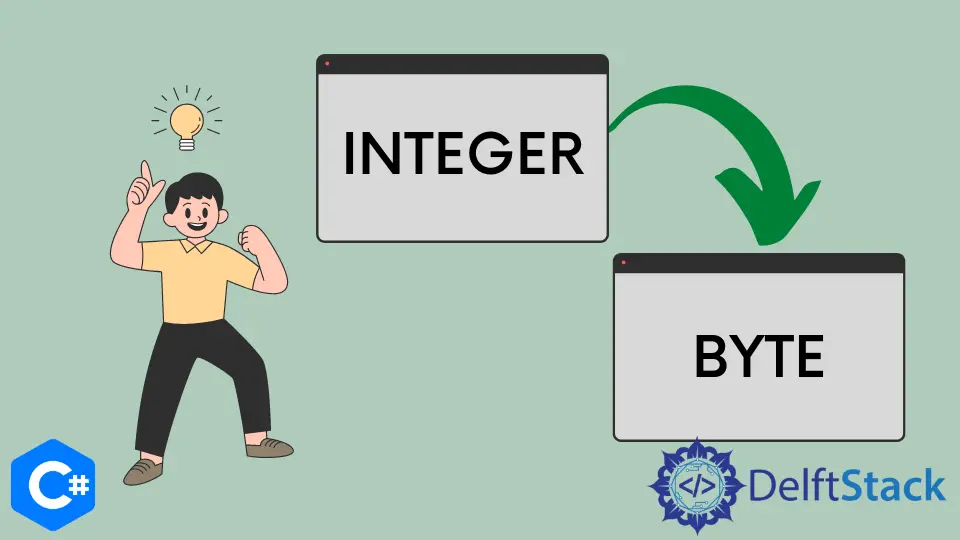
我们将研究在 C# 中将 Int
转换为 Byte[]
的几种不同技术。
在 C#
中使用 ToByte(String)
方法将 Int
转换为 Byte[]
这种方法通过使用 ToByte(String)
方法将提供的数字字符串表示形式转换为等效的 8 位无符号整数来工作。它作为一个字符串参数,包含要转换的数字。
下面的示例创建一个字符串数组并将每个字符串转换为一个字节。
首先,添加这些库。
using System;
using System.Diagnostics;
我们首先创建一个名为 Alldatavalues
的 string[]
类型变量并分配一些值。
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
现在使用 Convert.ToByte()
使用 for each
循环将整数转换为字节。
byte num = Convert.ToByte(value);
当值没有可选符号后跟一系列数字时,会发生格式异常(0 到 9)。我们利用 FormatException
处理程序来克服这个问题。
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
当值反映小于 MinValue
或大于 MaxValue
时,会出现溢出错误。
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
源代码:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' --> {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
输出:
'<null>' --> 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' --> 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
在 C#
中使用 ToByte(UInt16)
方法将 Int
转换为 Byte[]
ToByte(UInt16)
方法将 16 位无符号整数的值转换为等效的 8 位无符号整数。要进行转换,它需要一个 16 位无符号整数作为参数。
在以下示例中,无符号 16 位整数数组被转换为字节值。
要添加的库有:
using System;
using System.Diagnostics;
首先,初始化一个名为 data
的 ushort[]
类型变量;之后,我们将为其分配一些值,例如 UInt16.MinValue
作为最小值,UInt16.MaxValue
作为最大值以及我们想要转换的其他值,就像我们插入 90
和 880
.
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
然后我们将创建一个名为 result
的 byte
类型变量。
byte result;
之后,应用一个 for each
循环,它将转换为 Byte
类型的数据;除此之外,如果一个数字超出 Byte
的范围,它将显示异常。
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
源代码:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
输出:
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
在 C#
中使用 ToByte(String, Int32)
方法将 Int
转换为 Byte[]
此方法将数字的字符串表示形式转换为给定基数的等效 8 位无符号整数。它需要一个包含要转换的数字的 string
参数值。
将添加以下库。
using System;
using System.Diagnostics;
首先,我们将构造一个名为 Allbasedata
的 int[]
类型变量并分配基数 8
和 10
。
int[] Allbasedata = { 8, 10 };
现在我们创建一个名为 values
的 string[]
类型变量并为其赋值,并声明一个名为 number
的 byte
类型变量。
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
然后我们将应用一个 foreach
循环,它将数字转换为 byte
格式,它还将处理以下异常:
FormatException
:当值包含的字符不是来自 base 提供的 base 中的有效数字时,会发生此错误。OverflowException
:当负号前缀到表示以 10 为基数的无符号数的值时,会发生此错误。ArgumentException
:当值为空时发生此错误。
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
源代码:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
输出:
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn