Convertir Int a Byte en C#
-
Utilice el método
ToByte(String)
para convertirInt
aByte[]
enC#
-
Utilice el método
ToByte(UInt16)
para convertirInt
aByte[]
enC#
-
Utilice el método
ToByte(String, Int32)
para convertirInt
aByte[]
enC#
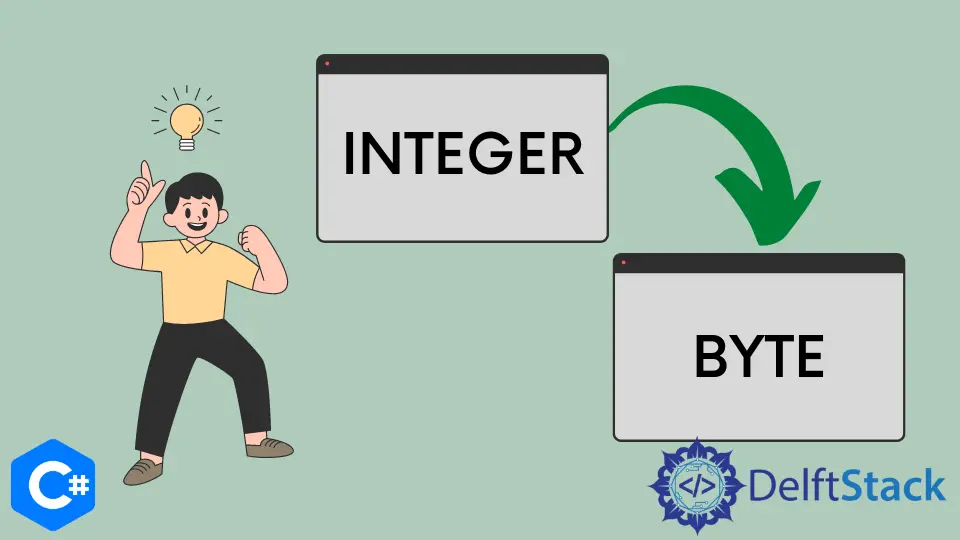
Veremos algunas técnicas diferentes para convertir Int
a Byte[]
en C#.
Utilice el método ToByte(String)
para convertir Int
a Byte[]
en C#
Este enfoque funciona convirtiendo la representación de cadena proporcionada de un número en un entero sin signo equivalente de 8 bits mediante el método ToByte(String)
. Se toma como argumento de cadena, que contiene el número a convertir.
El siguiente ejemplo crea un array de cadenas y convierte cada cadena en un byte.
Primero, agregue estas bibliotecas.
using System;
using System.Diagnostics;
Comenzamos creando una variable de tipo string[]
llamada Alldatavalues
y asignando algunos valores.
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
Ahora use un bucle for each
para convertir enteros a bytes usando Convert.ToByte()
.
byte num = Convert.ToByte(value);
Se produce una excepción de formato (0 a 9) cuando un valor no tiene un signo opcional seguido de una serie de números. Utilizamos el controlador FormatException
para superar esto.
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
Un error de desbordamiento surge cuando un valor refleja menor que MinValue
o mayor que MaxValue
.
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
Código fuente:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' --> {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
Producción :
'<null>' --> 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' --> 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
Utilice el método ToByte(UInt16)
para convertir Int
a Byte[]
en C#
El método ToByte(UInt16)
convierte el valor de un entero sin signo de 16 bits en un entero equivalente de 8 bits sin signo. Para convertir, requiere un entero sin signo de 16 bits como argumento.
En el siguiente ejemplo, un array de enteros de 16 bits sin signo se convierte en valores de byte.
Las bibliotecas que se agregarán son:
using System;
using System.Diagnostics;
Primero, inicialice una variable de tipo ushort[]
llamada data
; después de eso, le asignaremos algún valor como UInt16.MinValue
como valor mínimo, UInt16.MaxValue
como valor máximo y otros valores que queremos convertir como hemos insertado 90
y 880
.
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
Luego crearemos una variable de tipo byte
llamada resultado
.
byte result;
Después de eso, aplique un bucle foreach
que convertirá los datos en tipo Byte
; aparte de eso, si un número excede el rango de Byte
, mostrará una excepción.
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
Código fuente:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
Producción :
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
Utilice el método ToByte(String, Int32)
para convertir Int
a Byte[]
en C#
Este método convierte la representación de cadena de un número en un entero sin signo equivalente de 8 bits en una base dada. Toma un valor de parámetro string
que contiene el número a convertir.
Se agregarán las siguientes bibliotecas.
using System;
using System.Diagnostics;
Primero, construiremos una variable de tipo int[]
llamada Allbasedata
y le asignaremos las bases 8
y 10
.
int[] Allbasedata = { 8, 10 };
Ahora crearemos una variable de tipo string[]
llamada values
y le asignaremos valores, y declararemos una variable de tipo byte
llamada number
.
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
Luego aplicaremos un bucle foreach
en el que convierte el número a formato byte
, y también manejará excepciones como:
FormatException
: este error ocurre cuando el valor contiene un carácter que no es un dígito válido en la base proporcionada por from base.OverflowException
: este error se produce cuando se antepone un signo negativo a un valor que representa un número sin signo en base 10.ArgumentException
: Este error ocurre cuando el valor está vacío.
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
Código fuente:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
Producción :
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn