Convertir Int en Byte en C#
-
Utilisez la méthode
ToByte(String)
pour convertirInt
enByte[]
enC#
-
Utilisez la méthode
ToByte(UInt16)
pour convertirInt
enByte[]
enC#
-
Utilisez la méthode
ToByte(String, Int32)
pour convertirInt
enByte[]
enC#
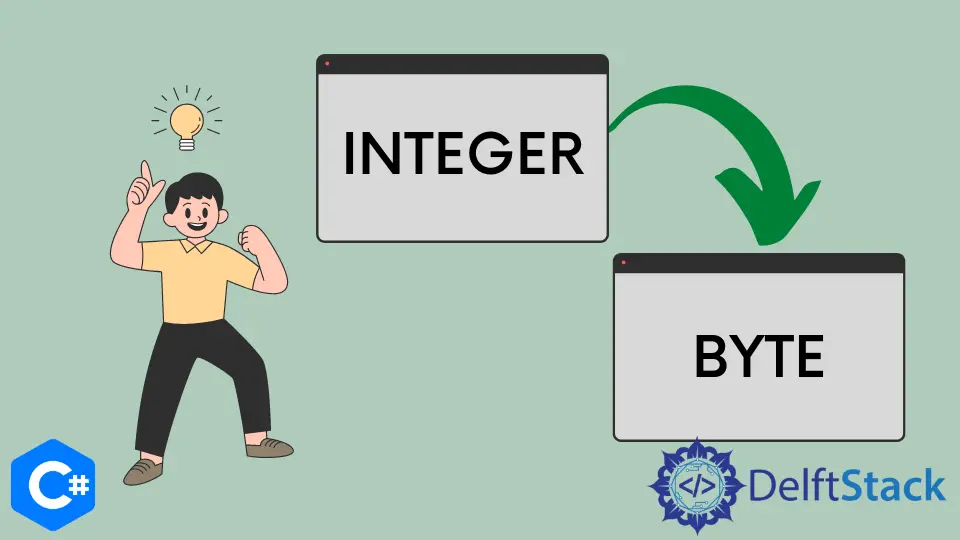
Nous allons examiner quelques techniques différentes pour convertir Int
en Byte[]
en C#.
Utilisez la méthode ToByte(String)
pour convertir Int
en Byte[]
en C#
Cette approche fonctionne en convertissant la représentation sous forme de chaîne fournie d’un nombre en un entier non signé 8 bits équivalent à l’aide de la méthode ToByte(String)
. Il prend comme argument de chaîne, contenant le nombre à convertir.
L’exemple ci-dessous crée un tableau de chaînes et convertit chaque chaîne en un octet.
Tout d’abord, ajoutez ces bibliothèques.
using System;
using System.Diagnostics;
Nous commençons par créer une variable de type string[]
appelée Alldatavalues
et lui attribuons des valeurs.
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
Utilisez maintenant une boucle for each
pour convertir un entier en octet en utilisant Convert.ToByte()
.
byte num = Convert.ToByte(value);
Une exception de format se produit (0 à 9) lorsqu’une valeur n’a pas de signe facultatif suivi d’une série de nombres. Nous utilisons le gestionnaire FormatException
pour surmonter cela.
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
Une erreur de débordement survient lorsqu’une valeur reflète une valeur inférieure à MinValue
ou supérieure à MaxValue
.
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
Code source:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' --> {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
Production:
'<null>' --> 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' --> 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
Utilisez la méthode ToByte(UInt16)
pour convertir Int
en Byte[]
en C#
La méthode ToByte(UInt16)
convertit la valeur d’un entier non signé 16 bits en un équivalent entier non signé 8 bits. Pour convertir, il nécessite un entier non signé 16 bits comme argument.
Dans l’exemple suivant, un tableau d’entiers 16 bits non signés est converti en valeurs Byte.
Les bibliothèques à ajouter sont :
using System;
using System.Diagnostics;
Tout d’abord, initialisez une variable de type ushort[]
nommée data
; après cela, nous lui attribuerons une valeur comme UInt16.MinValue
comme valeur minimale, UInt16.MaxValue
comme valeur maximale et d’autres valeurs que nous voulons convertir comme nous avons inséré 90
et 880
.
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
Ensuite, nous allons créer une variable de type byte
nommée resultat
.
byte result;
Après cela, appliquez une boucle pour chaque
qui convertira les données en type Byte
; à part cela, si un nombre dépasse la plage de Byte
, il affichera une exception.
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
Code source:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
Production:
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
Utilisez la méthode ToByte(String, Int32)
pour convertir Int
en Byte[]
en C#
Cette méthode convertit la représentation sous forme de chaîne d’un nombre en un entier non signé 8 bits équivalent dans une base donnée. Il prend une valeur de paramètre chaîne
contenant le nombre à convertir.
Les bibliothèques suivantes seront ajoutées.
using System;
using System.Diagnostics;
Dans un premier temps, nous allons construire une variable de type int[]
appelée Allbasedata
et lui affecter les bases 8
et 10
.
int[] Allbasedata = { 8, 10 };
Maintenant, nous créons une variable de type chaîne[]
appelée valeurs
et lui attribuons des valeurs, et déclarons une variable de type octet
appelée name
.
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
Ensuite, nous appliquerons une boucle foreach
dans laquelle elle convertit le nombre au format byte
, et elle gérera également les exceptions telles que :
FormatException
: cette erreur se produit lorsque la valeur contient un caractère qui n’est pas un chiffre valide dans la base fournie par de base.OverflowException
: cette erreur se produit lorsqu’un signe négatif est préfixé à une valeur représentant un nombre non signé en base 10.ArgumentException
: cette erreur se produit lorsque la valeur est vide.
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
Code source:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
Production:
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn