C#에서 Int를 바이트로 변환
-
ToByte(String)
메서드를 사용하여C#
에서Int
를Byte[]
로 변환 -
ToByte(UInt16)
메서드를 사용하여C#
에서Int
를Byte[]
로 변환 -
ToByte(String, Int32)
메서드를 사용하여C#
에서Int
를Byte[]
로 변환
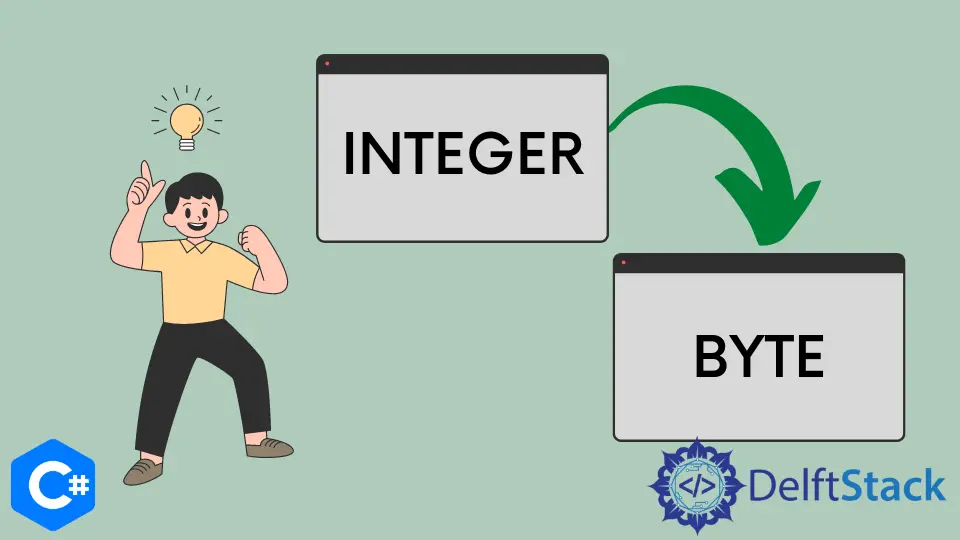
C#에서 Int
를 Byte[]
로 변환하는 몇 가지 다른 기술을 살펴보겠습니다.
ToByte(String)
메서드를 사용하여 C#
에서 Int
를 Byte[]
로 변환
이 접근 방식은 ToByte(String)
메서드를 사용하여 숫자의 제공된 문자열 표현을 동등한 8비트 부호 없는 정수로 변환하여 작동합니다. 변환할 숫자를 포함하는 문자열 인수로 사용합니다.
아래 예제에서는 문자열 배열을 만들고 각 문자열을 바이트로 변환합니다.
먼저 이러한 라이브러리를 추가합니다.
using System;
using System.Diagnostics;
먼저 Alldatavalues
라는 string[]
유형 변수를 만들고 일부 값을 할당합니다.
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
이제 for each
루프를 사용하여 Convert.ToByte()
를 사용하여 정수를 바이트로 변환합니다.
byte num = Convert.ToByte(value);
값에 일련의 숫자가 오는 선택적 기호가 없으면 형식 예외가 발생합니다(0 - 9). 이를 극복하기 위해 FormatException
핸들러를 사용합니다.
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
값이 MinValue
보다 작거나 MaxValue
보다 큰 경우 오버플로 오류가 발생합니다.
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
소스 코드:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' --> {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
출력:
'<null>' --> 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' --> 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
ToByte(UInt16)
메서드를 사용하여 C#
에서 Int
를 Byte[]
로 변환
‘ToByte(UInt16)’ 메서드는 16비트 부호 없는 정수 값을 8비트 부호 없는 정수로 변환합니다. 변환하려면 인수로 16비트 부호 없는 정수가 필요합니다.
다음 예에서는 부호 없는 16비트 정수 배열이 바이트 값으로 변환됩니다.
추가할 라이브러리는 다음과 같습니다.
using System;
using System.Diagnostics;
먼저 data
라는 ushort[]
유형 변수를 초기화합니다. 그 후 UInt16.MinValue
를 최소값으로, UInt16.MaxValue
를 최대값으로, 그리고 90
과 880
을 삽입한 것처럼 변환하려는 다른 값을 할당합니다. .
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
그런 다음 result
라는 byte
유형 변수를 생성합니다.
byte result;
그런 다음 Byte
유형의 데이터를 변환하는 for each
루프를 적용합니다. 그 외에 숫자가 Byte
의 범위를 초과하면 예외가 표시됩니다.
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
소스 코드:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
출력:
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
ToByte(String, Int32)
메서드를 사용하여 C#
에서 Int
를 Byte[]
로 변환
이 메서드는 숫자의 문자열 표현을 주어진 기수에서 해당하는 8비트 부호 없는 정수로 변환합니다. 변환할 숫자를 포함하는 string
매개변수 값을 취합니다.
다음 라이브러리가 추가됩니다.
using System;
using System.Diagnostics;
먼저 Allbasedata
라는 int[]
유형 변수를 구성하고 기본 8
과 10
을 할당합니다.
int[] Allbasedata = { 8, 10 };
이제 values
라는 string[]
유형 변수를 만들고 여기에 값을 할당하고 number
라는 byte
유형 변수를 선언합니다.
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
그런 다음 숫자를 byte
형식으로 변환하는 foreach
루프를 적용하고 다음과 같은 예외도 처리합니다.
FormatException
: 이 오류는 base에서 제공한 base의 유효한 숫자가 아닌 문자가 값에 포함된 경우 발생합니다.OverflowException
: 이 오류는 음수 부호가 밑수 10의 부호 없는 숫자를 나타내는 값 앞에 접두어일 때 발생합니다.ArgumentException
: 이 오류는 값이 비어 있을 때 발생합니다.
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
소스 코드:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
출력:
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn