C#에서 Char를 Int로 변환
-
C#
의char
데이터 유형 -
Char.GetNumericValue()
메서드를 사용하여C#
에서char
를int
로 변환 -
Convert.ToInt32()
메서드를 사용하여C#
에서char
를int
로 변환 -
Int32.Parse()
메서드를 사용하여C#
에서char
를int
로 변환
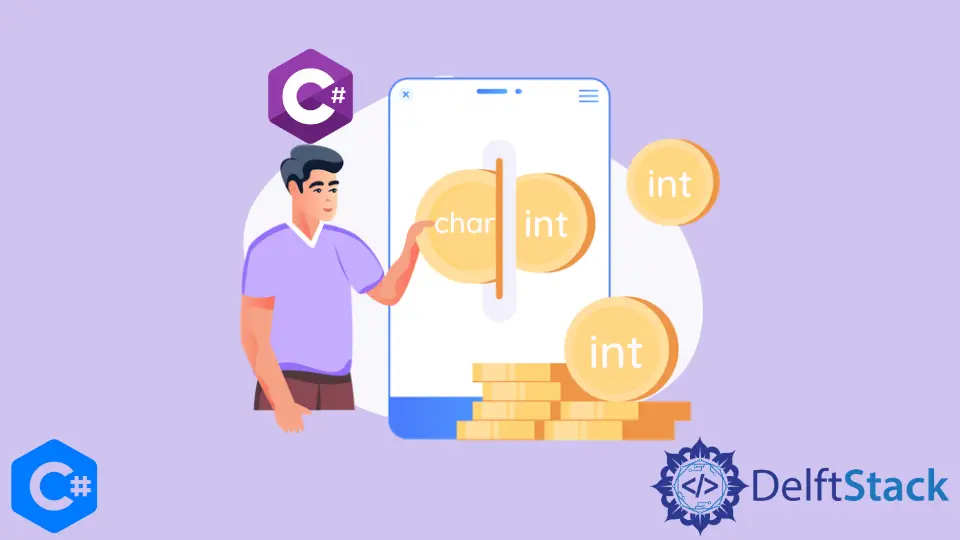
C#은 객체 지향 프로그래밍 언어입니다. 이는 변수가 저장할 값의 유형을 나타내면서 모든 변수를 선언해야 함을 의미합니다.
C# 변수는 데이터 유형
이라는 다양한 형식 또는 유형으로 저장됩니다.
C#
의 char
데이터 유형
Char
데이터 유형으로 변수를 선언하는 샘플 구문.
type variableName = value;
char grade = 'A';
char myCharacter = 'X';
char myLuckyNumber = '3';
단일 문자는 char
데이터 유형에 저장됩니다. 문자는 'A'
또는 'X'
와 같이 작은따옴표로 묶어야 합니다.
Char.GetNumericValue()
메서드를 사용하여 C#
에서 char
를 int
로 변환
Char.GetNumericValue()
메서드는 지정된 숫자 유니코드 문자를 배정밀도 부동 소수점 숫자로 변환합니다.
작은따옴표로 묶인 숫자 값이 있는 char
변수에 메서드를 적용하면 해당 숫자가 반환되고, 그렇지 않으면 -1이 반환됩니다.
코드 조각:
using System;
public class GetNumericValue {
public static void Main() {
int result = (int)Char.GetNumericValue('8');
Console.WriteLine(result); // Output: "8"
Console.WriteLine(Char.GetNumericValue('X')); // Output: "-1"
}
}
출력:
8-1
Convert.ToInt32()
메서드를 사용하여 C#
에서 char
를 int
로 변환
Convert.ToInt32()
함수는 지정된 값을 32비트 부호 있는 정수로 변환합니다. ToInt32()
메서드에는 전달된 인수의 데이터 유형에 따라 많은 변형이 있습니다.
코드 조각:
using System;
public class ConvertCharToInt {
public static void Main() {
char c = '3';
Console.WriteLine(Convert.ToInt32(c.ToString())); // Output: "3"
Console.WriteLine(Convert.ToInt32(c)); // Output: "51"
}
}
출력:
351
char
데이터 유형이 Convert.Tolnt32()
메서드에 인수로 전달되면 ASCII
에 해당하는 값이 반환됩니다.
Int32.Parse()
메서드를 사용하여 C#
에서 char
를 int
로 변환
Int32.Parse()
메서드는 숫자의 문자열
표현을 해당하는 32비트 부호 있는 정수로 변환합니다. 이 방법의 단점은 숫자 값을 문자열
인수로 전달해야 한다는 것입니다.
char
데이터 유형은 먼저 string
데이터 유형으로 변환되어야 하며 작은 따옴표 ' '
로 묶인 숫자 값을 포함해야 합니다. 그렇지 않으면 코드에서 오버플로 예외
가 발생합니다.
코드 조각:
using System;
public class GetNumericValue {
public static void Main() {
char c = '3';
char s = 'x';
string str = c.ToString();
string ex = s.ToString();
Console.WriteLine(Int32.Parse(str));
// Console.WriteLine(Int32.Parse(ex)); // Output: "ThrowEx"
}
}
출력:
3
char
값이 아래와 같이 작은따옴표 ' '
로 묶인 숫자가 아닌 경우 예외가 발생합니다.
코드 조각:
using System;
public class GetNumericValue {
public static void Main() {
char s = 'x';
string ex = s.ToString();
Console.WriteLine(Int32.Parse(ex));
}
}
출력:
Unhandled Exception:
System.FormatException: Input string was not in a correct format.
관련 문장 - Csharp Char
- C# char를 int로 변환
- C#에서 문자열 내부의 문자 발생 횟수 계산
- C#에서 문자열의 첫 문자 가져 오기
- C#에서 문자의 ASCII 값 가져 오기
- C#의 문자열에서 문자 제거