How to Convert Char to Int in C#
-
the
char
Data Type inC#
-
Use the
Char.GetNumericValue()
Method to Convertchar
toint
inC#
-
Use the
Convert.ToInt32()
Method to Convertchar
toint
inC#
-
Use the
Int32.Parse()
Method to Convertchar
toint
inC#
- Conclusion
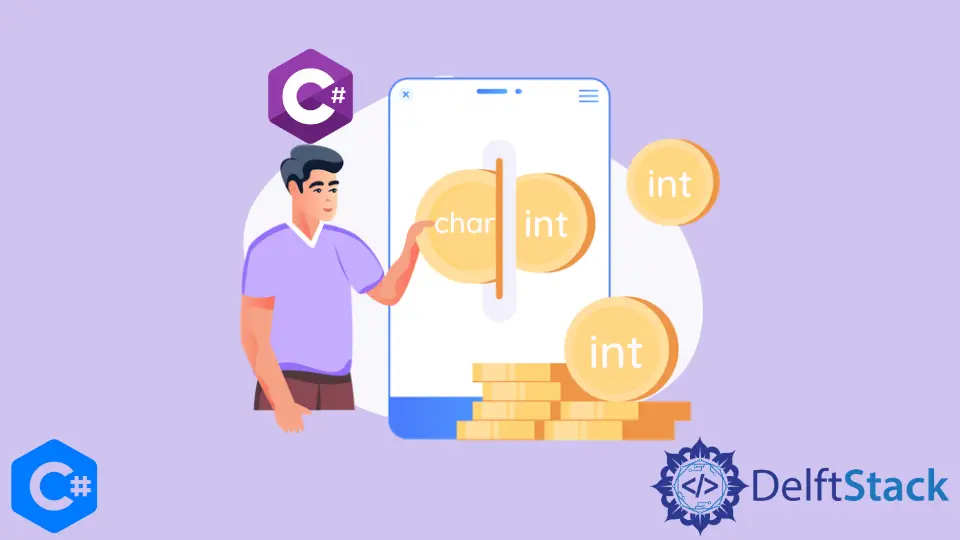
C# is an object-oriented programming language. This implies that every variable must be declared while indicating the type of values that variable will store.
C# variables are stored in different forms or types called Data types
.
the char
Data Type in C#
Sample syntax of declaring a variable with Char
data type.
type variableName = value;
char grade = 'A';
char myCharacter = 'X';
char myLuckyNumber = '3';
A single character is stored in the char
data type. The characters must be enclosed in single quotes, such as 'A'
or 'X'
.
Use the Char.GetNumericValue()
Method to Convert char
to int
in C#
The Char.GetNumericValue()
method converts a specified numeric Unicode character to a double-precision floating-point number.
Syntax:
double numericValue = Char.GetNumericValue(char c);
double
: This is the data type of the value returned by the method. It indicates that the method returns a double-precision floating-point number.numericValue
: This is the variable that will store the numeric value obtained from the character.Char
: This is the class name where theGetNumericValue
method is defined..GetNumericValue()
: This is the method being called on theChar
class.char c
: This is the parameter you pass to the method, representing the character for which you want to get the numeric value.
If the method is applied on a char
variable with a numeric value enclosed in single quotes, that number is returned; else, -1
is returned.
Code Snippet:
using System;
public class GetNumericValue {
public static void Main() {
int result = (int)Char.GetNumericValue('8');
Console.WriteLine(result); // Output: "8"
Console.WriteLine(Char.GetNumericValue('X')); // Output: "-1"
}
}
First, we get the numeric value of the character '8'
and cast it to an integer (int result = (int)Char.GetNumericValue('8');
). We then print the result (8
) to the console.
Next, we get the numeric value of the character 'X'
. Since 'X'
is not a valid numeric character, it returns -1
.
The result (-1
) is then printed to the console.
Output:
8-1
Use the Convert.ToInt32()
Method to Convert char
to int
in C#
The Convert.ToInt32()
function converts a specified value to a 32-bit signed integer. The ToInt32()
method has many variants depending on the data type of the argument passed.
Syntax:
int integerValue = Convert.ToInt32(object value);
int
: This is the data type of the variableintegerValue
, which will store the result of the conversion as a 32-bit integer (int
).integerValue
: This is the variable that will store the numeric value obtained from the character.Convert.ToInt32()
: This is the method you call to perform the conversion.object value
: This is the parameter of the method, representing the value you want to convert to an integer. The parameter accepts an object, allowing you to pass values of different types that can be converted to integers, such as strings and numbers.
Here’s an example of using the Convert.ToInt32()
Method:
Code Snippet:
using System;
public class ConvertCharToInt {
public static void Main() {
char c = '3';
Console.WriteLine(Convert.ToInt32(c.ToString())); // Output: "3"
Console.WriteLine(Convert.ToInt32(c)); // Output: "51"
}
}
In the code example, we declare a character variable 'c'
and assign the value '3'
to it (char c = '3';
).
We convert the character 'c'
to a string using c.ToString()
and convert the resulting string to an integer. The character '3'
is then converted to the string "3"
and then to the integer 3
.
Lastly, we directly convert the character 'c'
to an integer. The character '3'
has an ASCII value of 51
, so it is converted to the integer 51
.
Output:
351
If the char
data type is passed as an argument into the Convert.Tolnt32()
method, the ASCII
equivalent is returned.
Use the Int32.Parse()
Method to Convert char
to int
in C#
The Int32.Parse()
method converts the string
representation of a number to its 32-bit signed integer equivalent. The downside of this method is that a numeric value must be passed as the string
argument.
The char
data type must first be converted to string
data type and must contain a numeric value in single quotes ' '
; else, the code will throw an overflow exception
.
Syntax:
Int32.Parse(string s);
Int32.Parse()
: This is the method you call to perform the conversion.string s
: This is the parameter of the method, representing the string you want to parse and convert to an integer. The parameter should contain a valid integer representation as a string.
Code Snippet:
using System;
public class GetNumericValue {
public static void Main() {
char c = '3';
char s = 'x';
string str = c.ToString();
string ex = s.ToString();
Console.WriteLine(Int32.Parse(str));
}
}
In the code above, we declare a character variable 'c'
and assign the value '3'
(char c = '3';
). We then declare another character variable 's'
and assign the value 'x'
(char s = 'x';
).
Next, we convert the characters 'c'
and 's'
to a string using the ToString()
method and store it in 'str'
and 'ex'
respectively.
Lastly, we parse the string 'str'
as an integer using Int32.Parse()
and print the result. The string "3"
is successfully parsed and converted to the integer 3
.
Output:
3
The output is "3"
because the Int32.Parse()
method successfully converts the string "3"
into the integer 3
.
An exception is thrown if the char
value is not numeric in single quotes ' '
as shown below.
Code Snippet:
using System;
public class GetNumericValue {
public static void Main() {
char s = 'x';
string ex = s.ToString();
Console.WriteLine(Int32.Parse(ex));
}
}
In this code, we tried to parse the string 'ex'
as an integer using Int32.Parse()
. Since 'ex'
contains the string "x"
, which is not a valid integer representation, it will throw a System.FormatException
because the input string is not in the correct format.
Output:
Unhandled Exception:
System.FormatException: Input string was not in a correct format.
The output above is an unhandled exception message. This exception occurs because the Int32.Parse()
method expects a valid integer representation as input, and "x"
does not fit that format.
Conclusion
We discussed three methods for converting char
values to integers in C#:
-
Char.GetNumericValue()
, which returns adouble
or-1.0
for invalid characters. -
Convert.ToInt32()
, ideal for obtaining the ASCII equivalent as a 32-bit signed integer. -
Int32.Parse ()
, which converts valid numericchar
values after converting them to strings.
Your choice among these methods depends on your code’s requirements and the data format, but always ensure data adherence to the expected format to avoid errors.
Related Article - Csharp Char
- How to Convert a Char to an Int in C#
- How to Count Occurrences of a Character Inside a String in C#
- How to Get the First Character of a String in C#
- How to Get ASCII Value of Character in C#
- How to Remove a Character From a String in C#