How to Get ASCII Value of Character in C#
- Get the ASCII Value of Characters in a String Using Typecasting in C#
-
Get the ASCII Value of Characters in a String Using the
Encoding.ASCII.GetBytes
Method in C# - Conclusion
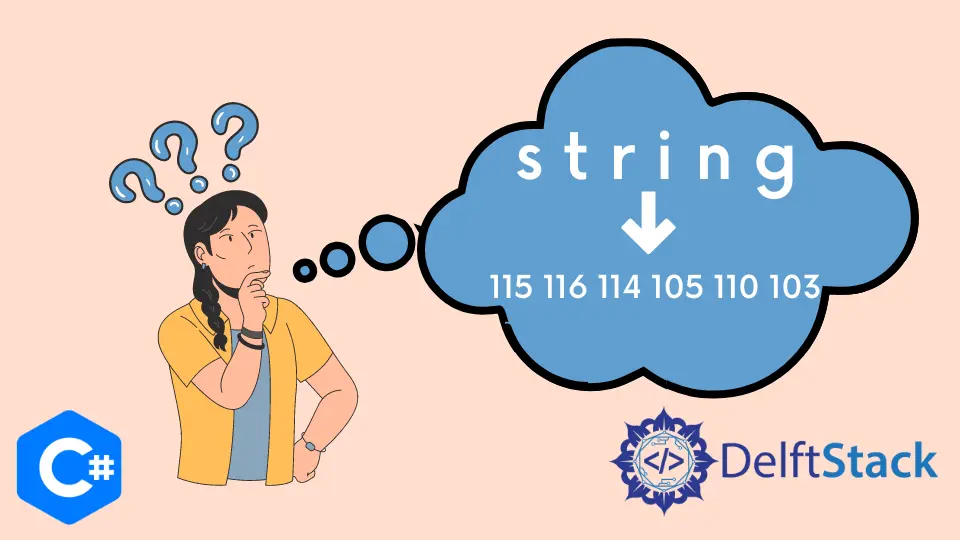
Understanding ASCII values and how to retrieve them in a programming language like C# is crucial for various applications involving character manipulation.
In this article, we will delve deeper into different methods of obtaining ASCII values for characters in a string using typecasting and the Encoding.ASCII.GetBytes
method.
Get the ASCII Value of Characters in a String Using Typecasting in C#
Typecasting involves converting a value from one data type to another. In the context of ASCII, we can use typecasting to convert characters (which are essentially numeric values representing characters) to their corresponding ASCII values.
The ASCII standard assigns unique numerical values to each character, facilitating various operations involving textual data.
Typecasting is achieved by using a casting operator. Specifically, we use the (int)
casting operator to convert a character (of type char
) to its corresponding ASCII value, which is represented as an integer (of type int
).
The syntax for typecasting is as follows:
(int)char
Here, (int)
is the casting operator, and char
is the character we want to convert.
Let’s take a look at the example code that demonstrates how to retrieve the ASCII values of characters in a string using typecasting in C#:
using System;
namespace ASCIIValueOfString
{
class Program
{
static void Main(string[] args)
{
string str = "ABCDEFGHI";
Console.WriteLine("ASCII values of characters in the string:");
foreach (var c in str)
{
// Typecast each character to int to obtain its ASCII value
Console.WriteLine((int)c);
}
}
}
}
The output of the provided code will be as follows:
65
66
67
68
69
70
71
72
73
In this code, we set up a string called str
with the value ABCDEFGHI
. Then, using Console.WriteLine
, we print the message ASCII values of characters in the string:
to the console.
Now comes the interesting part: we use a foreach
loop to go through each character (denoted by c
) in our string.
For every character c
, we perform a typecasting operation, converting it into an integer using (int)c
. This integer is essentially the ASCII value of that character.
We display these ASCII values one by one on the console using Console.WriteLine
.
Get the ASCII Value of Characters in a String Using the Encoding.ASCII.GetBytes
Method in C#
If we don’t want to typecast each string’s character into an int
, we can use a byte array instead. We can get the ASCII values of characters in a string by converting the string into an array of bytes using the Encoding.ASCII.GetBytes
method and then displaying each element of the resulting byte array.
The Encoding.ASCII.GetBytes
method is part of the System.Text.Encoding
class and provides a straightforward way to work with ASCII-encoded strings. This method has the following signature:
public virtual byte[] GetBytes(string s);
This method takes a string s
as an input and returns a byte array that represents the ASCII-encoded version of the input string.
Let’s explore how to use the Encoding.ASCII.GetBytes
method:
using System;
using System.Text;
namespace ASCII_value_of_a_string {
class Program {
static void Main(string[] args) {
string str = "ABCDEFGHI";
byte[] ASCIIvalues = Encoding.ASCII.GetBytes(str);
foreach (var value in ASCIIvalues) {
Console.WriteLine(value);
}
}
}
}
Output:
65
66
67
68
69
70
71
72
73
In this code snippet, we start by bringing in the necessary capabilities from the System.Text
namespace, which provides functionalities for text encoding and decoding. Within the Main
method, we initiate a string variable str
with the content "ABCDEFGHI"
.
Next, we utilize the Encoding.ASCII.GetBytes
method by passing our string str
as an input. This method converts the characters in the string to their corresponding ASCII values and stores them in a byte array called ASCIIvalues
.
Following that, we employ a foreach
loop to traverse through the ASCIIvalues
array, retrieving each ASCII value. For each value, we utilize Console.WriteLine
to display it on the console. Consequently, we obtain and showcase the ASCII values of the characters A to I.
Conclusion
This article delved into two key methods for obtaining ASCII values from characters within a string: typecasting and utilizing the Encoding.ASCII.GetBytes
method.
Typecasting, a process of converting a value from one data type to another, proved effective for converting characters into their corresponding ASCII values. By using the (int)
casting operator in C#, characters (of type char
) were seamlessly transformed into their ASCII representations (of type int
).
Additionally, the article explored an alternative approach using the Encoding.ASCII.GetBytes
method, which eliminated the need for individual typecasting. This method allowed us to convert an entire string into an array of bytes, each byte representing the ASCII value of a character.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn