How to Remove a Character From a String in C#
-
Remove Characters From a String With the
string.Replace()
Function inC#
-
Remove Characters From a String With the
string.Join()
and thestring.Split()
Functions inC#
-
Remove Characters From a String With the
Regex.Replace()
Function inC#
-
Remove Characters From a String With Linq in
C#
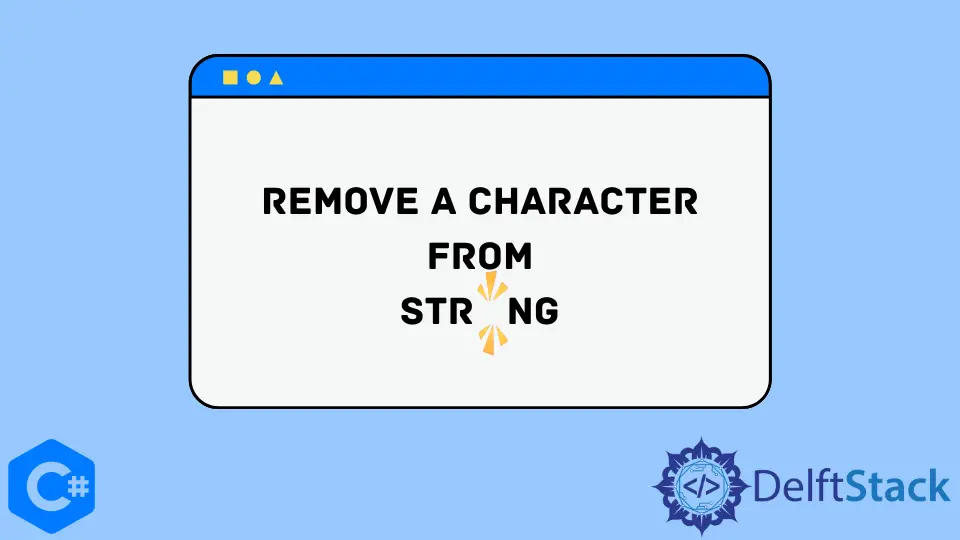
In this tutorial, we will discuss how to remove a character from a string in C#. You can refer to this article to learn how to remove whitespaces from a string in C#.
Remove Characters From a String With the string.Replace()
Function in C#
The string.Replace()
function can be used to replace a certain character inside a list. We can remove a character from a string by replacing the character with string.Empty
in C#. If we want to remove multiple characters from a string, we have to iterate through each character using a loop. The following code example shows us how we can remove multiple characters from a string with the string.Replace()
function in C#.
using System;
namespace remore_characters_from_string {
class Program {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
string[] charsToRemove = new string[] { "@", ",", ".", ";", "'" };
foreach (var c in charsToRemove) {
str = str.Replace(c, string.Empty);
}
Console.WriteLine(str);
}
}
}
Output:
Something to Write
We removed the characters { "@", ",", ".", ";", "'" }
from the string str
with the string.Replace()
function in C#. We created an array of all the characters that we want to remove from the str
string. To remove the characters, we iterated through each element of the array and replaced it with the string.Empty
by using the string.Replace()
function in C#.
Remove Characters From a String With the string.Join()
and the string.Split()
Functions in C#
The string.Join()
function is used to concatenate the elements of the string array passed in the parameters with the specified separator. The string.Split()
function is used to split a string into multiple string with the specified separator. The string.Join()
function can be used with the string.Split()
function to remove a character from a string. This approach is better than the string.Replace()
function because we do not have to use a loop to remove multiple characters. The following code example shows us how we remove multiple characters from a string with the string.Join()
and string.Split()
functions in C#.
using System;
namespace remore_characters_from_string {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
str = string.Join("", str.Split('@', ',', '.', ';', '\''));
Console.WriteLine(str);
}
}
}
Output:
Something to Write
We removed the characters { "@", ",", ".", ";", "'" }
from the string str
with the string.Join()
and the string.Split()
functions in C#. To remove the characters, we specified the characters in the parameters of string.Split()
function.
Remove Characters From a String With the Regex.Replace()
Function in C#
The Regex
, also known as regular expressions, are designed for robust text manipulation in C#. The Regex.Replace()
function is used to replace two texts in a specified order. We can remove characters from a string by replacing each character with a string.Empty
by specifying each character in the regex pattern parameter. The following code example shows us how we can remove multiple characters from a string with the Regex.Replace()
function in C#.
using System;
using System.Text.RegularExpressions;
namespace remore_characters_from_string {
class Program {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
str = Regex.Replace(str, "[@,\\.\";'\\\\]", string.Empty);
Console.WriteLine(str);
}
}
}
Output:
Something to Write
We removed the characters { "@", ",", ".", ";", "'" }
from the string str
with the Regex.Replace()
function in C#.
Remove Characters From a String With Linq in C#
The Language integrated query of Linq provides SQL-like functionality in C#. We can use Linq to remove characters from a string. The following code example shows us how we can remove multiple characters from a string with Linq in C#.
using System;
using System.Linq;
using System.Text.RegularExpressions;
namespace remore_characters_from_string {
class Program {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
str = new string((from c in str
where char.IsWhiteSpace(c) || char.IsLetterOrDigit(c)
select c
).ToArray());
Console.WriteLine(str);
}
}
}
Output:
Something to Write
We removed everything that is not a white-space or a letter or digit from the string str
with Linq in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Char
- How to Convert a Char to an Int in C#
- How to Convert Char to Int in C#
- How to Count Occurrences of a Character Inside a String in C#
- How to Get the First Character of a String in C#
- How to Get ASCII Value of Character in C#